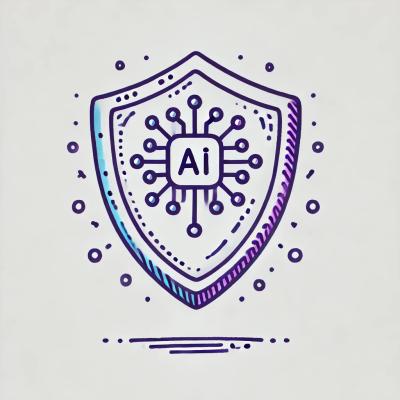
Security News
38% of CISOs Fear They’re Not Moving Fast Enough on AI
CISOs are racing to adopt AI for cybersecurity, but hurdles in budgets and governance may leave some falling behind in the fight against cyber threats.
The scuid npm package is designed to generate short, collision-resistant, URL-friendly unique identifiers. These identifiers are suitable for use where a unique string is necessary, such as database keys, component ids, or any other unique element identifier in a distributed system.
Generating a unique identifier
This feature allows the generation of a unique identifier string that is URL-friendly and highly unlikely to collide with other generated identifiers.
"const scuid = require('scuid');
const id = scuid();
console.log(id); // Outputs a unique identifier string"
Generating a unique identifier with a custom prefix
This feature allows the generation of a unique identifier with a custom prefix, which can be useful for namespacing or categorizing identifiers.
"const scuid = require('scuid');
const idWithPrefix = scuid('usr_');
console.log(idWithPrefix); // Outputs a unique identifier string with 'usr_' prefix"
The uuid package generates RFC-compliant UUIDs. It offers different versions of UUIDs, such as v1 (timestamp-based), v4 (random), and others. Unlike scuid, which generates shorter, URL-friendly IDs, UUIDs are longer and standardized.
NanoID is a tiny, secure, URL-friendly, unique string ID generator. It is similar to scuid in that it produces short, unique identifiers, but it also allows for custom alphabet and size, providing more flexibility in the generation process.
ShortId is another package for generating short non-sequential url-friendly unique ids. However, it has been deprecated in favor of NanoID due to the potential for local collisions under heavy use and other issues.
Collision-resistant IDs optimized for horizontal scaling and performance.
A slim, alternative, and compatible implementation of cuid for node, also featuring a wide range of options, as well as custom random number generator support. It can serve as a drop-in replacement, and is also faster than cuid.
$ npm install --save scuid
var scuid = require( 'scuid' )
Generate an ID
var id = scuid()
> 'ciux3hs0x0000io10cusdm8r2'
Generate a slug
var slug = scuid.slug()
> '6x1i0r0'
Get the process' fingerprint
var fingerprint = scuid.fingerprint()
> 'io10'
Use a custom (P)RNG
// Create a random number generator;
// It has to have a method called `random`
var generator = {
random: function() {
return 5 // chosen by fair dice roll
}
}
// Create a custom scuid instance
var scuid = require( 'scuid' ).create({
rng: generator
})
Use other custom options
Note that fiddeling with these might make your IDs incompatible with cuid's guarantees.
var scuid = require( 'scuid' ).create({
prefix: 'c', // the ID's prefix
base: 36, // radix used in .toString() calls (2-36)
blockSize: 4, // block size to pad and trim to
fill: '0', // block padding character
pid: process.pid, // process ID
fingerprint: scuid.createFingerprint( [pid], [hostname] ), // Machine fingerprint
rng: Math, // Random number generator
})
Just like cuid
, collision resistance for both – slugs and IDs – is tested
over 1 million and 2 million iterations respectively.
To run the tests, run:
$ npm test
$ npm run benchmark
scuid
id ............................................. 573,618 op/s
slug ........................................... 673,732 op/s
fingerprint .................................... 131,156,394 op/s
cuid
id ............................................. 445,260 op/s
slug ........................................... 531,770 op/s
fingerprint .................................... 125,159,685 op/s
FAQs
Collision-resistant IDs optimized for horizontal scaling and performance
The npm package scuid receives a total of 507,856 weekly downloads. As such, scuid popularity was classified as popular.
We found that scuid demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
CISOs are racing to adopt AI for cybersecurity, but hurdles in budgets and governance may leave some falling behind in the fight against cyber threats.
Research
Security News
Socket researchers uncovered a backdoored typosquat of BoltDB in the Go ecosystem, exploiting Go Module Proxy caching to persist undetected for years.
Security News
Company News
Socket is joining TC54 to help develop standards for software supply chain security, contributing to the evolution of SBOMs, CycloneDX, and Package URL specifications.