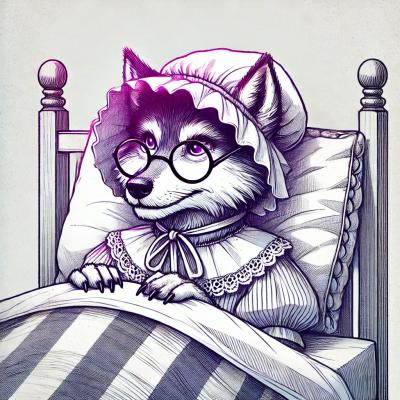
Security News
GitHub Removes Malicious Pull Requests Targeting Open Source Repositories
GitHub removed 27 malicious pull requests attempting to inject harmful code across multiple open source repositories, in another round of low-effort attacks.
sphero-pwn
Advanced tools
This is a node.js driver for the communication protocol used by Sphero's robots.
This project is an independent effort from the official sphero.js project, and is less supported. At the same time, we are free to develop functionality that is unlikely to be added to the official project, such as driving a BB-8.
This project is written in CoffeeScript and tested using mocha.
This package's API relies heavily on ES6 Promises.
Each robot that your computer can connect to receives an identifier. The following command looks for reachable robots and shows their identifiers.
npm start
Once you have an identifier, you can use the discovery service to connect to the robot, as shown below. The discovery service has other useful methods as well.
var sphero = require('sphero-pwn');
var robot = null;
sphero.Discovery.findChannel('serial:///dev/cu.Sphero-YRG-AMP-SPP').
then(foundChannel); // foundChannel is defined below.
The discovery service produces a communication channel, which can be used to
create a Robot
. Robot's methods are convenient wrappers
for the
Sphero API commands.
function foundChannel(channel) {
console.log("Found robot");
robot = new sphero.Robot(channel);
play(); // play is defined below.
}
For example, the following snippet sets the Sphero's permanent RGB LED color.
function play() {
robot.setUserRgbLed({red: 255, green: 128, blue: 0}).
then(function() {
console.log("Set LED color");
robot.close();
}).
then(function() {
console.log("Done");
});
}
The following example uses orbBasic to flash the robot's RGB LED.
function play() {
robot.on('basic', function(event) {
console.log("basic print: " + event.message);
});
robot.on('basicError', function(event) {
console.log("basic error: " + event.message);
});
var script = "10 RGB 0, 255, 0\n" +
"20 delay 2000\n" +
"30 RGB 0, 0, 255\n" +
"40 delay 2000\n";
robot.loadBasic("ram", script).
then(function() {
console.log("Loaded script");
robot.runBasic("ram", 10);
}).
then(function() {
console.log("Started script");
robot.close();
}).
then(function() {
console.log("Done");
}).
catch(function() {
console.error(error)
});
}
Last, the following example uses our macro compiler to compile and execute a macro that flashes the robot's RGB LED.
var macros = require('sphero-pwn-macros');
function play() {
var macroSource = "rgb 0 255 0\n" +
"delay 2000\n" +
"rgb 0 0 255\n" +
"delay 2000\n";
var macro = macros.compile(macroSource);
robot.on('macro', function(event) {
console.log("macro marker: " + event.markerId);
});
robot.loadMacro(0xFF, new Buffer(macro.bytes)).
then(function() {
console.log("Loaded macro in RAM");
robot.runMacro(0xFF);
}).
then(function() {
console.log("Started macro");
robot.close();
}).
then(function() {
console.log("Done");
}).
catch(function() {
console.error(error)
});
}
Install all the dependencies.
npm install
List the Bluetooth devices connected to your computer.
npm start
Set the SPHERO_DEV
environment variable to point to your Sphero.
export SPHERO_DEV=serial:///dev/cu.Sphero-XXX-AMP-SPP
export SPHERO_DEV=ble://ef:80:a8:4a:12:34
Run the tests.
npm test
This project is Copyright (c) 2015 Victor Costan, and distributed under the MIT License.
FAQs
Driver for Sphero robots
The npm package sphero-pwn receives a total of 0 weekly downloads. As such, sphero-pwn popularity was classified as not popular.
We found that sphero-pwn demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
GitHub removed 27 malicious pull requests attempting to inject harmful code across multiple open source repositories, in another round of low-effort attacks.
Security News
RubyGems.org has added a new "maintainer" role that allows for publishing new versions of gems. This new permission type is aimed at improving security for gem owners and the service overall.
Security News
Node.js will be enforcing stricter semver-major PR policies a month before major releases to enhance stability and ensure reliable release candidates.