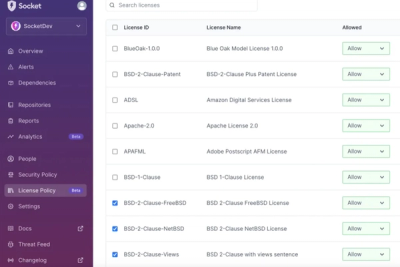
Product
Introducing License Enforcement in Socket
Ensure open-source compliance with Socket’s License Enforcement Beta. Set up your License Policy and secure your software!
Simple Elasticsearch query builder
$ npm install relastic
Relastic uses method chaining to construct Elasticsearch queries.
var Relastic = require('relastic')
var r = new Relastic()
r.query().match({title: 'thai chili'})
r.output()
// {
// query: {
// match: {
// title: 'thai chilies'
// }
// }
// }
This approach can be much simpler than constructing large ES queries when compared to explicitly defining and modifying complex objects.
r.filter()
.bool()
.must()
.term({type: 'chili pepper'})
.terms({spiciness: ['high', 'very high']})
.should()
.term({color: 'green'})
.term({size: 'small'})
r.output()
// {
// query: {},
// filter: {
// bool: {
// must: [
// {
// term: {
// type: 'chili pepper'
// }
// },
// {
// terms: {
// spiciness: ['high', 'very high']}
// }
// }
// ],
// should: [
// {
// term: {
// color: 'green'
// }
// },
// {
// term: {
// size: 'small'
// }
// }
// ]
// }
// }
// }
Relastic also allows construction of query objects progressively.
r.filteredQuery()
.query()
.match({ingredients: 'cayenne'})
r.filteredQuery()
.filter()
.term({type: 'soup'})
r.output()
// {
// query: {
// filtered: {
// query: {
// match: {
// ingredients: 'cayenne'
// }
// },
// filter: {
// term: {
// type: 'soup'
// }
// }
// }
// }
// }
Each invocation of a Relastic query constructor method returns a chainable instance of Relastic. The chain can be started anew at whatever point the final method invocation referred to.
var bool = r.filter().bool()
bool.must().term({color: 'green'})
bool.must_not().terms({spiciness: ['none', 'low', 'medium']})
r.output()
// {
// query: {},
// filter: {
// bool : {
// must: {
// term: {
// color: 'green'
// }
// },
// must_not: {
// terms: {
// spiciness: ['none', 'low', 'medium']
// }
// }
// }
// }
// }
FAQs
Elasticsearch query builder
The npm package relastic receives a total of 0 weekly downloads. As such, relastic popularity was classified as not popular.
We found that relastic demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Product
Ensure open-source compliance with Socket’s License Enforcement Beta. Set up your License Policy and secure your software!
Product
We're launching a new set of license analysis and compliance features for analyzing, managing, and complying with licenses across a range of supported languages and ecosystems.
Product
We're excited to introduce Socket Optimize, a powerful CLI command to secure open source dependencies with tested, optimized package overrides.