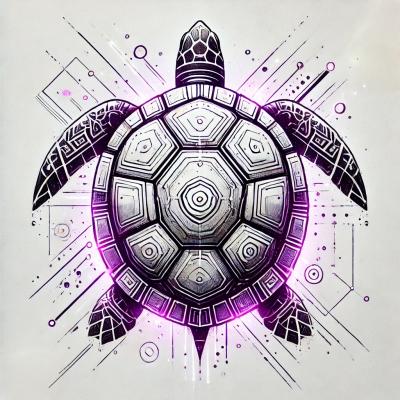
Security Fundamentals
Turtles, Clams, and Cyber Threat Actors: Shell Usage
The Socket Threat Research Team uncovers how threat actors weaponize shell techniques across npm, PyPI, and Go ecosystems to maintain persistence and exfiltrate data.
remote-controllers-manager
Advanced tools
An experimental package that makes easy manage devices that can act as a remote control of your web app through an websocket connection.
This package creates an abstraction on top of socket.io to easily manage devices (smartphones for example) that can act as a remote controller of you web app, similar to how AirConsole works.
This is a super experimental package ⚠, I'm building it for personal usage in a project but if it gets good enough may I publish to npm and make some demos.
Examples of apps I have in mind:
yarn add remote-controllers-manager socket.io socket.io-client
import * as io from 'socket.io'
import { applyRCMMiddleware } from 'remote-controllers-manager/server'
import { green, blue } from 'colors'
const server = io.listen(3000)
applyRCMMiddleware(server, {
// You can configure some behaviors.
maxControllersPerRoom: 4,
eachRoomNeedsAMasterController: true,
ifMasterControllerDisconnects: 'waitReconnect',
})
console.log(green('⚡ Listening on port http://localhost:3000'))
Screen
class, like this:Note that you will need a bundler.
// screen.js
import * as io from 'socket.io-client'
import { Screen } from 'remote-controllers-manager/client'
const screen = new Screen({
io,
uri: 'http://localhost:3000',
})
screen.start().then(() => {
console.log('Successfully connected to server!')
// Show screen id so user can connect
document.body.innerHTML = `SCREEN_ID = ${screen.deviceId}`
})
Controller
class:// controller.js
import * as io from 'socket.io-client'
import { Controller } from 'remote-controllers-manager/client'
const controller = new Controller({
io,
uri: 'http://localhost:3000',
})
controller.connectToScreen('<SCREEN_ID>').then(() => {
console.log('Successfully connected to screen!')
})
Just that, the package will take care of managing all controllers that connect in the room.
You can now easily send messages to all connected devices, like that:
// controller.js
// ...
document.querySelector('button').addEventListener('click', () => {
controller.sendToScreen({ eventName: 'change_title' })
})
// screen.js
// ...
screen.onMessage(({ eventName, data }) => {
if (eventName === 'change_title') {
// ...
}
})
screen.onConnect(() => {
screen.broadcastToControllers({ eventName: 'new_controller' })
})
The documentation is not written yet because the package needs to be refined, but you can see what the final API will looks like in this file.
MIT License
FAQs
An experimental package that makes easy manage devices that can act as a remote control of your web app through an websocket connection.
The npm package remote-controllers-manager receives a total of 3 weekly downloads. As such, remote-controllers-manager popularity was classified as not popular.
We found that remote-controllers-manager demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security Fundamentals
The Socket Threat Research Team uncovers how threat actors weaponize shell techniques across npm, PyPI, and Go ecosystems to maintain persistence and exfiltrate data.
Security News
At VulnCon 2025, NIST scrapped its NVD consortium plans, admitted it can't keep up with CVEs, and outlined automation efforts amid a mounting backlog.
Product
We redesigned our GitHub PR comments to deliver clear, actionable security insights without adding noise to your workflow.