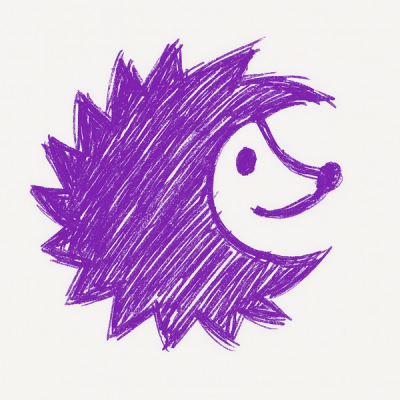
Security News
Browserslist-rs Gets Major Refactor, Cutting Binary Size by Over 1MB
Browserslist-rs now uses static data to reduce binary size by over 1MB, improving memory use and performance for Rust-based frontend tools.
solvemedia.js
Advanced tools
An (unofficial) JavaScript wrapper for the SolveMedia API.
An (unofficial) JavaScript wrapper for the SolveMedia API.
A quick example as a CLI (which doesn't really make sense, but you get the idea).
require("dotenv").config()
const open = require("open")
const inquirer = require("inquirer")
const { SolveMediaClient } = require("solvemedia.js")
const solvemedia = new SolveMediaClient()
;(async () => {
await solvemedia.login(process.env.C_KEY, process.env.V_KEY, process.env.H_KEY)
const challenge = await solvemedia.getChallenge()
await challenge.writeImageToFile("./captcha.png")
await open("./captcha.png")
const { answer } = await inquirer.prompt([{
name: "answer",
message: "Type the content of the captcha:"
}])
const verified = await challenge.verify(answer)
console.log(verified ? "Hello, human!" : "Beep boop!" )
})()
This is captcha.png
:
This is the CLI itself (running the program twice):
I recommend storing your authorization keys in a .env
file and using a library like dotenv
, if your project is open source.
In SolveMedia, once a client has requested the image of a certain challenge, it will start redirecting following requests to a "media error" image.
This means that once you used, for example Challenge#getImageBuffer()
, you won't be able to use Challenge#writeImageToFile()
or Challenge#getImageBuffer()
again on the same challenge; they will throw a SolveMediaAPIError
with error code IMAGE_USED
.
SolveMedia also requires for the image URL to be used before verifying it; not doing so will throw a SolveMediaAPIError
with error code IMAGE_UNUSED
.
const { SolveMediaClient, Challenge, SolveMediaAPIError, AuthorizationError } = require("solvemedia.js")
new SolveMediaClient()
Store and validate your SolveMedia credentials.
name | description | type | default |
---|---|---|---|
challengeKey | Your challenge key | string | |
verificationKey | Your verification key | string | null |
authenticationHashKey | Your authentication hash key | string | null |
validate | Whether to validate the given key(s) by requesting a challenge | boolean | true |
Obtain a challenge/captcha from the SolveMedia API.
name | description | type | default |
---|---|---|---|
userIP | The user's IP, to increase/decrease the difficulty accordingly | string | null (random IP) |
You probably shouldn't initialize this class manually, use SolveMediaClient#getChallenge()
instead.
new Challenge(body, auth, userIP)
name | description | type | default |
---|---|---|---|
body | Options to personalize the image | string | |
auth | The client's authorization keys | object | |
auth.challengeKey | The client's challenge key | string | |
auth.verificationKey | The client's verification key | string | null |
auth.authenticationHashKey | The client's authentication hash key | string | null |
userIP | The user's IP | string |
Verify the user's answer. Requires the verification key to be stored.
name | description | type | default |
---|---|---|---|
answer | The user's answer | string | |
authenticateResponse | Whether to authenticate SolveMedia's response (requires the authentication hash key) | boolean | true |
Get the URL of the image.
name | description | type | default |
---|---|---|---|
options | Options to personalize the image | object | {} |
options.width | The width of the image | number | 300 |
options.height | The height of the image | number | 150 |
options.foreground | The foreground color of the image | string | "000000" |
options.background | The background color of the image | string | "f8f8f8" |
Get the image as a buffer.
name | description | type | default |
---|---|---|---|
imageOptions | Options to personalize the image, passed to getImageURL() | object | {} |
Write the image to a file.
name | description | type | default |
---|---|---|---|
path | The path of the new file | string | |
imageOptions | Options to personalize the image, passed to getImageURL() | object | {} |
Thrown when the response from SolveMedia is either invalid, or contains an error.
new SolveMediaAPIError(code, unknownErrorMessage)
name | description | type | default |
---|---|---|---|
code | The error code | string | |
unknownErrorMessage | The SM error message to format the "UNKNOWN_ERROR" message | string | null |
The error code.
The possible error codes are:
UNKNOWN_ERROR
: Unknown error: {...}
JSON_INVALID
: Response body is not valid JSON.
BODY_INCOMPLETE
: Response body does not contain the necessary values.
IMAGE_UNUSED
: The image URL has to be used before verifying an answer.
IMAGE_USED
: The image URL has already been used.
IP_INVALID
: Invalid IP address.
CHALLENGE_ALREADY_VERIFIED
: This challenge has already been verified.
CHALLENGE_INVALID
: Invalid challenge ID.
CHALLENGE_EXPIRED
: This challenge has expired.
The error message.
Thrown when the provided credentials are invalid or unavailable to the client.
new SolveMediaAPIError(code, unknownErrorMessage)
name | description | type | default |
---|---|---|---|
code | The error code | string |
The error code.
The possible error codes are:
AUTH_MISSING
: Credentials are unavailable.
CKEY_MISSING
: Challenge key is unavailable.
CKEY_INVALID
: Invalid challenge key.
VKEY_MISSING
: Verification key is unavailable.
VKEY_INVALID
: Invalid verification key.
HKEY_MISSING
: Authentication hash key is unavailable.
RESPONSE_NOT_AUTHENTIC
: The response is not authentic, or the authentication hash key is invalid.
FAQs
An (unofficial) JavaScript wrapper for the SolveMedia API.
The npm package solvemedia.js receives a total of 0 weekly downloads. As such, solvemedia.js popularity was classified as not popular.
We found that solvemedia.js demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Browserslist-rs now uses static data to reduce binary size by over 1MB, improving memory use and performance for Rust-based frontend tools.
Research
Security News
Eight new malicious Firefox extensions impersonate games, steal OAuth tokens, hijack sessions, and exploit browser permissions to spy on users.
Security News
The official Go SDK for the Model Context Protocol is in development, with a stable, production-ready release expected by August 2025.