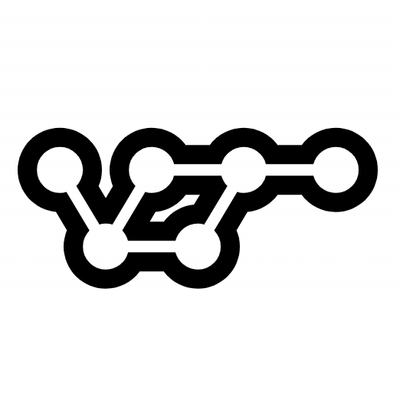
Security News
vlt Launches "reproduce": A New Tool Challenging the Limits of Package Provenance
vlt's new "reproduce" tool verifies npm packages against their source code, outperforming traditional provenance adoption in the JavaScript ecosystem.
remove-empty-divs
Advanced tools
remove-empty-divs
A simple utility function to remove empty div
elements from the current HTML page. This package is designed for use in web applications where cleaning up empty div
elements is needed.
You can install the package via npm if it's published:
npm install remove-empty-divs
Or, if you're using it locally for development or testing, you can link it:
Navigate to the package directory and run:
npm link
In your React application directory, run:
npm link remove-empty-divs
Import the Function
Import the removeEmptyDivs
function into your React component:
import removeEmptyDivs from "remove-empty-divs";
Use the Function
Call the function within a useEffect
hook to ensure it runs after the component mounts:
import React, { useEffect } from "react";
import removeEmptyDivs from "remove-empty-divs";
const App: React.FC = () => {
useEffect(() => {
// Call the function to remove empty divs
removeEmptyDivs();
}, []);
return (
<div className="App">
<div>
<p>Non-empty div</p>
</div>
<div></div>
<div>
<span>Another non-empty div</span>
</div>
<div></div>
</div>
);
};
export default App;
Import the Function
import removeEmptyDivs from "remove-empty-divs";
Use the Function
Call the function in your JavaScript code:
document.addEventListener("DOMContentLoaded", () => {
removeEmptyDivs();
});
removeEmptyDivs()
This function iterates over all div
elements in the current HTML page and removes those that do not contain any child nodes.
Contributions are welcome! Please follow these steps to contribute:
This project is licensed under the MIT License. See the LICENSE file for details.
Feel free to modify and extend this README to better fit your project's needs.
removeEmptyDivs
function.FAQs
A package to remove empty divs from the current HTML page
The npm package remove-empty-divs receives a total of 5 weekly downloads. As such, remove-empty-divs popularity was classified as not popular.
We found that remove-empty-divs demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
vlt's new "reproduce" tool verifies npm packages against their source code, outperforming traditional provenance adoption in the JavaScript ecosystem.
Research
Security News
Socket researchers uncovered a malicious PyPI package exploiting Deezer’s API to enable coordinated music piracy through API abuse and C2 server control.
Research
The Socket Research Team discovered a malicious npm package, '@ton-wallet/create', stealing cryptocurrency wallet keys from developers and users in the TON ecosystem.