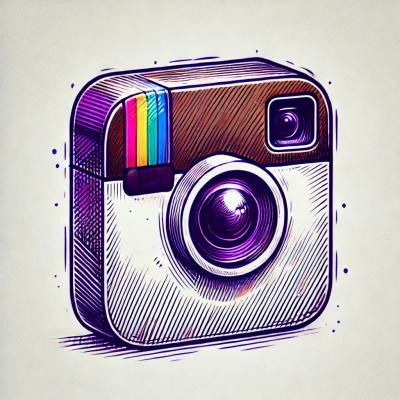
Research
PyPI Package Disguised as Instagram Growth Tool Harvests User Credentials
A deceptive PyPI package posing as an Instagram growth tool collects user credentials and sends them to third-party bot services.
Secure localStorage/sessionStorage data with high level of encryption and data compression
Secure localStorage/sessionStorage data with high level of encryption and data compression.
AES
, DES
, Rabbit
and RC4
. (defaults to Base64
encoding).localStorage
to save extra bytes (defaults to true
).localStorage
API, providing other basic utilities.localStorage
and secure-ls
will always remember it's creation.Via NPM
npm install secure-ls
Via yarn
yarn add secure-ls
Encryption / Decryption using The Cipher Algorithms
It requires secret-key for encrypting and decrypting data securely. If custom secret-key is provided as mentioned below in APIs, then the library will pick that otherwise it will generate yet another very secure
unique password key using PBKDF2, which will be further used for future API requests.
PBKDF2
is a password-based key derivation function. In many applications of cryptography, user security is ultimately dependent on a password, and because a password usually can't be used directly as a cryptographic key, some processing is required.
A salt provides a large set of keys for any given password, and an iteration count increases the cost of producing keys from a password, thereby also increasing the difficulty of attack.
Eg: 55e8f5585789191d350329b9ebcf2b11
and db51d35aad96610683d5a40a70b20c39
.
For the generation of such strings, secretPhrase
is being used and can be found in code easily but that won't make it unsecure, PBKDF2
's layer on top of that will handle security.
Compresion / Decompression using lz-string
default
settings i.e. Base64
Encoding and Data Compressionconst ls = new SecureLS();
ls.set('key1', { data: 'test' }); // set key1
ls.get('key1'); // print data
// {data: 'test'}
AES
Encryption and Data Compressionconst ls = new SecureLS({ encodingType: 'aes' });
ls.set('key1', { data: 'test' }); // set key1
ls.get('key1'); // print data
// {data: 'test'}
ls.set('key2', [1, 2, 3]); // set another key
ls.getAllKeys(); // get all keys
// ["key1", "key2"]
ls.removeAll(); // remove all keys
RC4
Encryption but no Data Compressionconst ls = new SecureLS({ encodingType: 'rc4', isCompression: false });
ls.set('key1', { data: 'test' }); // set key1
ls.get('key1'); // print data
// {data: 'test'}
ls.set('key2', [1, 2, 3]); // set another key
ls.getAllKeys(); // get all keys
// ["key1", "key2"]
ls.removeAll(); // remove all keys
DES
Encryption, no Data Compression and custom secret keyconst ls = new SecureLS({ encodingType: 'des', isCompression: false, encryptionSecret: 'my-secret-key' });
ls.set('key1', { data: 'test' }); // set key1
ls.get('key1'); // print data
// {data: 'test'}
ls.set('key2', [1, 2, 3]); // set another key
ls.getAllKeys(); // get all keys
// ["key1", "key2"]
ls.removeAll(); // remove all keys
const ls = new SecureLS();
Contructor
accepts a configurable Object
with all three keys being optional.
Config Keys | default | accepts |
---|---|---|
encodingType | Base64 | base64 /aes /des /rabbit /rc4 /'' |
isCompression | true | true /false |
encryptionSecret | PBKDF2 value | String |
encryptionNamespace | null | String |
Note: encryptionSecret
will only be used for the Encryption and Decryption of data
with AES
, DES
, RC4
, RABBIT
, and the library will discard it if no encoding / Base64
encoding method is choosen.
encryptionNamespace
is used to make multiple instances with different encryptionSecret
and/or different encryptionSecret
possible.
const ls1 = new SecureLS({ encodingType: 'des', encryptionSecret: 'my-secret-key-1' });
const ls2 = new SecureLS({ encodingType: 'aes', encryptionSecret: 'my-secret-key-2' });
Examples:
Base64 Encoding
and Data compression
const ls = new SecureLS();
// or
const ls = new SecureLS({});
Normal
way of storing dataconst ls = new SecureLS({ encodingType: '', isCompression: false });
Base64
encoding but no
data compressionconst ls = new SecureLS({ isCompression: false });
AES
encryption and data compression
const ls = new SecureLS({ encodingType: 'aes' });
RC4
encryption and no
data compressionconst ls = new SecureLS({ encodingType: 'rc4', isCompression: false });
RABBIT
encryption, no
data compression and custom
encryptionSecretconst ls = new SecureLS({ encodingType: 'rc4', isCompression: false, encryptionSecret: 's3cr3tPa$$w0rd@123' });
set
Saves data
in specifed key
in localStorage. If the key is not provided, the library will warn. Following types of JavaScript objects are supported:
Parameter | Description |
---|---|
key | key to store data in |
data | data to be stored |
ls.set('key-name', { test: 'secure-ls' });
get
Gets data
back from specified key
from the localStorage library. If the key is not provided, the library will warn.
Parameter | Description |
---|---|
key | key in which data is stored |
ls.get('key-name');
remove
Removes the value of a key from the localStorage. If the meta key
, which stores the list of keys, is tried to be removed even if there are other keys which were created by secure-ls
library, the library will warn for the action.
Parameter | Description |
---|---|
key | remove key in which data is stored |
ls.remove('key-name');
removeAll
Removes all the keys that were created by the secure-ls
library, even the meta key
.
ls.removeAll();
clear
Removes all the keys ever created for that particular domain. Remember localStorage works differently for http
and https
protocol;
ls.clear();
getAllKeys
Gets the list of keys that were created using the secure-ls
library. Helpful when data needs to be retrieved for all the keys or when keys name are not known(dynamically created keys).
getAllKeys()
ls.getAllKeys();
npm run build
- produces production version of the library under the dist
foldernpm run build-dev
- produces development version of the library and runs a watchernpm run test
- well ... it runs the tests :)npm install
and npm run dev
.<fix-typo>
and do your work.npm run build
to build dist files and npm run test
to ensure all test cases are passing.src
compilation & bundling and dist
generation.ES6 source files
|
|
webpack
|
+--- babel, eslint
|
ready to use
library
in umd format
Many thanks to:
@brix for the awesome crypto-js library for encrypting and decrypting data securely.
@pieroxy for the lz-string js library for data compression / decompression.
The MIT license (MIT)
Copyright (c) 2015-2024 Varun Malhotra
Permission is hereby granted, free of charge, to any person obtaining a copy of this software and associated documentation files (the "Software"), to deal in the Software without restriction, including without limitation the rights to use, copy, modify, merge, publish, distribute, sublicense, and/or sell copies of the Software, and to permit persons to whom the Software is furnished to do so, subject to the following conditions:
The above copyright notice and this permission notice shall be included in all copies or substantial portions of the Software.
THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE.
FAQs
Secure localStorage/sessionStorage data with high level of encryption and data compression
The npm package secure-ls receives a total of 21,211 weekly downloads. As such, secure-ls popularity was classified as popular.
We found that secure-ls demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
A deceptive PyPI package posing as an Instagram growth tool collects user credentials and sends them to third-party bot services.
Product
Socket now supports pylock.toml, enabling secure, reproducible Python builds with advanced scanning and full alignment with PEP 751's new standard.
Security News
Research
Socket uncovered two npm packages that register hidden HTTP endpoints to delete all files on command.