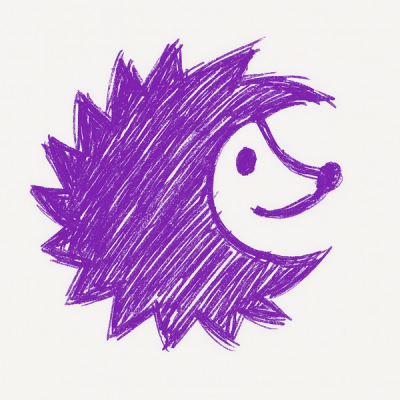
Security News
Browserslist-rs Gets Major Refactor, Cutting Binary Size by Over 1MB
Browserslist-rs now uses static data to reduce binary size by over 1MB, improving memory use and performance for Rust-based frontend tools.
Small JS utility for defining classes, performing inheritance and implementing interfaces.
Small JS utility for defining classes, performing inheritance and implementing interfaces. It is easy to use and supported IE8 and all modern browsers.
npm install sclass.js --save
bower install sclass.js --save
<script type="text/javascript" src="path_to_sclass/index.min.js"></script>
var $Class = require("sclass.js").$Class;
var MyClass = $Class(); // creating class
MyClass.prototype.$constructor = function(id) {
// this function is applied in its context during performing statement: new MyClass()
this.id = id;
this.text = "Good evening ... infidels!";
};
MyClass.prototype.getText = function() {
return this.text;
};
MyClass.prototype.getId = function() {
return this.id;
};
var instance = new MyClass(1); // creating instance of class
console.log(instance.getText()); // "Good evening ... infidels!"
console.log(instance.getId()); // 1
var DeathStar = $Class({
singleton : true
});
DeathStar.prototype.$constructor = function(id) {
this.weapons = [];
};
DeathStar.prototype.getWeapons = function() {
return this.weapons;
};
console.log(DeathStar.getInstance().getWeapons()); // []
// you can not call singleton with new, but always with getInstance method
// this throws an error
var dStar = new DeathStar();
var Knight = $Class();
Knight.prototype.$constructor = function() {
this.weapon = "saber";
};
Knight.prototype.getWeapon = function() {
return this.weapon;
};
var JediKnight = $Class({
extending: Knight // you can see we give reference to Knight
});
JediKnight.prototype.$constructor = function() {
this.weapon = "lightsaber";
};
var jKnight = new JediKnight();
console.log(jKnight instanceof JediKnight); // true
console.log(jKnight instanceof Knight); // true
console.log(jKnight.getWeapon()); // shows "lightsaber"
var Knight = $Class();
Knight.prototype.$constructor = function(name) {
this.name = name || "noname";
this.weapon = "saber";
};
Knight.prototype.getWeapon = function() {
return this.weapon;
};
Knight.prototype.setWeapon = function(name) {
this.weapon = name;
};
var JediKnight = $Class({
extending: Knight // you can see we give reference to Knight
});
JediKnight.prototype.$constructor = function(name, skill) {
this.$super(name); // just simply call $super to apply parent $constructor
this.skill = skill || 0;
this.weapon = "";
this.weaponSkill = 0;
};
JediKnight.prototype.setWeapon = function(name, skill) {
this.$super(name); // just simply call $super to apply parent setWeapon
this.weaponSkill = skill;
};
JediKnight.prototype.getSkill = function() {
return this.skill;
};
JediKnight.prototype.getWeaponSkill = function() {
return this.weaponSkill;
};
var jKnight = new JediKnight("Obi-Wan Kenobi", 5);
jKnight.setWeapon("lightsaber", 10);
console.log(jKnight.getSkill()); // 5
console.log(jKnight.getWeapon()); // "lightsaber"
console.log(jKnight.getWeaponSkill()); // 10
var IFaceWeapons = {
setWeapon: function(name) {
this.name = name;
},
getWeapon: function() {
return this.name;
}
};
var IFaceSkills = {
setSkill: function(skill) {
this.skill = skill;
},
getSkill: function() {
return this.skill;
}
};
var Knight = $Class({
implementing: IFaceWeapons
});
Knight.prototype.$constructor = function(name) {
this.name = name || "noname";
};
var JediKnight = $Class({
extending: Knight,
implementing: IFaceSkills
}); // we use parent $constructor, we can not define it again
var jKnight = new JediKnight("Obi-Wan Kenobi");
jKnight.setWeapon("lightsaber");
jKnight.setSkill(10);
console.log(jKnight.getWeapon()); // "lightsaber"
console.log(jKnight.getSkill()); // 10
var Sith = $Class({
extending: Knight,
implementing: [IFaceWeapons, IFaceSkills] // first IFaceWeapons is implemented and then IFaceSkills
});
FAQs
Small JS utility for defining classes, performing inheritance and implementing interfaces.
We found that sclass.js demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Browserslist-rs now uses static data to reduce binary size by over 1MB, improving memory use and performance for Rust-based frontend tools.
Research
Security News
Eight new malicious Firefox extensions impersonate games, steal OAuth tokens, hijack sessions, and exploit browser permissions to spy on users.
Security News
The official Go SDK for the Model Context Protocol is in development, with a stable, production-ready release expected by August 2025.