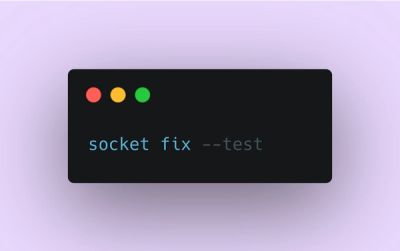
Product
Introducing Socket Fix for Safe, Automated Dependency Upgrades
Automatically fix and test dependency updates with socket fix—a new CLI tool that turns CVE alerts into safe, automated upgrades.
A simple Node.js package for generating CRUD operations using Mongoose.
A CRUD generator for Node.js applications.
You can install the package using npm:
npm install saksh-crud
To use the CRUD generator, run the script and follow the prompts:
node saksh-crud.js
You can configure the generator by creating a saksh-crud.config.json
file in the root directory. Here is an example configuration:
{
"defaultOutputDir": "./output",
"includeAuth": true,
"customTemplateDir": "./templates"
}
Here’s how to use the generator:
Run the script:
node saksh-crud.js
Follow the prompts to enter the model file path, choose whether to include authentication, and specify the output directory and custom template directory.
The generated routes file will be saved in the specified output directory.
You can customize the CRUD generator to fit your specific needs:
saksh-crud.config.json
file.{{modelName}}
, {{modelFile}}
, and {{modelNameLower}}
, which will be replaced with actual values during generation.saksh-crud.config.json
file to set default values for the output directory, authentication inclusion, and custom template directory.Example configuration:
{
"defaultOutputDir": "./customOutput",
"includeAuth": false,
"customTemplateDir": "./myTemplates"
}
The CRUD generator emits several events during its execution. You can listen to these events to perform custom actions:
beforeGenerate
Emitted before the routes file is generated.
Example:
generator.on('beforeGenerate', (modelName) => {
console.log(`Generating routes for model: ${modelName}`);
});
afterGenerate
Emitted after the routes file has been generated.
Example:
generator.on('afterGenerate', (outputPath) => {
console.log(`Routes file generated at: ${outputPath}`);
});
error
Emitted when an error occurs during the generation process.
Example:
generator.on('error', (err) => {
console.error('An error occurred:', err);
});
The generated CRUD routes also emit events that you can listen to:
beforeCreate
Emitted before a new record is created.
Example:
router.post('/', async (req, res, next) => {
try {
router.emit('beforeCreate', req.body);
const newRecord = await Model.create(req.body);
router.emit('afterCreate', newRecord);
res.status(201).json(newRecord);
} catch (err) {
router.emit('error', err);
next(err);
}
});
afterCreate
Emitted after a new record has been created.
Example:
router.post('/', async (req, res, next) => {
try {
const newRecord = await Model.create(req.body);
router.emit('afterCreate', newRecord);
res.status(201).json(newRecord);
} catch (err) {
router.emit('error', err);
next(err);
}
});
beforeUpdate
Emitted before an existing record is updated.
Example:
router.put('/:id', async (req, res, next) => {
try {
router.emit('beforeUpdate', req.params.id, req.body);
const updatedRecord = await Model.findByIdAndUpdate(req.params.id, req.body, { new: true });
router.emit('afterUpdate', updatedRecord);
res.status(200).json(updatedRecord);
} catch (err) {
router.emit('error', err);
next(err);
}
});
afterUpdate
Emitted after an existing record has been updated.
Example:
router.put('/:id', async (req, res, next) => {
try {
const updatedRecord = await Model.findByIdAndUpdate(req.params.id, req.body, { new: true });
router.emit('afterUpdate', updatedRecord);
res.status(200).json(updatedRecord);
} catch (err) {
router.emit('error', err);
next(err);
}
});
beforeDelete
Emitted before a record is deleted.
Example:
router.delete('/:id', async (req, res, next) => {
try {
router.emit('beforeDelete', req.params.id);
await Model.findByIdAndDelete(req.params.id);
router.emit('afterDelete', req.params.id);
res.status(204).end();
} catch (err) {
router.emit('error', err);
next(err);
}
});
afterDelete
Emitted after a record has been deleted.
Example:
router.delete('/:id', async (req, res, next) => {
try {
await Model.findByIdAndDelete(req.params.id);
router.emit('afterDelete', req.params.id);
res.status(204).end();
} catch (err) {
router.emit('error', err);
next(err);
}
});
Contributions are welcome! Please open an issue or submit a pull request.
This project is licensed under the ISC License.
FAQs
A simple Node.js package for generating CRUD operations using Mongoose.
The npm package saksh-crud receives a total of 0 weekly downloads. As such, saksh-crud popularity was classified as not popular.
We found that saksh-crud demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Product
Automatically fix and test dependency updates with socket fix—a new CLI tool that turns CVE alerts into safe, automated upgrades.
Security News
CISA denies CVE funding issues amid backlash over a new CVE foundation formed by board members, raising concerns about transparency and program governance.
Product
We’re excited to announce a powerful new capability in Socket: historical data and enhanced analytics.