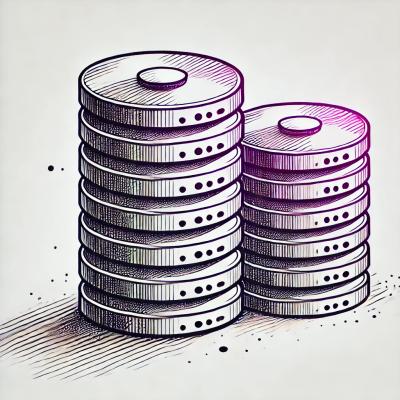
Security News
MCP Community Begins Work on Official MCP Metaregistry
The MCP community is launching an official registry to standardize AI tool discovery and let agents dynamically find and install MCP servers.
redux-mock-store
Advanced tools
A mock store for testing your redux async action creators and middleware
The redux-mock-store npm package is used for testing Redux async action creators and middleware. The package provides a mock store for your tests with a state that you can control. It allows you to dispatch actions and test the state of the store after they are dispatched without having to interact with the real Redux store.
Creating a mock store
This code sample demonstrates how to create a mock store using redux-mock-store. The `configureStore` function is imported from the package, and then it is called with an array of middlewares (empty in this case). Finally, the `mockStore` function is used to create an instance of the store with an initial state.
const configureStore = require('redux-mock-store');
const middlewares = [];
const mockStore = configureStore(middlewares);
const store = mockStore({ todos: [] });
Dispatching actions and checking dispatched actions
This code sample shows how to dispatch an action to the mock store and then retrieve the dispatched actions using `getActions` method. The `expect` function is used to assert that the dispatched actions are as expected.
store.dispatch({ type: 'ADD_TODO', text: 'Test Redux' });
const actions = store.getActions();
expect(actions).toEqual([{ type: 'ADD_TODO', text: 'Test Redux' }]);
Resetting the state of the store
This code sample illustrates how to reset the state of the mock store. The `clearActions` method is used to clear all actions in the store, and then `getActions` is used to verify that the store's actions have been reset.
store.clearActions();
const actions = store.getActions();
expect(actions).toEqual([]);
jest-redux is another testing utility that provides a set of Jest matchers for Redux. It is similar to redux-mock-store in that it helps with testing Redux-related code, but it is more focused on integrating with Jest and providing a different set of utilities for assertions.
The Redux team does not recommend testing using this library. Instead, see our docs for recommended practices, using a real store.
Testing with a mock store leads to potentially confusing behaviour, such as state not updating when actions are dispatched. Additionally, it's a lot less useful to assert on the actions dispatched rather than the observable state changes.
You can test the entire combination of action creators, reducers, and selectors in a single test, for example:
it('should add a todo', () => {
const store = makeStore() // a user defined reusable store factory
store.dispatch(addTodo('Use Redux'))
expect(selectTodos(store.getState())).toEqual([
{ text: 'Use Redux', completed: false }
])
})
This avoids common pitfalls of testing each of these in isolation, such as mocked state shape becoming out of sync with the actual application.
A mock store for testing Redux async action creators and middleware. The mock store will create an array of dispatched actions which serve as an action log for tests.
Please note that this library is designed to test the action-related logic, not the reducer-related one. In other words, it does not update the Redux store. If you want a complex test combining actions and reducers together, take a look at other libraries (e.g., redux-actions-assertions). Refer to issue #71 for more details.
npm install redux-mock-store --save-dev
Or
yarn add redux-mock-store --dev
The simplest usecase is for synchronous actions. In this example, we will test if the addTodo
action returns the right payload. redux-mock-store
saves all the dispatched actions inside the store instance. You can get all the actions by calling store.getActions()
. Finally, you can use any assertion library to test the payload.
import configureStore from 'redux-mock-store' //ES6 modules
const { configureStore } = require('redux-mock-store') //CommonJS
const middlewares = []
const mockStore = configureStore(middlewares)
// You would import the action from your codebase in a real scenario
const addTodo = () => ({ type: 'ADD_TODO' })
it('should dispatch action', () => {
// Initialize mockstore with empty state
const initialState = {}
const store = mockStore(initialState)
// Dispatch the action
store.dispatch(addTodo())
// Test if your store dispatched the expected actions
const actions = store.getActions()
const expectedPayload = { type: 'ADD_TODO' }
expect(actions).toEqual([expectedPayload])
})
A common usecase for an asynchronous action is a HTTP request to a server. In order to test those types of actions, you will need to call store.getActions()
at the end of the request.
import configureStore from 'redux-mock-store'
import thunk from 'redux-thunk'
const middlewares = [thunk] // add your middlewares like `redux-thunk`
const mockStore = configureStore(middlewares)
// You would import the action from your codebase in a real scenario
function success() {
return {
type: 'FETCH_DATA_SUCCESS'
}
}
function fetchData() {
return (dispatch) => {
return fetch('/users.json') // Some async action with promise
.then(() => dispatch(success()))
}
}
it('should execute fetch data', () => {
const store = mockStore({})
// Return the promise
return store.dispatch(fetchData()).then(() => {
const actions = store.getActions()
expect(actions[0]).toEqual(success())
})
})
configureStore(middlewares?: Array) => mockStore: Function
Configure mock store by applying the middlewares.
mockStore(getState?: Object,Function) => store: Function
Returns an instance of the configured mock store. If you want to reset your store after every test, you should call this function.
store.dispatch(action) => action
Dispatches an action through the mock store. The action will be stored in an array inside the instance and executed.
store.getState() => state: Object
Returns the state of the mock store.
store.getActions() => actions: Array
Returns the actions of the mock store.
store.clearActions()
Clears the stored actions.
store.subscribe(callback: Function) => unsubscribe: Function
Subscribe to the store.
store.replaceReducer(nextReducer: Function)
Follows the Redux API.
< 1.x.x
)https://github.com/arnaudbenard/redux-mock-store/blob/v0.0.6/README.md
The following versions are exposed by redux-mock-store from the package.json
:
main
: commonJS Versionmodule
/js:next
: ES Module Versionbrowser
: UMD versionThe MIT License
FAQs
A mock store for testing your redux async action creators and middleware
The npm package redux-mock-store receives a total of 1,220,691 weekly downloads. As such, redux-mock-store popularity was classified as popular.
We found that redux-mock-store demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 2 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
The MCP community is launching an official registry to standardize AI tool discovery and let agents dynamically find and install MCP servers.
Research
Security News
Socket uncovers an npm Trojan stealing crypto wallets and BullX credentials via obfuscated code and Telegram exfiltration.
Research
Security News
Malicious npm packages posing as developer tools target macOS Cursor IDE users, stealing credentials and modifying files to gain persistent backdoor access.