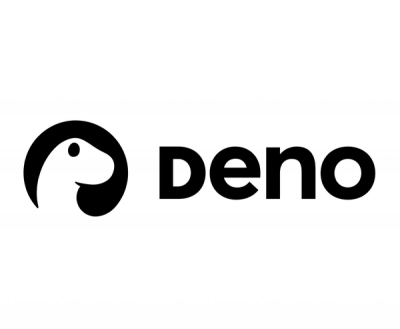
Security News
Deno 2.2 Improves Dependency Management and Expands Node.js Compatibility
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
rmmbr
gives you a decorator you can place around async functions to have them be cached, locally or in the cloud.
Usage:
npm i rmmbr
import { cloudCache, localCache } from "rmmbr";
const cacher = localCache({ id: "some-id" });
let nCalled = 0;
const f = (x: number) => {
nCalled++;
return Promise.resolve(x);
};
const fCached = cacher(f);
await fCached(3);
await fCached(3);
// nCalled is 1 here
The local cache stores data in a text file under a .rmmbr
directory.
There is also a memCache
, if you are feeling nostalgic 😉 and just want to store stuff in memory.
If you want to persist across devices, we offer a use cloud service, it is free to use up to a quota:
const cacher = cloudCache({
token: "service-token",
cacheId: "some name for the cache",
url: "https://uriva-rmmbr.deno.dev",
ttl: 60 * 60 * 24, // Values will expire after one day, omission of this field implies max.
});
If your data is sensitive, you can encrypt it by adding an encryptionKey
parameter:
const cacher = cloudCache({
token: "service-token",
cacheId: "some name for the cache",
url: "https://uriva-rmmbr.deno.dev",
encryptionKey: "eeeeeeeeeeeeeeeeeeeeeeeeeeeeeeee",
});
Note that this is implemented as e2e encryption.
At the moment this service is with no guarantees, but we are working on a production tier as well. Please contact us or post an issue if you want to try it out!
We also accept issues for feature requests 👩🔧
pip install rmmbr
The python api mimicks the js one, with exported decorators named mem_cache
, local_cache
and cloud_cache
.
FAQs
Easy caching.
The npm package rmmbr-cli receives a total of 0 weekly downloads. As such, rmmbr-cli popularity was classified as not popular.
We found that rmmbr-cli demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
Security News
React's CRA deprecation announcement sparked community criticism over framework recommendations, leading to quick updates acknowledging build tools like Vite as valid alternatives.
Security News
Ransomware payment rates hit an all-time low in 2024 as law enforcement crackdowns, stronger defenses, and shifting policies make attacks riskier and less profitable.