remove-markdown
Advanced tools
Comparing version
18
index.js
@@ -7,5 +7,9 @@ module.exports = function(md, options) { | ||
var output = md; | ||
// Remove horizontal rules (stripListHeaders conflict with this rule, which is why it has been moved to the top) | ||
output = output.replace(/^(-\s*?|\*\s*?|_\s*?){3,}\s*$/gm, ''); | ||
try { | ||
if (options.stripListLeaders) { | ||
output = output.replace(/^([\s\t]*)([\*\-\+]|\d\.)\s+/gm, '$1'); | ||
output = output.replace(/^([\s\t]*)([\*\-\+]|\d+\.)\s+/gm, '$1'); | ||
} | ||
@@ -23,3 +27,3 @@ if (options.gfm){ | ||
// Remove HTML tags | ||
.replace(/<(.*?)>/g, '$1') | ||
.replace(/<[^>]*>/g, '') | ||
// Remove setext-style headers | ||
@@ -39,7 +43,11 @@ .replace(/^[=\-]{2,}\s*$/g, '') | ||
// Remove atx-style headers | ||
.replace(/^\#{1,6}\s*([^#]*)\s*(\#{1,6})?/gm, '$1') | ||
.replace(/([\*_]{1,3})(\S.*?\S)\1/g, '$2') | ||
.replace(/^(\n)?\s{0,}#{1,6}\s+| {0,}(\n)?\s{0,}#{0,} {0,}(\n)?\s{0,}$/gm, '$1$2$3') | ||
// Remove emphasis (repeat the line to remove double emphasis) | ||
.replace(/([\*_]{1,3})(\S.*?\S{0,1})\1/g, '$2') | ||
.replace(/([\*_]{1,3})(\S.*?\S{0,1})\1/g, '$2') | ||
// Remove code blocks | ||
.replace(/(`{3,})(.*?)\1/gm, '$2') | ||
.replace(/^-{3,}\s*$/g, '') | ||
// Remove inline code | ||
.replace(/`(.+?)`/g, '$1') | ||
// Replace two or more newlines with exactly two? Not entirely sure this belongs here... | ||
.replace(/\n{2,}/g, '\n\n'); | ||
@@ -46,0 +54,0 @@ } catch(e) { |
{ | ||
"name": "remove-markdown", | ||
"version": "0.1.0", | ||
"version": "0.2.0", | ||
"description": "Remove Markdown formatting from text", | ||
@@ -23,2 +23,3 @@ "main": "index.js", | ||
"devDependencies": { | ||
"chai": "^4.0.2", | ||
"mocha": "^2.1.0", | ||
@@ -25,0 +26,0 @@ "should": "^5.0.0" |
@@ -15,8 +15,8 @@ ## What is it? | ||
```js | ||
var removeMd = require('remove-markdown'); | ||
var markdown = '# This is a heading\n\nThis is a paragraph with [a link](http://www.disney.com/) in it.'; | ||
var plainText = removeMd(markdown); // plainText is now 'This is a heading\n\nThis is a paragraph with a link in it.' | ||
const removeMd = require('remove-markdown'); | ||
const markdown = '# This is a heading\n\nThis is a paragraph with [a link](http://www.disney.com/) in it.'; | ||
const plainText = removeMd(markdown); // plainText is now 'This is a heading\n\nThis is a paragraph with a link in it.' | ||
``` | ||
You can also supply an options object to the function. Currently, the only two options are for stripping list headers and supporting Github Flavored Markdown: | ||
You can also supply an options object to the function. Currently, the only two options are for stripping list leaders and supporting Github Flavored Markdown: | ||
@@ -30,4 +30,10 @@ ```js | ||
Stripping list headers will retain any list characters (`*, -, +, (digit).`). | ||
Setting `stripListLeaders` to false will retain any list characters (`*, -, +, (digit).`). | ||
## TODO | ||
PRs are very much welcome. | ||
* Allow the RegEx expressions to be customized per rule | ||
* Make the rules more robust, support more edge cases | ||
* Add more (comprehensive) tests | ||
## Credits | ||
@@ -34,0 +40,0 @@ The code is based on [Markdown Service Tools - Strip Markdown](http://brettterpstra.com/2013/10/18/a-markdown-service-to-strip-markdown/) by Brett Terpstra. |
'use strict'; | ||
const expect = require('chai').expect; | ||
const removeMd = require('../'); | ||
var fs = require('fs'), | ||
path = require('path'), | ||
should = require('should'), | ||
removeMd = require('../'), | ||
markdown = fs.readFileSync(path.resolve(__dirname, 'markdown.md')).toString(), | ||
result = fs.readFileSync(path.resolve(__dirname, 'result.txt')).toString(); | ||
describe('remove Markdown', function () { | ||
describe('removeMd', function () { | ||
it('should leave a string alone without markdown', function () { | ||
const string = 'Javascript Developers are the best.'; | ||
expect(removeMd(string)).to.equal(string); | ||
}); | ||
describe('remove-markdown', function () { | ||
it('should remove markdown', function () { | ||
removeMd(markdown).should.eql(result); | ||
it('should strip out remaining markdown', function () { | ||
const string = '*Javascript* developers are the _best_.'; | ||
const expected = 'Javascript developers are the best.'; | ||
expect(removeMd(string)).to.equal(expected); | ||
}); | ||
it('should leave non-matching markdown markdown', function () { | ||
const string = '*Javascript* developers* are the _best_.'; | ||
const expected = 'Javascript developers* are the best.'; | ||
expect(removeMd(string)).to.equal(expected); | ||
}); | ||
it('should leave non-matching markdown, but strip empty anchors', function () { | ||
const string = '*Javascript* [developers]()* are the _best_.'; | ||
const expected = 'Javascript developers* are the best.'; | ||
expect(removeMd(string)).to.equal(expected); | ||
}); | ||
it('should strip HTML', function () { | ||
const string = '<p>Hello World</p>'; | ||
const expected = 'Hello World'; | ||
expect(removeMd(string)).to.equal(expected); | ||
}); | ||
it('should strip anchors', function () { | ||
const string = '*Javascript* [developers](https://engineering.condenast.io/)* are the _best_.'; | ||
const expected = 'Javascript developers* are the best.'; | ||
expect(removeMd(string)).to.equal(expected); | ||
}); | ||
it('should strip img tags', function () { | ||
const string = '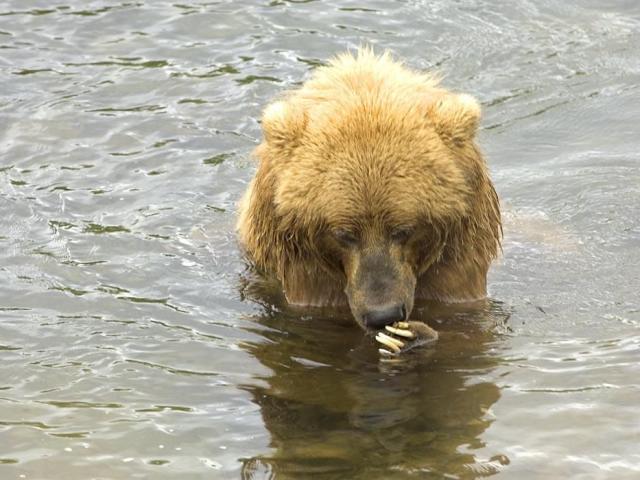*Javascript* developers are the _best_.'; | ||
const expected = 'Javascript developers are the best.'; | ||
expect(removeMd(string)).to.equal(expected); | ||
}); | ||
it('should strip code tags', function () { | ||
const string = 'In `Getting Started` we set up `something` foo.'; | ||
const expected = 'In Getting Started we set up something foo.'; | ||
expect(removeMd(string)).to.equal(expected); | ||
}); | ||
it('should leave hashtags in headings', function () { | ||
const string = '## This #heading contains #hashtags'; | ||
const expected = 'This #heading contains #hashtags'; | ||
expect(removeMd(string)).to.equal(expected); | ||
}); | ||
it('should remove emphasis', function () { | ||
const string = 'I italicized an *I* and it _made_ me *sad*.'; | ||
const expected = 'I italicized an I and it made me sad.'; | ||
expect(removeMd(string)).to.equal(expected); | ||
}); | ||
it('should remove double emphasis', function () { | ||
const string = '**this sentence has __double styling__**'; | ||
const expected = 'this sentence has double styling'; | ||
expect(removeMd(string)).to.equal(expected); | ||
}); | ||
it('should remove horizontal rules', function () { | ||
const string = 'Some text on a line\n\n---\n\nA line below'; | ||
const expected = 'Some text on a line\n\nA line below'; | ||
expect(removeMd(string)).to.equal(expected); | ||
}); | ||
it('should remove horizontal rules with space-separated asterisks', function () { | ||
const string = 'Some text on a line\n\n* * *\n\nA line below'; | ||
const expected = 'Some text on a line\n\nA line below'; | ||
expect(removeMd(string)).to.equal(expected); | ||
}); | ||
it('should strip unordered list leaders', function () { | ||
const string = 'Some text on a line\n\n* A list Item\n* Another list item'; | ||
const expected = 'Some text on a line\n\nA list Item\nAnother list item'; | ||
expect(removeMd(string)).to.equal(expected); | ||
}); | ||
it('should strip ordered list leaders', function () { | ||
const string = 'Some text on a line\n\n9. A list Item\n10. Another list item'; | ||
const expected = 'Some text on a line\n\nA list Item\nAnother list item'; | ||
expect(removeMd(string)).to.equal(expected); | ||
}); | ||
it('should handle paragraphs with markdown', function () { | ||
const paragraph = '\n## This is a heading ##\n\nThis is a paragraph with [a link](http://www.disney.com/).\n\n### This is another heading\n\nIn `Getting Started` we set up `something` foo.\n\n * Some list\n * With items\n * Even indented'; | ||
const expected = '\nThis is a heading\n\nThis is a paragraph with a link.\n\nThis is another heading\n\nIn Getting Started we set up something foo.\n\n Some list\n With items\n Even indented'; | ||
expect(removeMd(paragraph)).to.equal(expected); | ||
}); | ||
}); | ||
}); | ||
}); |
URL strings
Supply chain riskPackage contains fragments of external URLs or IP addresses, which the package may be accessing at runtime.
Found 1 instance in 1 package
Filesystem access
Supply chain riskAccesses the file system, and could potentially read sensitive data.
Found 1 instance in 1 package
URL strings
Supply chain riskPackage contains fragments of external URLs or IP addresses, which the package may be accessing at runtime.
Found 1 instance in 1 package
9753
81.42%139
139.66%42
16.67%1
-50%3
50%6
-25%