Comparing version
module.exports = { | ||
Shoukaku: require('./src/Shoukaku.js'), | ||
Constants: require('./src/ShoukakuConstants.js'), | ||
Constants: require('./src/constants/ShoukakuConstants.js'), | ||
version: require('./package.json').version | ||
}; | ||
}; |
{ | ||
"name": "shoukaku", | ||
"version": "0.1.1", | ||
"version": "0.2.0", | ||
"description": "A lavalink client for Discord.js v12 only", | ||
"main": "index.js", | ||
"types": "index.d.ts", | ||
"keywords": [ | ||
@@ -20,3 +21,3 @@ "bot", | ||
"repository": { | ||
"type": "git", | ||
"type": "git", | ||
"url": "https://github.com/Deivu/Shoukaku" | ||
@@ -28,3 +29,7 @@ }, | ||
"ws": "^7.0.0" | ||
}, | ||
"devDependencies": { | ||
"discord.js": "github:discordjs/discord.js", | ||
"typescript": "^3.5.2" | ||
} | ||
} |
118
README.md
# Shoukaku | ||
<p align="center"> | ||
<img src="https://vignette.wikia.nocookie.net/kancolle/images/9/97/Shoukaku_Christmas_Full.png/revision/latest/"> | ||
<img src="https://vignette.wikia.nocookie.net/kancolle/images/b/b3/Shoukaku_Christmas_Full_Damaged.png/revision/latest/"> | ||
</p> | ||
@@ -10,4 +10,19 @@ | ||
Currently being used by [Kashima](https://discordbots.org/bot/kashima-is-a-good-girl) | ||
✅ Currently being used by: | ||
[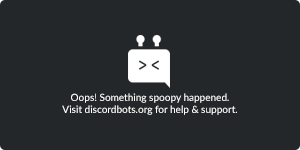](https://discordbots.org/bot/424137718961012737) | ||
### Why Shoukaku? | ||
✅ Straightforward | ||
✅ Scalable | ||
✅ Reliable | ||
✅ Maintained | ||
✅ Very Cute and Charming Shipgirl ❤ | ||
✅ And will make your library weeb 😂 | ||
### Documentation | ||
@@ -26,2 +41,37 @@ https://deivu.github.io/Shoukaku/?api | ||
### 0.1.1 -> 0.2.0 Migration | ||
> ShoukakuLink is now a property of ShoukakuPlayer, meaning all link related getters are changed to player getters. | ||
> You can access ShoukakuLink via .voiceConnection property of ShoukakuPlayer | ||
> ShoukakuPlayer events are "GREATLY CHANGED". Those marked as optional can be left out. | ||
The events is as follows | ||
- end | ||
- closed | ||
- error | ||
- nodeDisconnect | ||
- trackException "optional" | ||
- resumed "optional" | ||
- playerUpdate "optional" | ||
> Shoukaku class have renamed methods and properties | ||
- getLink() -> getPlayer() | ||
- .links -> .players | ||
- .totalLinks -> .totalPlayers | ||
> ShoukakuSocket also have a renamed property | ||
- .links -> .players | ||
> You don't disconnect / clean the player on ShoukakuLink but on ShoukakuPlayer now making your code more clean and better | ||
```js | ||
// 0.1.1 and earlier versions | ||
ShoukakuLink.player.playTrack(); | ||
ShoukakuLink.disconnect(); | ||
// 0.2.0 | ||
ShoukakuPlayer.playTrack(); | ||
ShoukakuPlayer.disconnect(); | ||
``` | ||
You can see more of the changes on updated example below. | ||
### Support Server | ||
@@ -48,5 +98,8 @@ If you need help on using this, Join Here [ShipGirls Community](https://discordapp.com/invite/FVqbtGu) and `ask at #support`. | ||
### Discord.js actual implementation. | ||
[View Kongou's Source Code Here](https://github.com/Deivu/Kongou) | ||
### Starting a Lavalink Server. | ||
[View Lavalink README here](https://github.com/Frederikam/Lavalink/blob/master/README.md) | ||
### Discord.js actual implementation. | ||
[View Kongou's source code here](https://github.com/Deivu/Kongou) | ||
### More simple implementation w/o queue. | ||
@@ -73,7 +126,11 @@ ```js | ||
}); | ||
// Attach listeners, currently this are the only listeners available | ||
// on ERROR must be handled. | ||
// Listeners you can use for Shoukaku | ||
Carrier.on('ready', (name) => console.log(`Lavalink Node: ${name} is now connected`)); | ||
// Error must be handled | ||
Carrier.on('error', (name, error) => console.log(`Lavalink Node: ${name} emitted an error.`, error)); | ||
// Close emits when a lavalink node disconnects. | ||
Carrier.on('close', (name, code, reason) => console.log(`Lavalink Node: ${name} closed with code ${code}. Reason: ${reason || 'No reason'}`)); | ||
// Disconnected emits when a lavalink node disconnected and will not try to reconnect again. | ||
Carrier.on('disconnected', (name, reason) => console.log(`Lavalink Node: ${name} disconnected. Reason: ${reason || 'No reason'}`)); | ||
@@ -91,4 +148,4 @@ client.on('ready', () => { | ||
// Check if there is already a link on your guild. | ||
if (Carrier.getLink(msg.guild.id)) return; | ||
// Check if there is already a link on your guild. Since this is a no queue implementation. | ||
if (Carrier.getPlayer(msg.guild.id)) return; | ||
@@ -109,37 +166,20 @@ const args = msg.content.split(' '); | ||
// Joining to a voice channel that returns the LINK property that we need. | ||
const link = await node.joinVoiceChannel({ | ||
// Getting the ShoukakuPlayer where we can play tracks and disconnect once we dont need it anymore. | ||
const player = await node.joinVoiceChannel({ | ||
guildID: msg.guild.id, | ||
voiceChannelID: msg.member.voice.channelID | ||
}); | ||
// Example of handling the non optional events. | ||
const endFunction = (param) => { | ||
console.log(param); | ||
player.disconnect(); | ||
} | ||
player.on('end', endFunction); | ||
player.on('closed', endFunction); | ||
player.on('error', endFunction); | ||
player.on('nodeDisconnect', endFunction); | ||
// link.player is our player class for that link, thats what we can use to play music | ||
link.player.on('end', (reason) => { | ||
console.log(reason); | ||
// Disconnect the link and clean everyting up | ||
link.disconnect(); | ||
}); | ||
link.player.on('exception', console.error); | ||
link.player.on('stuck', (reason) => { | ||
console.warn(reason); | ||
// In stuck event, end will not fire automatically, either we just disconnect or play another song | ||
link.disconnect(); | ||
}); | ||
link.player.on('voiceClose', (reason) => { | ||
// Make sure you log the reason because it may be an error. | ||
console.log(reason); | ||
// There is no more reason for us to do anything so lets just clean up in voiceClose event | ||
link.disconnect(); | ||
}); | ||
link.player.on('nodeDisconnect', () => { | ||
// You still need to clean your link when player.on 'nodeDisconnect' fires. This means the node that governs this link disconnected. | ||
link.disconnect(); | ||
}) | ||
// Play the lavalink track we got | ||
await link.player.playTrack(data.track); | ||
await player.playTrack(data.track); | ||
await msg.channel.send("Now Playing: " + data.info.title); | ||
@@ -149,2 +189,2 @@ } | ||
client.login('token'); | ||
``` | ||
``` |
@@ -1,4 +0,4 @@ | ||
const { RawRouter, ReconnectRouter } = require('./ShoukakuRouter.js'); | ||
const constants = require('./ShoukakuConstants.js'); | ||
const ShoukakuSocket = require('./ShoukakuSocket.js'); | ||
const { RawRouter, ReconnectRouter } = require('./router/ShoukakuRouter.js'); | ||
const constants = require('./constants/ShoukakuConstants.js'); | ||
const ShoukakuSocket = require('./node/ShoukakuSocket.js'); | ||
const EventEmitter = require('events'); | ||
@@ -58,17 +58,19 @@ | ||
/** | ||
* Gets all the links governed by the Nodes / Sockets in this instance. | ||
* Gets all the Players governed by the Nodes / Sockets in this instance. | ||
* @type {external:Map} | ||
*/ | ||
get links() { | ||
const links = new Map(); | ||
for (const [key, val] of this.nodes) links.set(key, val); | ||
return links; | ||
get players() { | ||
const players = new Map(); | ||
for (const node of this.nodes.values()) { | ||
for (const [id, player] of node.players) players.set(id, player); | ||
} | ||
return players; | ||
} | ||
/** | ||
* Gets the number of total links that is currently active on all nodes in this instance. | ||
* Gets the number of total Players that is currently active on all nodes in this instance. | ||
* @type {number} | ||
*/ | ||
get totalLinks() { | ||
get totalPlayers() { | ||
let counter = 0; | ||
for (const node of this.nodes.values()) counter += node.links.size; | ||
for (const node of this.nodes.values()) counter += node.players.size; | ||
return counter; | ||
@@ -88,3 +90,3 @@ } | ||
* @param {string} name The name of the Lavalink Node that sent an error event or 'Shoukaku' if the error is from Shoukaku. | ||
* @param {error} error The error encountered. | ||
* @param {Error} error The error encountered. | ||
* @example | ||
@@ -116,3 +118,3 @@ * // <Shoukaku> is your own instance of Shoukaku | ||
/** | ||
* The starting point of Shoukaku, must be called in ready event in order for Shouaku to work. | ||
* The starting point of Shoukaku, must be called in ready event in order for Shoukaku to work. | ||
* @param {ShoukakuConstants#ShoukakuNodeOptions} nodes An array of lavalink nodes for Shoukaku to connect to. | ||
@@ -137,3 +139,3 @@ * @param {ShoukakuConstants#ShoukakuBuildOptions} options Options that is need by Shoukaku to build herself. | ||
* Function to register a Lavalink Node | ||
* @param {ShoukakuConstants#ShoukakuNodeOptions} nodeOptions An array of lavalink nodes for Shoukaku to connect to. | ||
* @param {ShoukakuConstants#ShoukakuNodeOptions} nodeOptions The Node Options to be used to connect to. | ||
* @returns {void} | ||
@@ -169,3 +171,3 @@ */ | ||
* Shortcut to get the Ideal Node or a manually specified Node from the current nodes that Shoukaku governs. | ||
* @param {boolean|string} [name=false] If blank, Shoukaku will automatically return the Ideal Node for you to connect to. If name is specifed, she will try to return the node you specified. | ||
* @param {boolean|string} [name] If blank, Shoukaku will automatically return the Ideal Node for you to connect to. If name is specifed, she will try to return the node you specified. | ||
* @returns {ShoukakuSocket} | ||
@@ -179,6 +181,6 @@ * @example | ||
* voiceChannelID: 'voice_channel_id' | ||
* }).then(link => link.player.playTrack(data.track)) | ||
* }).then(player => player.playTrack(data.track)) | ||
* }) | ||
*/ | ||
getNode(name = false) { | ||
getNode(name) { | ||
if (!this.nodes.size) | ||
@@ -194,14 +196,10 @@ throw new Error('No nodes available. What happened?'); | ||
/** | ||
* Shortcut to get the Link of a guild, if there is any. | ||
* Shortcut to get the Player of a guild, if there is any. | ||
* @param {string} guildID The guildID of the guild we are trying to get. | ||
* @returns {?ShoukakuLink} | ||
* @returns {?ShoukakuPlayer} | ||
*/ | ||
getLink(guildID) { | ||
getPlayer(guildID) { | ||
if (!guildID) return null; | ||
if (!this.nodes.size) return null; | ||
for (const node of this.nodes.values()) { | ||
const link = node.links.get(guildID); | ||
if (link) return link; | ||
} | ||
return null; | ||
return this.players.get(guildID); | ||
} | ||
@@ -208,0 +206,0 @@ |
Major refactor
Supply chain riskPackage has recently undergone a major refactor. It may be unstable or indicate significant internal changes. Use caution when updating to versions that include significant changes.
Found 1 instance in 1 package
59875
24.64%12
9.09%1375
25.11%184
28.67%2
Infinity%1
Infinity%