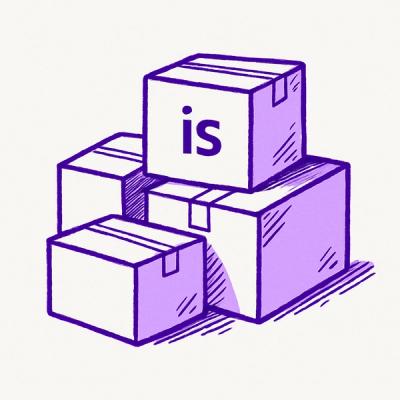
Security News
npm ‘is’ Package Hijacked in Expanding Supply Chain Attack
The ongoing npm phishing campaign escalates as attackers hijack the popular 'is' package, embedding malware in multiple versions.
#Scribble ###Simple Last.fm scrobbler for node.js
#####Scribble down the song
###PreReqs
Get a last.fm API account and save the api key, api secret, and your username and password.
###Install npm install scribble
###Use it
var scribble = require('scribble');
// Build your scrobbler using your API keys and user info
var Scrobbler = new scribble('your_api_key','your_api_secret','your_lastfm_username','your_lastfm_password');
// Make a song object in your app
var song = {
artist: 'Slayer',
track: 'Disciple',
album: 'God Hates Us All' // Only needed for album info call
};
/*
POST methods
All return the XML response from the POST requesta
*/
// Now Playing
Scrobbler.NowPlaying(song, function(post_return_data) {});
// Scrobble
Scrobbler.Scrobble(song, function(post_return_data) {});
// Love
Scrobbler.Love(song, function(post_return_data) {});
/*
GET methods
All return the parsed JSON response from Last.fm
*/
// Get Album
Scrobbler.GetAlbum(song, function(ret) {});
// Get Artist Info
Scrobbler.GetArtistInfo(song.artist, function(ret) {});
// Get Similar Artists
Scrobbler.GetSimilarArtists(song.artist, function(ret) {}, optional_return_amount_defaults_to_50);
// Get Artist Events
Scrobbler.GetArtistEvents(song.artist, function(ret) {}, optional_return_amount_defaults_to_50);
// Get Artist Top Albums
Scrobbler.GetTopAlbums(song.artist, function(ret) {}, optional_return_amount_defaults_to_50);
// Get Artist Top Tracks
Scrobbler.GetArtistTopTracks(song.artist, function(ret) {}, optional_return_amount_defaults_to_50);
// Get Similar Songs
Scrobbler.GetSimilarSongs(song, function(ret) {}, optional_return_amount_defaults_to_50);
// Get Track Info
Scrobbler.GetTrackInfo(song, function(ret) {});
// Get Album Info
Scrobbler.GetAlbumInfo(song, function(ret) {});
###Tests
npm install
make test
or
npm test
FAQs
quick and sloppy Last.fm scrobbler and API library
The npm package scribble receives a total of 2 weekly downloads. As such, scribble popularity was classified as not popular.
We found that scribble demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
The ongoing npm phishing campaign escalates as attackers hijack the popular 'is' package, embedding malware in multiple versions.
Security News
A critical flaw in the popular npm form-data package could allow HTTP parameter pollution, affecting millions of projects until patched versions are adopted.
Security News
Bun 1.2.19 introduces isolated installs for smoother monorepo workflows, along with performance boosts, new tooling, and key compatibility fixes.