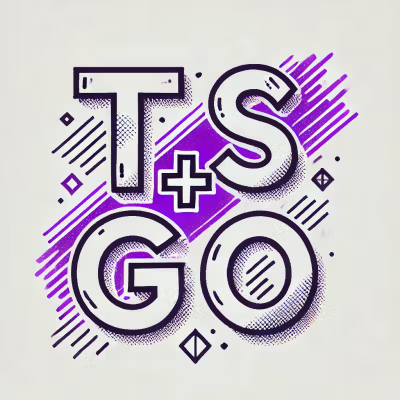
Security News
TypeScript is Porting Its Compiler to Go for 10x Faster Builds
TypeScript is porting its compiler to Go, delivering 10x faster builds, lower memory usage, and improved editor performance for a smoother developer experience.
regexp-to-ast
Advanced tools
The `regexp-to-ast` npm package is a utility that converts regular expressions into Abstract Syntax Trees (ASTs). This can be useful for analyzing, transforming, or generating regular expressions programmatically.
Convert RegExp to AST
This feature allows you to convert a regular expression into its corresponding Abstract Syntax Tree (AST). The AST can then be used for further analysis or transformation.
const { regExpParser } = require('regexp-to-ast');
const ast = regExpParser.pattern('/a*b+/');
console.log(JSON.stringify(ast, null, 2));
Analyze AST
This feature demonstrates how to traverse and analyze the AST generated from a regular expression. You can inspect different nodes and their properties.
const { regExpParser } = require('regexp-to-ast');
const ast = regExpParser.pattern('/a*b+/');
function analyzeAST(node) {
if (node.type === 'Character') {
console.log(`Character: ${node.value}`);
}
if (node.children) {
node.children.forEach(analyzeAST);
}
}
analyzeAST(ast);
The `regexpp` package is a regular expression parser for ECMAScript. It provides a way to parse regular expressions into ASTs and offers utilities for AST traversal and manipulation. Compared to `regexp-to-ast`, `regexpp` supports a broader range of ECMAScript regular expression features and provides more detailed AST nodes.
The `regexp-tree` package is a toolkit for working with regular expressions. It includes a parser that converts regular expressions into ASTs, as well as utilities for transforming and generating regular expressions. `regexp-tree` offers a more comprehensive set of tools for working with regular expressions compared to `regexp-to-ast`.
Reads a JavaScript Regular Expression literal(text) and outputs an Abstract Syntax Tree.
npm install regexp-to-ast
<script src="https://unpkg.com/regexp-to-ast/lib/parser.js"></script>
The API is defined as a TypeScript definition file.
Parsing to an AST:
const RegExpParser = require("regexp-to-ast").RegExpParser
const regexpParser = new RegExpParser.parser()
// from a regexp text
const astOutput = regexpParser.pattern("/a|b|c/g")
// text from regexp instance.
const input2 = /a|b/.toString()
// The same parser instance can be reused
const anotherAstOutput = regexpParser.pattern(input2)
Visiting the AST:
// parse to an AST as before.
const { RegExpParser, BaseRegExpVisitor } = require("regexp-to-ast")
const regexpParser = new RegExpParser.parser()
const regExpAst = regexpParser.pattern("/a|b|c/g")
// Override the visitor methods to add your logic.
class MyRegExpVisitor extends BaseRegExpVisitor {
visitPattern(node) {}
visitFlags(node) {}
visitDisjunction(node) {}
visitAlternative(node) {}
// Assertion
visitStartAnchor(node) {}
visitEndAnchor(node) {}
visitWordBoundary(node) {}
visitNonWordBoundary(node) {}
visitLookahead(node) {}
visitNegativeLookahead(node) {}
// atoms
visitCharacter(node) {}
visitSet(node) {}
visitGroup(node) {}
visitGroupBackReference(node) {}
visitQuantifier(node) {}
}
const myVisitor = new MyRegExpVisitor()
myVisitor.visit(regExpAst)
// extract visit results from the visitor state.
This library is written in ES5 style and is compatiable with all major browsers and modern node.js versions.
0.5.0 (11-12-2019)
FAQs
Parses a Regular Expression and outputs an AST
We found that regexp-to-ast demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
TypeScript is porting its compiler to Go, delivering 10x faster builds, lower memory usage, and improved editor performance for a smoother developer experience.
Research
Security News
The Socket Research Team has discovered six new malicious npm packages linked to North Korea’s Lazarus Group, designed to steal credentials and deploy backdoors.
Security News
Socket CEO Feross Aboukhadijeh discusses the open web, open source security, and how Socket tackles software supply chain attacks on The Pair Program podcast.