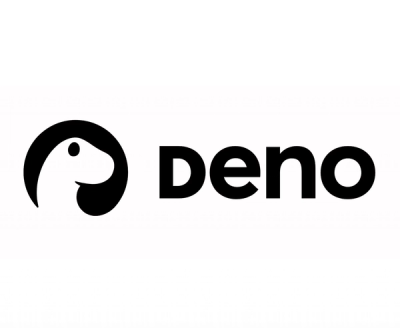
Security News
Deno 2.4 Brings Back deno bundle, Improves Dependency Management and Observability
Deno 2.4 brings back bundling, improves dependency updates and telemetry, and makes the runtime more practical for real-world JavaScript projects.
simple-array-generator
Advanced tools
Here are a few different ways to create an array. So simple and fast.
Here are a few different ways to create an array. So simple and fast.
const ARRAY_GEN = (x,y) => (function*(){
while (x <= y) yield x++;
})();
for (let res of ARRAY_GEN(1,5)){
console.log(res);
}
Output:
{
"output": "
1
2
3
4
5
"
}
const ARRAY_GEN = (x,y) => Array.from((function*(){
while (x <= y) yield x++;
})());
console.log(ARRAY_GEN(1,5));
Output:
{
"output": "[1, 2, 3, 4, 5]"
}
function range(s, e, str){
function *gen(s, e, str){
while(s <= e){
yield (!str) ? s : str[s]
s++
}
}
if (typeof s === 'string' && !str)
str = 'abcdefghijklmnopqrstuvwxyz'
const from = (!str) ? s : str.indexOf(s)
const to = (!str) ? e : str.indexOf(e)
// Return fonction.
return [...gen(from, to, str)]
}
// console.log(range('a', 'e'))
// For Lowercase
// [ 'a', 'b', 'c', 'd', 'e' ]
// console.log(range('a', 'e').map(v=>v.to.reverse())
// For Lowercase and Reverse
// [ 'e', 'd', 'c', 'b', 'a' ]
// console.log(range('a', 'e').map(v=>v.toUpperCase()))
// For Uppercase
// [ 'A', 'B', 'C', 'D', 'E' ]
// console.log(range('a', 'e').map(v=>v.toUpperCase()).reverse())
// For Uppercase and Reverse
// [ 'E', 'D', 'C', 'B', 'A' ]
FAQs
Here are a few different ways to create an array. So simple and fast.
The npm package simple-array-generator receives a total of 3 weekly downloads. As such, simple-array-generator popularity was classified as not popular.
We found that simple-array-generator demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Deno 2.4 brings back bundling, improves dependency updates and telemetry, and makes the runtime more practical for real-world JavaScript projects.
Security News
CVEForecast.org uses machine learning to project a record-breaking surge in vulnerability disclosures in 2025.
Security News
Browserslist-rs now uses static data to reduce binary size by over 1MB, improving memory use and performance for Rust-based frontend tools.