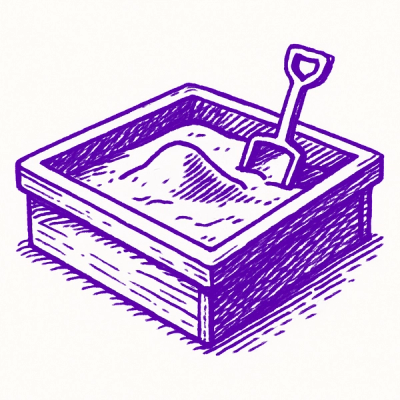
Research
/Security News
Critical Vulnerability in NestJS Devtools: Localhost RCE via Sandbox Escape
A flawed sandbox in @nestjs/devtools-integration lets attackers run code on your machine via CSRF, leading to full Remote Code Execution (RCE).
remove-trailing-slash
Advanced tools
The 'remove-trailing-slash' npm package is a utility that helps in removing trailing slashes from URLs or strings. This can be particularly useful in web development where consistent URL formatting is important for routing, SEO, and avoiding duplicate content issues.
Remove trailing slash from a single URL
This feature allows you to remove the trailing slash from a single URL string. It ensures that the URL is consistently formatted without a trailing slash.
const removeTrailingSlash = require('remove-trailing-slash');
const url = 'https://example.com/';
const cleanUrl = removeTrailingSlash(url);
console.log(cleanUrl); // 'https://example.com'
Remove trailing slash from multiple URLs
This feature demonstrates how to remove trailing slashes from an array of URLs. It uses the map function to apply the removeTrailingSlash function to each URL in the array.
const removeTrailingSlash = require('remove-trailing-slash');
const urls = ['https://example.com/', 'https://example.org/', 'https://example.net/'];
const cleanUrls = urls.map(url => removeTrailingSlash(url));
console.log(cleanUrls); // ['https://example.com', 'https://example.org', 'https://example.net']
The 'normalize-url' package is a more comprehensive solution for URL normalization. It not only removes trailing slashes but also handles other aspects like removing duplicate slashes, converting URLs to lowercase, and removing unnecessary query parameters. It is more feature-rich compared to 'remove-trailing-slash'.
The 'url-parse' package is a robust URL parsing library that allows you to manipulate and normalize URLs. While it doesn't specifically focus on removing trailing slashes, it provides a wide range of functionalities for URL manipulation, making it a versatile tool for developers.
removes trailing slashes
with component(1):
$ component install stephenmathieson/remove-trailing-slash
with npm:
$ npm install remove-trailing-slash
removeTrailingSlash(str)
Removes trailing slashes from the given str
var slashes = require('remove-trailing-slash')
slashes('http://google.com/').should.be.equal('http://google.com');
MIT
FAQs
removes trailing slashes
The npm package remove-trailing-slash receives a total of 918,919 weekly downloads. As such, remove-trailing-slash popularity was classified as popular.
We found that remove-trailing-slash demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
/Security News
A flawed sandbox in @nestjs/devtools-integration lets attackers run code on your machine via CSRF, leading to full Remote Code Execution (RCE).
Product
Customize license detection with Socket’s new license overlays: gain control, reduce noise, and handle edge cases with precision.
Product
Socket now supports Rust and Cargo, offering package search for all users and experimental SBOM generation for enterprise projects.