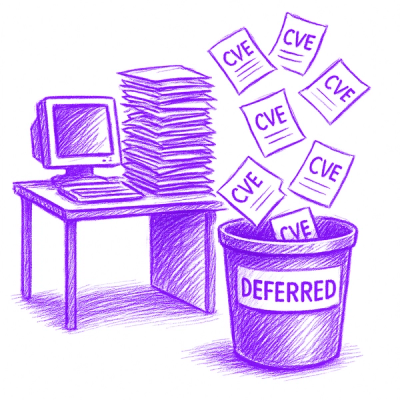
Security News
NVD Quietly Sweeps 100K+ CVEs Into a “Deferred” Black Hole
NVD now marks all pre-2018 CVEs as "Deferred," signaling it will no longer enrich older vulnerabilities, further eroding trust in its data.
redux-registry
Advanced tools
Because redux is amazing, but the verbosity (const definitions, switch statements in primary reducers, etc) and fragmentation of the redux definitions can be painful to implement. This module adds a heap of magic with just enough flexibility to be useful. It basically just removes the repetitive parts and simplifies the cutting and pasting.
npm install redux-registry
npm install --save react-redux redux
The basic steps are as follows:
let register = new ReduxRegister('todos')
name
(name of action), reduce
function and optionally a creator (simple creator functions that output something like { type: 'todos:addTodo', value: 'text' } will be automatically created). No "type" declaration necessary (this is why reducers are paired in the definition)! import { List } from 'immutable'
register
.setInitialState(List())
.add({
name: 'addTodo',
create: (value) => ({ value }), // this is created by default if not overwritten and may be omitted
reduce: (state, action) => state.push(action.value)
})
let registry = new ReduxRegistry
registry.add(register)
let action = registry.create('todos')('addTodo')('go to the store')
// assumes a state from somewhere, usually passed in from a redux store
state = registry.reduce(state, action)
// example state after execution:
// { todos: ['go to the store'] }
import { createStore } from 'redux'
import { combineReducers } from 'redux-immutable'
// import ReduxRegistry and extract reducers from shared instance
import ReduxRegistry from './registry'
let { reducers } = ReduxRegistry
// create redux state store with default state of Map()
const appReducer = combineReducers(reducers)
// define root reducer
const rootReducer = (state, action) => appReducer(state, action)
// create redux store
const store = createStore(
rootReducer,
Map(), // initial state
window.devToolsExtension ? window.devToolsExtension() : c => c
)
// use store like you normally would (e.g. in Provider)
ReactDOM.render(<Provider store={store}><App /></Provider>)
import { bindActionCreators } from 'redux'
import { connect } from 'react-redux'
import ReduxRegistry from 'redux-registry'
export default ReduxRegistry
.setConnect(connect) // internally sets "connect" function
.setBindActionCreators(bindActionCreators) // internally sets "bindActionCreators" function
// continue adding registers (shown above)
Then in a component:
import React, { Component } from 'react'
import { connect } from 'redux-registry'
export const App = ({ username, user }) => (
<div className="app">
<div>User: {username}</div>
<div>Age: {user.age} (can pull entire state branches or named nodes if using immutable)</div>
<button onClick={logoutAction}>Logout fires action dispatcher</button>
</div>
)
export default connect({
props: {
username: 'user.name',
user: 'user',
},
dispatchers: {
'logoutAction': 'user.logout'
}
})(App)
npm run test:watch
FAQs
Redux registry class for cleaner action+reducer definition
The npm package redux-registry receives a total of 73 weekly downloads. As such, redux-registry popularity was classified as not popular.
We found that redux-registry demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
NVD now marks all pre-2018 CVEs as "Deferred," signaling it will no longer enrich older vulnerabilities, further eroding trust in its data.
Research
Security News
Lazarus-linked threat actors expand their npm malware campaign with new RAT loaders, hex obfuscation, and over 5,600 downloads across 11 packages.
Security News
Safari 18.4 adds support for Iterator Helpers and two other TC39 JavaScript features, bringing full cross-browser coverage to key parts of the ECMAScript spec.