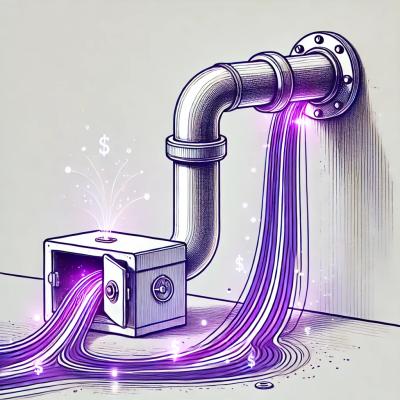
Research
Security News
Malicious npm Package Targets Solana Developers and Hijacks Funds
A malicious npm package targets Solana developers, rerouting funds in 2% of transactions to a hardcoded address.
rich-textarea
Advanced tools
A small customizable textarea for React to colorize, highlight, decorate texts, offer autocomplete and much more.
A small customizable textarea for React to colorize, highlight, decorate texts, offer autocomplete and much more.
[!NOTE] You may also like https://github.com/inokawa/edix
https://inokawa.github.io/rich-textarea/
Sometimes we need customized text editor in web. However creating it with raw contenteditable is so hard to do properly and editor frameworks are usually too heavy... Maybe you really need is just a textarea with highlighting and some hovered menus, but native textarea and many of textarea libraries are far from it because of the limited customizability. This library is aiming to solve the problem.
npm install rich-textarea
If you use ESM and webpack 5, use react >= 18 to avoid Can't resolve react/jsx-runtime
error.
import { useState } from "react";
import { RichTextarea } from "rich-textarea";
export const App = () => {
const [text, setText] = useState("Lorem ipsum");
return (
<RichTextarea
value={text}
style={{ width: "600px", height: "400px" }}
onChange={(e) => setText(e.target.value)}
>
{(v) => {
return v.split("").map((t, i) => (
<span key={i} style={{ color: i % 2 === 0 ? "red" : undefined }}>
{t}
</span>
));
}}
</RichTextarea>
);
};
import { RichTextarea } from "rich-textarea";
export const App = () => {
return (
<RichTextarea
defaultValue="Lorem ipsum"
style={{ width: "600px", height: "400px" }}
>
{(v) => {
return v.split("").map((t, i) => (
<span key={i} style={{ color: i % 2 === 0 ? "red" : undefined }}>
{t}
</span>
));
}}
</RichTextarea>
);
};
You can use helper for regex if you don't want to create your own render function.
import { useState } from "react";
import { RichTextarea, createRegexRenderer } from "rich-textarea";
const renderer = createRegexRenderer([
[/[A-Z][a-z]+/g, { borderRadius: "3px", backgroundColor: "#d0bfff" }],
]);
export const App = () => {
const [text, setText] = useState("Lorem ipsum");
return (
<RichTextarea
value={text}
style={{ width: "600px", height: "400px" }}
onChange={(e) => setText(e.target.value)}
>
{renderer}
</RichTextarea>
);
};
// _components/Textarea.tsx
"use client";
import { RichTextarea, RichTextareaProps } from "rich-textarea";
export const Textarea = (
props: Omit<RichTextareaProps, "children" | "ref">
) => {
return (
<RichTextarea {...props}>
{(v) => {
return v.split("").map((t, i) => (
<span key={i} style={{ color: i % 2 === 0 ? "red" : undefined }}>
{t}
</span>
));
}}
</RichTextarea>
);
};
// page.tsx in App Router of Next.js
import { Textarea } from "../_components/Textarea";
async function hello(formData: FormData) {
"use server";
console.log(formData.get("hello"));
}
export default () => {
return (
<div>
<div>this is server!</div>
<form action={hello}>
<Textarea defaultValue="Lorem ipsum" name="hello" />
<button type="submit">submit</button>
</form>
</div>
);
};
import type { ActionFunction } from "@remix-run/node";
import { Form } from "@remix-run/react";
import { RichTextarea, RichTextareaProps } from "rich-textarea";
const Textarea = (props: Omit<RichTextareaProps, "children" | "ref">) => {
return (
<RichTextarea {...props}>
{(v) => {
return v.split("").map((t, i) => (
<span key={i} style={{ color: i % 2 === 0 ? "red" : undefined }}>
{t}
</span>
));
}}
</RichTextarea>
);
};
export const action: ActionFunction = async ({ request }) => {
console.log((await request.formData()).get("hello"));
};
export default () => {
return (
<Form method="post">
<Textarea defaultValue="Lorem ipsum" name="hello" />
<button type="submit">submit</button>
</Form>
);
};
All contributions are welcome. If you find a problem, feel free to create an issue or a PR.
npm install
.FAQs
A small customizable textarea for React to colorize, highlight, decorate texts, offer autocomplete and much more.
We found that rich-textarea demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
A malicious npm package targets Solana developers, rerouting funds in 2% of transactions to a hardcoded address.
Security News
Research
Socket researchers have discovered malicious npm packages targeting crypto developers, stealing credentials and wallet data using spyware delivered through typosquats of popular cryptographic libraries.
Security News
Socket's package search now displays weekly downloads for npm packages, helping developers quickly assess popularity and make more informed decisions.