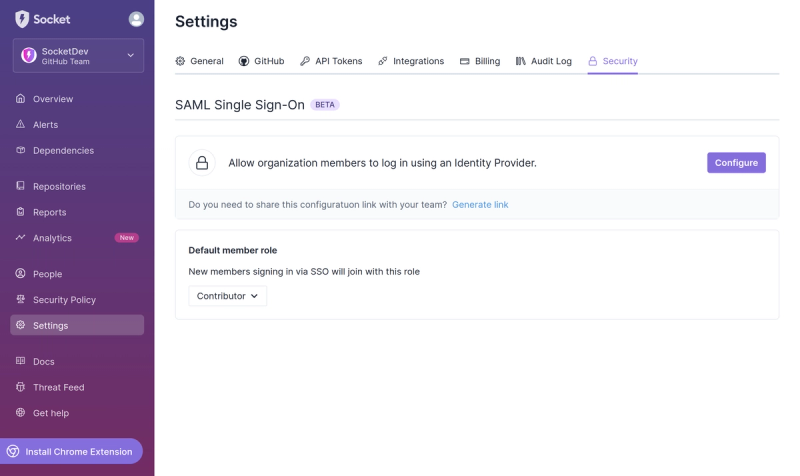
Product
Introducing SSO
Streamline your login process and enhance security by enabling Single Sign-On (SSO) on the Socket platform, now available for all customers on the Enterprise plan, supporting 20+ identity providers.
scanner-box
Advanced tools
Readme
npm install scanner-box
The following code uses expo-barcode-scanner for the barcode scanner
import * as React from "react";
import { StyleSheet, View, Text, Button, Dimensions } from "react-native";
import { useState, useEffect } from "react";
import QrCodeScanner from "./QrCodeScanner";
import { BarCodeScanner, BarCodeScannerResult } from "expo-barcode-scanner";
export default function App() {
const [boundOrigin, setBoundOrigin] = useState({ x: 0, y: 0 });
const [boundSize, setBoundSize] = useState({ x: 0, y: 0 });
const [hasPermission, setHasPermission] = useState(false);
const [scanned, setScanned] = useState(false);
useEffect(() => {
askForCameraPermission();
});
const askForCameraPermission = () => {
(async () => {
const { status } = await BarCodeScanner.requestPermissionsAsync();
setHasPermission(status == "granted");
})();
};
const handleBarCodeScanned = (scanningResult: BarCodeScannerResult) => {
if (!scanned) {
const { type, data, bounds } = scanningResult;
if (bounds) {
setBoundOrigin({ x: bounds.origin.x, y: bounds.origin.y });
setBoundSize({ width: bounds.size.width, height: bounds.size.height });
}
setScanned(true);
setTimeout(() => {
setScanned(false);
}, 1000);
}
};
if (hasPermission === null) {
return (
<View style={styles.container}>
<Text>Requesting for camera permission</Text>
</View>
);
}
if (hasPermission === false) {
return (
<View style={styles.container}>
<Text style={{ margin: 10 }}>No access to camera</Text>
<Button onPress={() => askForCameraPermission()} title="Test">
Allow Camera
</Button>
</View>
);
}
return (
<View style={styles.container}>
<BarCodeScanner
barCodeTypes={[BarCodeScanner.Constants.BarCodeType.qr]}
onBarCodeScanned={scanned ? undefined : handleBarCodeScanned}
style={[StyleSheet.absoluteFill, styles.scan]}
></BarCodeScanner>
<QrCodeScanner
cornerLength={20}
color="blue"
borderSize={10}
radius={30}
shape="corners"
origin={boundOrigin}
size={boundSize}
borderOffSet={5}
/>
</View>
);
}
const styles = StyleSheet.create({
container: {
flex: 1,
},
});
The following scanner-box component usage renders this bounding box with blue corners
<QrCodeScanner
cornerLength={30}
color="blue"
borderSize={5}
radius={30}
shape="corners"
origin={boundOrigin}
size={boundSize}
borderOffSet={10}
/>
The The following scanner-box component usage renders this red bounding box
<QrCodeScanner
color="red"
borderSize={5}
radius={10}
shape="square"
origin={boundOrigin}
size={boundSize}
/>
cornerLength only needed to be defined if user is going for a 'corners' bounding box, this is because cornerLength is use to set the legth and width of the corners and is not needed if the bounding box is a 'square'
borderOffset is used so the borders rendered does not land directly on top of the qr code.
FAQs
renders a bounding box around the qr code
The npm package scanner-box receives a total of 6 weekly downloads. As such, scanner-box popularity was classified as not popular.
We found that scanner-box demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Product
Streamline your login process and enhance security by enabling Single Sign-On (SSO) on the Socket platform, now available for all customers on the Enterprise plan, supporting 20+ identity providers.
Security News
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
Security News
As cyber threats become more autonomous, AI-powered defenses are crucial for businesses to stay ahead of attackers who can exploit software vulnerabilities at scale.