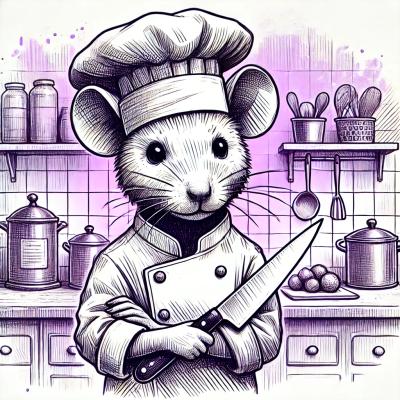
Research
Security News
Quasar RAT Disguised as an npm Package for Detecting Vulnerabilities in Ethereum Smart Contracts
Socket researchers uncover a malicious npm package posing as a tool for detecting vulnerabilities in Etherium smart contracts.
Utility method to run function either synchronously or asynchronously using the common `this.async()` style.
The run-async npm package allows you to easily run functions asynchronously, supporting both callback-style and promise-based workflows. It is particularly useful for wrapping functions that use callbacks into a promise-based interface, making it easier to work with in modern JavaScript applications that heavily utilize promises or async/await syntax.
Running asynchronous functions with callback
This feature allows you to run functions that use the traditional callback pattern asynchronously. The example demonstrates wrapping a setTimeout call, which is asynchronous, in runAsync to handle it with a callback.
const runAsync = require('run-async');
runAsync(function (callback) {
setTimeout(function () {
callback(null, 'result');
}, 100);
})(function (err, result) {
console.log(result); // 'result'
});
Using with Promises
This feature demonstrates how run-async can be used to convert a callback-based asynchronous function into a promise-based one. This is particularly useful for integrating older, callback-based APIs into newer codebases that use promises or async/await.
const runAsync = require('run-async');
const asyncFunction = runAsync(function (callback) {
setTimeout(function () {
callback(null, 'result');
}, 100);
});
asyncFunction().then(result => {
console.log(result); // 'result'
});
Awaiting asynchronous functions
This feature showcases how run-async can be used with async/await syntax for an even cleaner and more intuitive asynchronous code. It wraps a callback-based asynchronous function and allows it to be awaited.
const runAsync = require('run-async');
(async () => {
const asyncFunction = runAsync(function (callback) {
setTimeout(function () {
callback(null, 'done');
}, 100);
});
const result = await asyncFunction();
console.log(result); // 'done'
})();
The 'async' package provides a powerful set of utilities for working with asynchronous JavaScript. While run-async focuses on converting callback-based functions into promises, 'async' offers a broader range of tools for managing and coordinating asynchronous operations, making it more versatile for complex workflows.
Bluebird is a fully-featured promise library with a focus on innovative features and performance. Unlike run-async, which is more about adapting callback-based functions to promises, Bluebird provides a wide range of promise-related utilities, including advanced features like cancellation, which are not directly related to run-async's core functionality.
Pify is a module that promisifies functions. It is similar to run-async in that it helps in converting callback-based asynchronous functions to promises, making it easier to work with async/await. However, pify is more focused and streamlined for this specific conversion process, without the additional layer of running the functions asynchronously as run-async does.
Utility method to run a function either synchronously or asynchronously using a series of common patterns. This is useful for library author accepting sync or async functions as parameter. runAsync
will always run them as an async method, and normalize the multiple signature.
npm install --save run-async
Here's a simple example print the function results and three options a user can provide a function.
var runAsync = require('run-async');
var printAfter = function (func) {
var cb = function (err, returnValue) {
console.log(returnValue);
};
runAsync(func, cb)(/* arguments for func */);
};
this.async
printAfter(function () {
var done = this.async();
setTimeout(function () {
done(null, 'done running with callback');
}, 10);
});
printAfter(function () {
return new Promise(function (resolve, reject) {
resolve('done running with promises');
});
});
printAfter(function () {
return 'done running sync function';
});
var runAsync = require('run-async');
runAsync(function() {
var callback = this.customAsync();
callback(null, a + b);
}, 'customAsync')(1, 2)
var runAsync = require('run-async');
runAsync(function() {
assert(this.isBound);
var callback = this.async();
callback(null, a + b);
}).call({ isBound: true }, 1, 2)
runAsync.cb
supports all the function types that runAsync
does and additionally a traditional callback as the last argument signature:
var runAsync = require('run-async');
// IMPORTANT: The wrapped function must have a fixed number of parameters.
runAsync.cb(function(a, b, cb) {
cb(null, a + b);
}, function(err, result) {
console.log(result)
})(1, 2)
If your version of node support Promises natively (node >= 0.12), runAsync
will return a promise. Example: runAsync(func)(arg1, arg2).then(cb)
Copyright (c) 2014 Simon Boudrias (twitter: @vaxilart)
Licensed under the MIT license.
FAQs
Utility method to run function either synchronously or asynchronously using the common `this.async()` style.
The npm package run-async receives a total of 18,108,510 weekly downloads. As such, run-async popularity was classified as popular.
We found that run-async demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
Socket researchers uncover a malicious npm package posing as a tool for detecting vulnerabilities in Etherium smart contracts.
Security News
Research
A supply chain attack on Rspack's npm packages injected cryptomining malware, potentially impacting thousands of developers.
Research
Security News
Socket researchers discovered a malware campaign on npm delivering the Skuld infostealer via typosquatted packages, exposing sensitive data.