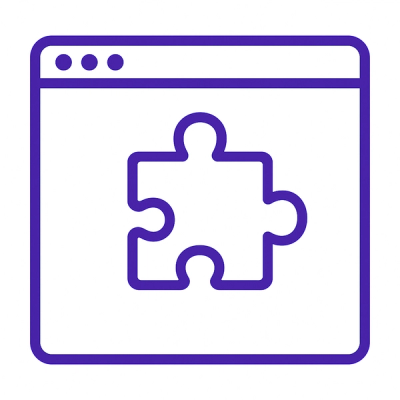
Research
Security News
The Growing Risk of Malicious Browser Extensions
Socket researchers uncover how browser extensions in trusted stores are used to hijack sessions, redirect traffic, and manipulate user behavior.
sorted-array-functions
Advanced tools
Maintain and search through a sorted array using some low level functions
The sorted-array-functions npm package provides utility functions for manipulating and querying sorted arrays. It ensures that the arrays remain sorted after operations such as insertion, deletion, and searching.
Insertion
This feature allows you to insert an element into a sorted array while maintaining the order.
const sortedArrayFunctions = require('sorted-array-functions');
let arr = [1, 3, 5, 7];
sortedArrayFunctions.add(arr, 4); // arr is now [1, 3, 4, 5, 7]
Deletion
This feature allows you to remove an element from a sorted array while maintaining the order.
const sortedArrayFunctions = require('sorted-array-functions');
let arr = [1, 3, 4, 5, 7];
sortedArrayFunctions.remove(arr, 4); // arr is now [1, 3, 5, 7]
Binary Search
This feature allows you to perform a binary search on a sorted array to find the index of a given element.
const sortedArrayFunctions = require('sorted-array-functions');
let arr = [1, 3, 5, 7];
let index = sortedArrayFunctions.search(arr, 5); // index is 2
Merge
This feature allows you to merge two sorted arrays into one sorted array.
const sortedArrayFunctions = require('sorted-array-functions');
let arr1 = [1, 3, 5];
let arr2 = [2, 4, 6];
let merged = sortedArrayFunctions.merge(arr1, arr2); // merged is [1, 2, 3, 4, 5, 6]
Lodash is a popular utility library that provides a wide range of functions for manipulating arrays, objects, and other data types. It includes functions for sorting, searching, and manipulating arrays, but it is a more general-purpose library compared to sorted-array-functions.
Underscore is another utility library similar to Lodash. It provides a variety of functions for working with arrays, objects, and other data types. While it includes functions for sorting and searching arrays, it is not specifically focused on sorted arrays like sorted-array-functions.
The binary-search package provides functions specifically for performing binary search operations on sorted arrays. It is more focused than general utility libraries like Lodash and Underscore, but it does not offer the full range of sorted array manipulations that sorted-array-functions does.
Maintain and search through a sorted array using some low level functions
npm install sorted-array-functions
var sorted = require('sorted-array-functions')
var list = []
sorted.add(list, 1)
sorted.add(list, 4)
sorted.add(list, 2)
console.log(list) // prints out [1, 2, 4]
console.log(sorted.has(list, 2)) // returns true
console.log(sorted.has(list, 3)) // returns false
console.log(sorted.eq(list, 2)) // returns 1 (the index)
console.log(sorted.gt(list, 2)) // returns 2
console.log(sorted.gt(list, 4)) // returns -1
sorted.add(list, value, [compare])
Insert a new value into the list sorted.
Optionally you can use a custom compare function that returns, compare(a, b)
that returns 1 if a > b
, 0 if a === b
and -1 if a < b
.
sorted.addFromFront(list, value, [compare])
Inserts a new value (same result as sorted.add()
) optimized for prepend.
var bool = sorted.remove(list, value, [compare])
Remove a value. Returns true if the value was in the list.
var bool = sorted.has(list, value, [compare])
Check if a value is in the list.
var index = sorted.eq(list, value, [compare])
Get the index of a value in the list (uses binary search). If the value could not be found -1 is returned.
var index = sorted.gte(list, value, [compare])
Get the index of the first value that is >=
.
If the value could not be found -1 is returned.
var index = sorted.gt(list, value, [compare])
Get the index of the first value that is >
.
If the value could not be found -1 is returned.
var index = sorted.lte(list, value, [compare])
Get the index of the first value that is <=
.
If the value could not be found -1 is returned.
var index = sorted.lt(list, value, [compare])
Get the index of the first value that is <
.
If the value could not be found -1 is returned.
MIT
FAQs
Maintain and search through a sorted array using some low level functions
The npm package sorted-array-functions receives a total of 1,889,276 weekly downloads. As such, sorted-array-functions popularity was classified as popular.
We found that sorted-array-functions demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
Socket researchers uncover how browser extensions in trusted stores are used to hijack sessions, redirect traffic, and manipulate user behavior.
Research
Security News
An in-depth analysis of credential stealers, crypto drainers, cryptojackers, and clipboard hijackers abusing open source package registries to compromise Web3 development environments.
Security News
pnpm 10.12.1 introduces a global virtual store for faster installs and new options for managing dependencies with version catalogs.