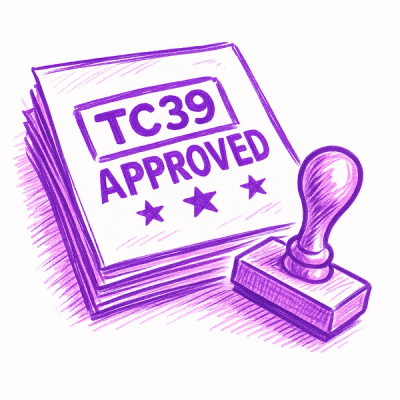
Security News
TC39 Advances 11 Proposals for Math Precision, Binary APIs, and More
TC39 advances 11 JavaScript proposals, with two moving to Stage 4, bringing better math, binary APIs, and more features one step closer to the ECMAScript spec.
specla-database
Advanced tools
This module is the database handler for the Specla Framework, its like Laravels Eloquent but just for javascript and MongoDB.
To install specla-database all you have to do is to download it via npm.
npm install specla-database --save
let Database = require('specla-database');
const DB = new Database({
driver: 'mongo',
host: '127.0.0.1',
port: 27017,
database: 'MyDB'
});
Specla database includes two modules the Query builder
and the DB.Model
.
DB.collection('users').get((err, result) => {
// Return all users at once
});
DB.collection('users').stream((user) => {
// do something with the data
return user;
}).done((result) => {
// returns the streamed data in an array
});
let schema = {
name: String,
age: Number,
address: {
city: String,
},
skills: Array,
admin: Boolean,
};
let data = {
name: 'Frederik',
age: 22,
address: {
city: 'Odense',
},
skills: ['Javascript'],
admin: true
};
DB.collection('users')
.schema(schema)
.insert(data, (err, result) => {
// do something when the operation is completed
});
DB.collection('users')
.where('name', 'John')
.get((err, result) => {
// do something with the data
});
It's also possible to just parse an object to the where()
like below
.where({ name: 'John' })
.sort('name', 'ASC')
.skip(5)
.limit(10)
let data = {
name: 'John',
};
DB.collection('users')
.insert(data, (err, result) => {
// Do something after data is inserted
});
let data = {
name: 'John',
};
DB.collection('users')
.where('_id', '5748aa5d45af47fc9909310b')
.update(data, (err, result) => {
// Do something after data is updated
});
DB.collection('users')
.where('_id', '5748aa5d45af47fc9909310b')
.remove((err, result) => {
// Do something after data is removed
});
If there is some Mongo functionality which isn't supported yet in this module, you can then use the raw method and have full access to the Mongo object.
Its important to notice when youre using the raw method, you have to manually close the db connection with the
done
callback
DB.raw((db, done) => {
var cursor = db.collection('users').find({});
cursor.each((err, doc) => {
if(doc !== null){
console.log(doc);
} else {
done(); // CLOSE CONNECTION!!!!
}
});
})
// the simplest model you can create
class User extends DB.Model {
collection(){
return 'users';
}
// the schema is optional
schema(){
return {
name: String,
age: Number
};
}
}
let user = new User;
user.set('name', 'John');
user.save(() => {
// do something when your user is created
console.log(user.get('_id'));
});
User.find('5748aa5d45af47fc9909310b', (err, user) => {
// do something with the user
});
User.find('5748aa5d45af47fc9909310b', (err, user) => {
user.set('name', 'Frederik');
user.save(() => {
// do something
});
});
User.find('5748aa5d45af47fc9909310b', (err, user) => {
user.delete(() => {
// do something
});
});
FAQs
database module
The npm package specla-database receives a total of 1 weekly downloads. As such, specla-database popularity was classified as not popular.
We found that specla-database demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
TC39 advances 11 JavaScript proposals, with two moving to Stage 4, bringing better math, binary APIs, and more features one step closer to the ECMAScript spec.
Research
/Security News
A flawed sandbox in @nestjs/devtools-integration lets attackers run code on your machine via CSRF, leading to full Remote Code Execution (RCE).
Product
Customize license detection with Socket’s new license overlays: gain control, reduce noise, and handle edge cases with precision.