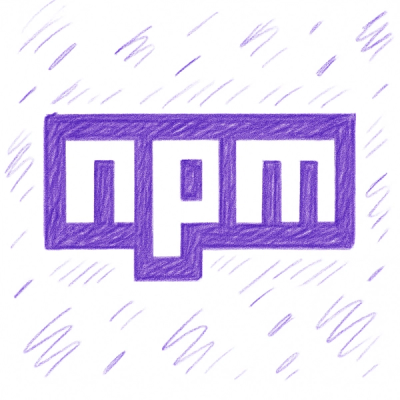
Security News
npm Adopts OIDC for Trusted Publishing in CI/CD Workflows
npm now supports Trusted Publishing with OIDC, enabling secure package publishing directly from CI/CD workflows without relying on long-lived tokens.
The rfdc (Really Fast Deep Clone) npm package is a module that provides a very fast deep cloning function for copying complex data structures in JavaScript. It is optimized for performance and can handle various types of data including objects, arrays, dates, and more.
Deep cloning objects
This feature allows you to create a deep clone of an object, meaning that all nested objects and arrays are also recursively cloned.
{"const rfdc = require('rfdc')();
const obj = { a: 1, b: { c: 2 } };
const clone = rfdc(obj);
console.log(clone); // { a: 1, b: { c: 2 } }"}
Deep cloning arrays
This feature allows you to create a deep clone of an array, including cloning all nested arrays and objects within it.
{"const rfdc = require('rfdc')();
const arr = [1, [2, 3], [4, 5]];
const clone = rfdc(arr);
console.log(clone); // [1, [2, 3], [4, 5]]"}
Customizing clone behavior
This feature allows you to customize the cloning behavior, such as whether to preserve the prototype chain or handle circular references.
{"const rfdc = require('rfdc')({ proto: false, circles: false });
const obj = { a: 1 };
obj.b = obj;
// Throws an error because 'circles: false' does not allow circular references.
const clone = rfdc(obj);"}
Lodash is a popular utility library that includes a deep cloning function (_.cloneDeep). It is more feature-rich than rfdc but may be slower for deep cloning large objects.
Clone-deep is another npm package that provides deep cloning functionality. It offers a similar feature set to rfdc but with different performance characteristics and additional options for cloning.
Deep-copy is a package that also allows for deep cloning of objects and arrays. It compares to rfdc in terms of functionality but may have different performance trade-offs.
Really Fast Deep Clone
const clone = require('rfdc')()
clone({a: 1, b: {c: 2}}) // => {a: 1, b: {c: 2}}
require('rfdc')(opts = { proto: false, circles: false, constructorHandlers: [] }) => clone(obj) => obj2
proto
optionCopy prototype properties as well as own properties into the new object.
It's marginally faster to allow enumerable properties on the prototype to be copied into the cloned object (not onto it's prototype, directly onto the object).
To explain by way of code:
require('rfdc')({ proto: false })(Object.create({a: 1})) // => {}
require('rfdc')({ proto: true })(Object.create({a: 1})) // => {a: 1}
Setting proto
to true
will provide an additional 2% performance boost.
circles
optionKeeping track of circular references will slow down performance with an
additional 25% overhead. Even if an object doesn't have any circular references,
the tracking overhead is the cost. By default if an object with a circular
reference is passed to rfdc
, it will throw (similar to how JSON.stringify
would throw).
Use the circles
option to detect and preserve circular references in the
object. If performance is important, try removing the circular reference from
the object (set to undefined
) and then add it back manually after cloning
instead of using this option.
constructorHandlers
optionSometimes consumers may want to add custom clone behaviour for particular classes
(for example RegExp
or ObjectId
, which aren't supported out-of-the-box).
This can be done by passing constructorHandlers
, which takes an array of tuples,
where the first item is the class to match, and the second item is a function that
takes the input and returns a cloned output:
const clone = require('rfdc')({
constructorHandlers: [
[RegExp, (o) => new RegExp(o)],
]
})
clone({r: /foo/}) // => {r: /foo/}
NOTE: For performance reasons, the handlers will only match an instance of the exact class (not a subclass). Subclasses will need to be added separately if they also need special clone behaviour.
default
importIt is also possible to directly import the clone function with all options set to their default:
const clone = require("rfdc/default")
clone({a: 1, b: {c: 2}}) // => {a: 1, b: {c: 2}}
rfdc
clones all JSON types:
Object
Array
Number
String
null
With additional support for:
Date
(copied)undefined
(copied)Buffer
(copied)TypedArray
(copied)Map
(copied)Set
(copied)Function
(referenced)AsyncFunction
(referenced)GeneratorFunction
(referenced)arguments
(copied to a normal object)All other types have output values that match the output
of JSON.parse(JSON.stringify(o))
.
For instance:
const rfdc = require('rfdc')()
const err = Error()
err.code = 1
JSON.parse(JSON.stringify(e)) // {code: 1}
rfdc(e) // {code: 1}
JSON.parse(JSON.stringify({rx: /foo/})) // {rx: {}}
rfdc({rx: /foo/}) // {rx: {}}
npm run bench
benchDeepCopy*100: 671.675ms
benchLodashCloneDeep*100: 1.574s
benchCloneDeep*100: 936.792ms
benchFastCopy*100: 822.668ms
benchFastestJsonCopy*100: 363.898ms // See note below
benchPlainObjectClone*100: 556.635ms
benchNanoCopy*100: 770.234ms
benchRamdaClone*100: 2.695s
benchJsonParseJsonStringify*100: 2.290s // JSON.parse(JSON.stringify(obj))
benchRfdc*100: 412.818ms
benchRfdcProto*100: 424.076ms
benchRfdcCircles*100: 443.357ms
benchRfdcCirclesProto*100: 465.053ms
It is true that fastest-json-copy may be faster, BUT it has such huge limitations that it is rarely useful. For example, it treats things like Date
and Map
instances the same as empty {}
. It can't handle circular references. plain-object-clone is also really limited in capability.
npm test
169 passing (342.514ms)
npm run cov
----------|----------|----------|----------|----------|-------------------|
File | % Stmts | % Branch | % Funcs | % Lines | Uncovered Line #s |
----------|----------|----------|----------|----------|-------------------|
All files | 100 | 100 | 100 | 100 | |
index.js | 100 | 100 | 100 | 100 | |
----------|----------|----------|----------|----------|-------------------|
__proto__
own property copyingrfdc
works the same way as Object.assign
when it comes to copying ['__proto__']
(e.g. when
an object has an own property key called 'proto'). It results in the target object
prototype object being set per the value of the ['__proto__']
own property.
For detailed write-up on how a way to handle this security-wise see https://www.fastify.io/docs/latest/Guides/Prototype-Poisoning/.
MIT
FAQs
Really Fast Deep Clone
The npm package rfdc receives a total of 25,350,641 weekly downloads. As such, rfdc popularity was classified as popular.
We found that rfdc demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 2 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
npm now supports Trusted Publishing with OIDC, enabling secure package publishing directly from CI/CD workflows without relying on long-lived tokens.
Research
/Security News
A RubyGems malware campaign used 60 malicious packages posing as automation tools to steal credentials from social media and marketing tool users.
Security News
The CNA Scorecard ranks CVE issuers by data completeness, revealing major gaps in patch info and software identifiers across thousands of vulnerabilities.