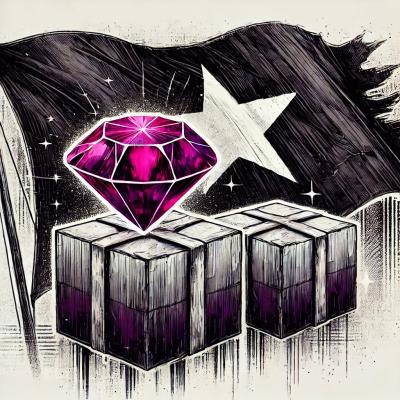
Research
Security News
Malicious Ruby Gems Exfiltrate Telegram Tokens and Messages Following Vietnam Ban
Malicious Ruby gems typosquat Fastlane plugins to steal Telegram bot tokens, messages, and files, exploiting demand after Vietnam’s Telegram ban.