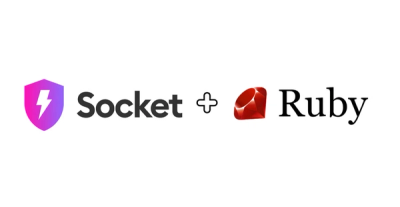
Product
Rubygems Ecosystem Support Now Generally Available
Socket's Rubygems ecosystem support is moving from beta to GA, featuring enhanced security scanning to detect supply chain threats beyond traditional CVEs in your Ruby dependencies.
replace-color-ts
Advanced tools
NOW WITH TS! Fully backwards-compatible
replace-color-ts
replaces color with another one pixel by pixel. Especially this will be helpful if you want to remove the watermarks from the images. This package is built on top of Jimp.
Kudos to Vladyslav Turak for the original JS version of this library.
npm install --save replace-color-ts
replace-color-ts
supports both Node.js error-first callbacks and promises. The package returns a Jimp's instance which you can use to execute some other image manipulations methods or save it with Jimp's write
method.
const replaceColor = require('replace-color-ts')
// or with import statement
// import replaceColor from 'replace-color-ts'
replaceColor({
image: './input.jpg',
colors: {
type: 'hex',
targetColor: '#FF0000',
replaceColor: '#FFFFFF'
}
}, (err, jimpObject) => {
if (err) return console.log(err)
jimpObject.write('./output.jpg', (err) => {
if (err) return console.log(err)
})
})
const replaceColor = require('replace-color-ts')
// or with import statement
// import replaceColor from 'replace-color-ts'
replaceColor({
image: './input.jpg',
colors: {
type: 'hex',
targetColor: '#FF0000',
replaceColor: '#FFFFFF'
}
})
.then((jimpObject) => {
jimpObject.write('./output.jpg', (err) => {
if (err) return console.log(err)
})
})
.catch((err) => {
console.log(err)
})
options
Object (required) - the options.
image
Buffer | Object | String (required) - an image being processed. It can be a buffer, Jimp's instance, a path to an image on your host machine or a URL address to an image on the internet. Please, take a look at the tests to understand all these options.colors
Object (required) - the colors.
type
String (required) - a targetColor
and replaceColor
type. Supported values are hex
and rgb
.targetColor
String | Array (required) - a color you want to replace. A 7-symbol string in case of hex
type (e.g. #000000
, #FFFFFF
). An array of 3
integers from 0
to 255
in case of rgb
type (e.g. [0, 0, 0]
, [255, 255, 255]
).replaceColor
String | Array (required) - a new color which will be used instead of a targetColor
color. A 7-symbol string in case of hex
type (e.g. #000000
, #FFFFFF
). An array of 3
integers from 0
to 255
in case of rgb
type (e.g. [0, 0, 0]
, [255, 255, 255]
). You can also define a transparent channel for a replaceColor
color. To achieve this, you can use a 9-symbol string in case of hex
type (e.g. #00000000
, #FFFFFFFF
). Based on this Stack Overflow answer, an alpha channel is controlled by the first pair of digits in a hex code (e.g., 00
means fully transparent, 7F
means 50%, FF
means fully opaque). Also, you can use an array of 4
integers in case of rgb
type. The first 3
integers must be from 0
to 255
and the last one must be from 0
to 1
(e.g., 0
means fully transparent, 0.5
means 50%, 1
means fully opaque).formula
String (optional) - one of the three formulas to calculate the color difference. Supported values are E76
, E94
and E00
. The default value is E00
(the best algorithm).deltaE
Number (optional) - a deltaE
value which corresponds to a JND. The default value is 2.3
. Please, read more about deltaE
here. Generaly speaking, if the processed by the replace-color-ts
package image still has the watermarks, you should increase the deltaE
value.callback
Function (optional) - a Node.js error-first callback.Let's try to remove a watermark from this picture.
const replaceColor = require('replace-color-ts')
replaceColor({
image: 'https://i.imgur.com/XqNTuzp.jpg',
colors: {
type: 'hex',
targetColor: '#FFB3B7',
replaceColor: '#FFFFFF'
},
deltaE: 20
})
.then((jimpObject) => {
jimpObject.write('./output.jpg', (err) => {
if (err) return console.log(err)
})
})
.catch((err) => {
console.log(err)
})
Let's try to change a background color for this picture.
const replaceColor = require('replace-color-ts')
replaceColor({
image: 'https://i.imgur.com/aCxZpaq.png',
colors: {
type: 'hex',
targetColor: '#66AE74',
replaceColor: '#63A4FF'
},
deltaE: 10
})
.then((jimpObject) => {
jimpObject.write('./output.png', (err) => {
if (err) return console.log(err)
})
})
.catch((err) => {
console.log(err)
})
hex
type)Let's try to change a background color for this picture.
const replaceColor = require('replace-color-ts')
replaceColor({
image: 'https://i.imgur.com/aCxZpaq.png',
colors: {
type: 'hex',
targetColor: '#66AE74',
replaceColor: '#00000000'
},
deltaE: 10
})
.then((jimpObject) => {
jimpObject.write('./output.png', (err) => {
if (err) return console.log(err)
})
})
.catch((err) => {
console.log(err)
})
rgb
type)Let's try to change a background color for this picture.
const replaceColor = require('replace-color-ts')
replaceColor({
image: 'https://i.imgur.com/aCxZpaq.png',
colors: {
type: 'rgb',
targetColor: [102, 174, 116],
replaceColor: [102, 174, 116, 0.5]
},
deltaE: 10
})
.then((jimpObject) => {
jimpObject.write('./output.png', (err) => {
if (err) return console.log(err)
})
})
.catch((err) => {
console.log(err)
})
To indicate the replace-color-ts
's errors you should use the err instanceof replaceColor.ReplaceColorError
class.
replaceColor({}, (err, jimpObject) => {
if (err instanceof replaceColor.ReplaceColorError) {
// A replace-color-ts's error occurred.
} else if (err) {
// An unknown error occurred.
}
// Everything went fine.
})
A replace-color-ts
's error
instance has the code
and field
properties. For now, the package has two codes: PARAMETER_INVALID
and PARAMETER_REQUIRED
. The field
property shows which exact property was not passed or is invalid using the glob notation (e.g. options.colors.type
). Please, take a look at the tests to see all the possible cases.
FAQs
Replace color with another one pixel by pixel.
The npm package replace-color-ts receives a total of 5 weekly downloads. As such, replace-color-ts popularity was classified as not popular.
We found that replace-color-ts demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Product
Socket's Rubygems ecosystem support is moving from beta to GA, featuring enhanced security scanning to detect supply chain threats beyond traditional CVEs in your Ruby dependencies.
Research
The Socket Research Team investigates a malicious npm package that appears to be an Advcash integration but triggers a reverse shell during payment success, targeting servers handling transactions.
Security Fundamentals
The Socket Threat Research Team uncovers how threat actors weaponize shell techniques across npm, PyPI, and Go ecosystems to maintain persistence and exfiltrate data.