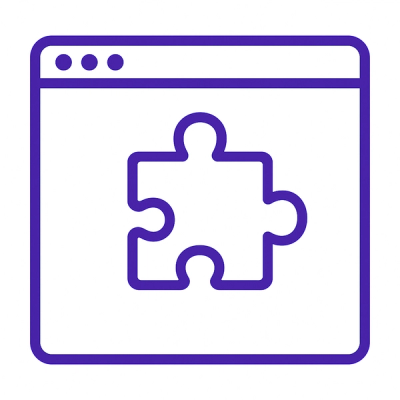
Research
Security News
The Growing Risk of Malicious Browser Extensions
Socket researchers uncover how browser extensions in trusted stores are used to hijack sessions, redirect traffic, and manipulate user behavior.
Reactive state and effect system for TypeScript, inspired by functional programming
Minimal reactive primitives for building fine-grained reactivity in JavaScript and TypeScript.
Composable, testable, and framework-agnostic.
state
, computed
, and event
primitivespipe(...)
for functional reactive compositionstateAsync
and mapAsync
bun add signux
# or
npm install signux
import { state } from "signux";
const counter = state(0);
console.log(counter()); // 0
counter
.on(
{ subscribe: (fn) => setInterval(() => fn(1), 1000) },
(prev, n) => prev + n,
)
.create();
computed
)import { computed } from "signux";
const first = state("Ada");
const last = state("Lovelace");
const fullName = computed(() => \`\${first()} \${last()}\`);
console.log(fullName()); // Ada Lovelace
import { event } from "signux";
const click = event<MouseEvent>();
click.subscribe((e) => console.log("Clicked!", e));
stateAsync
import { stateAsync } from "signux";
const user = stateAsync(fetchUser);
user.fetch(1);
const { loading, data, error } = user();
mapAsync
operatorimport { event } from "signux";
import { mapAsync } from "signux/operators";
const search = event<string>();
const results = search.pipe(mapAsync((query) => fetchResults(query))).toState();
Operators are chainable transformations for events and state:
import { map, filter, debounce } from "signux/operators";
const search = event<string>();
const trimmed = search.pipe(
map((s) => s.trim()),
filter((s) => s.length > 2),
debounce(300),
);
Available operators:
map
filter
debounce
merge
mapAsync
state<T>()
โ create mutable reactive statecomputed<T>()
โ create derived state that auto-tracks dependenciesevent<T>()
โ create an event emitter that can be observedstateAsync(fetcher)
โ reactive wrapper for async operations.pipe(...)
โ apply transformation operatorseffect(fn)
โ run a reactive side-effectAll primitives and operators are fully typed and documented using JSDoc.
IntelliSense works out of the box in TypeScript and modern editors.
No proxies. No magic. Just simple reactive building blocks you control.
MIT
FAQs
Reactive state and effect system for TypeScript, inspired by functional programming
The npm package signux receives a total of 5 weekly downloads. As such, signux popularity was classified as not popular.
We found that signux demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago.ย It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
Socket researchers uncover how browser extensions in trusted stores are used to hijack sessions, redirect traffic, and manipulate user behavior.
Research
Security News
An in-depth analysis of credential stealers, crypto drainers, cryptojackers, and clipboard hijackers abusing open source package registries to compromise Web3 development environments.
Security News
pnpm 10.12.1 introduces a global virtual store for faster installs and new options for managing dependencies with version catalogs.