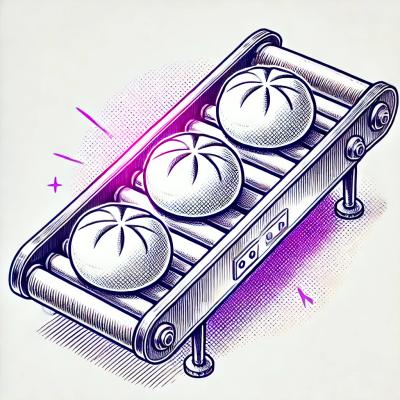
Security News
Bun 1.2 Released with 90% Node.js Compatibility and Built-in S3 Object Support
Bun 1.2 enhances its JavaScript runtime with 90% Node.js compatibility, built-in S3 and Postgres support, HTML Imports, and faster, cloud-first performance.
rekognition-node
Advanced tools
This is a fork of node-rekognition by oleurud
Use this if you need to ability to send AWS image bytes
npm install rekognition-node
const Rekognition = require('rekognition-node');
// Set your AWS credentials
const AWSParameters = {
accessKeyId: 'XXX',
secretAccessKey: 'XXX',
region: 'XXX',
bucket: 'XXX',
ACL: 'XXX' // optional
};
const rekognition = new Rekognition(AWSParameters);
The ACL is optional and its possible values are: "private", "public-read", "public-read-write", "authenticated-read", "aws-exec-read", "bucket-owner-read", "bucket-owner-full-control" More info
Some methods from AWS Rekognition need one or more images uploaded to AWS S3 bucket
/**
* Upload image or images array to S3 bucket into specified folder
*
* @param {Array.<string>|string} imagePaths
* @param {string} folder a folder name inside your AWS S3 bucket (it will be created if not exists)
*/
const s3Images = await rekognition.uploadToS3(imagePaths, folder)
/**
* Detects instances of real-world labels within an image
*
* @param {Object|Buffer} image
* @param {string} threshold (optional. Defaults 50)
*/
const imageLabels = await rekognition.detectLabels(image)
/**
* Detects faces within an image
*
* @param {Object|Buffer} image
*/
const imageFaces = await rekognition.detectFaces(image)
/**
* Compares a face in the source input image with each face detected in the target input image
*
* @param {Object|Buffer} sourceImage
* @param {Object|Buffer} targetImage
* @param {string} threshold (optional. Defaults 90)
*/
const faceMatches = await rekognition.compareFaces(sourceImage, targetImage, threshold)
/**
* Detects explicit or suggestive adult content in image
*
* @param {Object|Buffer} image
* @param {number} threshold (optional. Defaults 50)
*/
const moderationLabels = await rekognition.detectModerationLabels(image, threshold)
/**
* Creates a collection
*
* @param {string} collectionId
*/
const collection = await rekognition.createCollection(collectionId)
/**
* Deletes a collection
*
* @param {string} collectionId
*/
const collection = await rekognition.deleteCollection(collectionId)
/**
* Detects faces in the input image and adds them to the specified collection
*
* @param {string} collectionId
* @param {Object} s3Image
*/
const facesIndexed = await rekognition.indexFaces(collectionId, s3Image)
/**
* List the metadata for faces indexed in the specified collection
*
* @param {string} collectionId
*/
const faces = await rekognition.listFaces(collectionId)
/**
* Searches in the collection for matching faces of faceId
*
* @param {string} collectionId
* @param {string} faceId
* @param {number} threshold (optional. Defaults 90)
*/
const faceMatches = await rekognition.searchFacesByFaceId(collectionId, faceId, threshold)
/**
* First detects the largest face in the image (indexes it), and then searches the specified collection for matching faces.
*
* @param {string} collectionId
* @param {Object} s3Image
* @param {number} threshold (optional. Defaults 90)
*/
const faceMatches = await rekognition.searchFacesByImage(collectionId, s3Image, threshold)
First of all, you must create a parameters.json file and set your AWS parameters. You have an example file parametrs.json.example
Then:
Releases are documented in the NEWS file
node >= 7.10.0
You are welcome contribute via pull requests.
http://docs.aws.amazon.com/rekognition/latest/dg/API_Operations.html http://docs.aws.amazon.com/AWSJavaScriptSDK/latest/AWS/Rekognition.html
FAQs
AWS Rekognition library
We found that rekognition-node demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Bun 1.2 enhances its JavaScript runtime with 90% Node.js compatibility, built-in S3 and Postgres support, HTML Imports, and faster, cloud-first performance.
Security News
Biden's executive order pushes for AI-driven cybersecurity, software supply chain transparency, and stronger protections for federal and open source systems.
Security News
Fluent Assertions is facing backlash after dropping the Apache license for a commercial model, leaving users blindsided and questioning contributor rights.