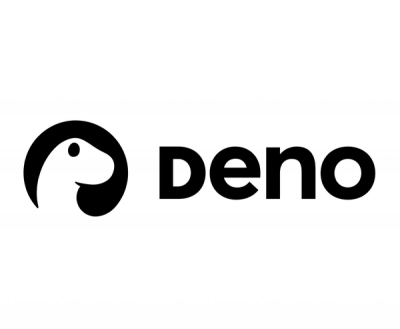
Security News
Deno 2.2 Improves Dependency Management and Expands Node.js Compatibility
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
redux-token-api-middleware
Advanced tools
Redux middleware for calling APIs with token-based auth
Alpha software - currently under heavy development
A redux middleware for making calls to APIs with token-based auth, automatically requesting a new token if needed. Actions can be calls to single or multiple API endpoints.
$ npm install redux-token-api-middleware
Actions that will be intercepted by the middleware are identified by the symbol
CALL_TOKEN_API
, and follow the Flux Standard Action pattern, however they must have a payload and have at least an endpoint
in the payload.
import { CALL_TOKEN_API } from 'redux-token-api-middleware'
export const BASIC_GET_ACTION = 'BASIC_GET_ACTION'
export const BASIC_POST_ACTION = 'BASIC_POST_ACTION'
// basic GET
// method defaults to GET if not provided
const basicGetAction = () => ({
[CALL_TOKEN_API]: {
type: BASIC_GET_ACTION,
payload: {
endpoint: 'http://localhost/foo'
}
}
})
// basic POST
const basicPostAction = () => ({
[CALL_TOKEN_API]: {
type: BASIC_POST_ACTION,
payload: {
endpoint: 'http://localhost/bar',
method: 'POST'
}
}
})
main.js
import { createTokenApiMiddleware } from 'redux-token-api-middleware'
import { createStore, applyMiddleware } from 'redux'
import reducer from './reducers'
// example refresh token action
const refreshAction = (token) => ({
[CALL_TOKEN_API]: {
type: 'REFRESH_TOKEN',
endpoint: 'http://localhost/token',
method: 'POST',
body: JSON.stringify(token)
}
})
You will need to provide a `retrieveRefreshToken` method to the config that will
return the refresh token that is used to obtain a new access token.
const retrieveRefreshToken = (key = 'RefreshTokenStorageKey') => {
// This return value is passed to `refreshAction`
return localStorage.getItem(key);
};
const config = {
refreshAction,
retrieveRefreshToken,
};
const apiTokenMiddleware = createTokenApiMiddleware(config)
const store = createStore(
reducer,
applyMiddleware(apiTokenMiddleware)
)
createTokenApiMiddleware(config)
Creates a Redux middleware to handle API objects.
In the config, you must define at least a refreshAction
method and a retrieveRefreshToken
method, which is used by the middleware to attempt to get a fresh token. This should be an API
action itself.
Other methods can be passed in via config, but have defaults:
// defaults shown
const config = {
defaultHeaders: { 'Content-Type': 'application/json' },
retrieveRefreshToken: () => {}; // To be implemented by the user for refreshing tokens.
tokenStorageKey: 'reduxMiddlewareAuthToken',
minTokenLifespan: 300, // seconds (min remaining lifespan to indicate new token should be requested)
storeToken: function storeToken(key, response) {
let token = response.token;
localStorage.setItem(key, JSON.stringify(token));
return token;
},
retrieveToken: function retrieveToken(key) {
let storedValue = localStorage.getItem(key);
if (!storedValue) {
return null;
}
try {
return JSON.parse(storedValue);
}
catch (e) {
if (e instanceof SyntaxError) {
return null;
}
throw e;
}
},
checkTokenFreshness: function checkTokenFreshness(token) {
let tokenPayload = jwt_decode(token);
let expiry = moment.unix(tokenPayload.exp);
return expiry.diff(moment(), 'seconds') > MIN_TOKEN_LIFESPAN;
},
shouldRequestNewToken: function shouldRequestNewToken() {
const token = retrieveToken();
return token
? checkTokenFreshness(token)
: false;
},
addTokenToRequest: function defaultAddTokenToRequest(headers, endpoint, body, token) {
return {
headers: Object.assign({
Authorization: `JWT ${token}`
}, headers),
endpoint,
body
}
}
}
Note: retrieveRefreshToken
, storeToken
and retrieveToken
methods may also return
promises that the middleware will resolve.
FAQs
Redux middleware for calling APIs with token-based auth
The npm package redux-token-api-middleware receives a total of 5 weekly downloads. As such, redux-token-api-middleware popularity was classified as not popular.
We found that redux-token-api-middleware demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
Security News
React's CRA deprecation announcement sparked community criticism over framework recommendations, leading to quick updates acknowledging build tools like Vite as valid alternatives.
Security News
Ransomware payment rates hit an all-time low in 2024 as law enforcement crackdowns, stronger defenses, and shifting policies make attacks riskier and less profitable.