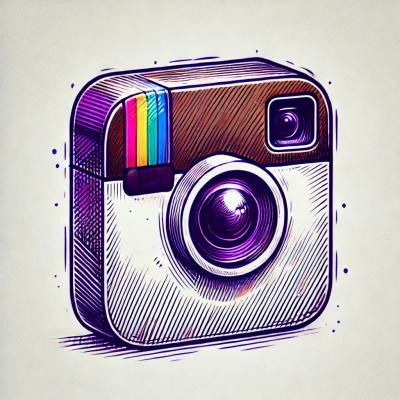
Research
PyPI Package Disguised as Instagram Growth Tool Harvests User Credentials
A deceptive PyPI package posing as an Instagram growth tool collects user credentials and sends them to third-party bot services.
spotify-react-query
Advanced tools
Simple React Query hooks for the Spotify Web API. With the power of React Query, requests for Spotify resources are automatically cached, and by leveraging dataloader under the hood, we can batch calls for similar resources to avoid using up your Spotify API quota.
This package is used by the musictaste.space beta.
Install this package by running the following command in your project:
yarn add spotify-react-query
In order to use the hooks, you must wrap dependent components in a SpotifyQueryProvider
and pass in a React Query QueryClient
. The client can be customized to suit your use case, or you can pass in the default client and it will work out of the box.
You must also provide a Spotify Client instance from spotify-web-api-node
. The library will not perform any requests until an access token is set on the client. You will need to manage the lifecycle of token refreshes outside of <SpotifyQueryProvider>
context.
import { QueryClient } from "@tanstack/react-query"
import SpotifyClient from "spotify-web-api-node"
const query = new QueryClient()
const spotify = new SpotifyClient()
// this is usually managed inside your application
spotify.setAccessToken("<ACCESS_TOKEN>")
const App = () => {
return (
<SpotifyQueryProvider query={query} spotify={spotify}>
<DependentComponents />
</SpotifyQueryProvider>
)
}
import { useSimplifiedTrack } from "spotify-react-query"
function TrackComponent({ uri }: { uri: string }) {
const { data: track, isLoading } = useSimplifiedTrack(uri)
if (isLoading) {
return <div>Loading...</div>
}
if (!track) {
return null
}
return (
<div>
{track.name} by ${track.artists[0].name}
</div>
)
}
For many Spotify API entities, there are two subtypes which are returned depending on your query - simplified
and full
. Please refer to the Spotify API documentation to differentiate between the two given the entity. In the majority of cases, the simplified result may be enough.
Under the hood, when a query fetches simplified data about related entities (eg. when you query for an album and it returns simplified artist and album tracks), the library will prime the cache with those entities. This means that if you first used the useFullAlbum
hook to fetch an album, and then use a component leveraging the useSimplifiedTrack
hook to render the tracks based on the album track URIs, the data will already be in the cache and an additional network request will not be made.
For this reason, it's recommended that you use the simplified entities wherever possible.
function useSimplifiedTrack(id: string, options?: ReactQueryOptions)
function useFullTrack(id: string, options?: ReactQueryOptions)
function useFullTracks(ids: string[], options?: ReactQueryOptions)
function useSimplifiedAlbum(id: string, options?: ReactQueryOptions)
function useFullAlbum(id: string, options?: ReactQueryOptions)
function useSimplifiedArtist(id: string, options?: ReactQueryOptions)
function useFullArtist(id: string, options?: ReactQueryOptions)
function useFullArtists(ids: string[], options?: ReactQueryOptions)
function usePlaylist(id: string, options?: ReactQueryOptions)
function usePlaylistTracks({
variables?: { id: string; fields?: string; limit?: number; offset?: number; market?: string } },
options?: ReactQueryOptions
)
function useUserTopTracks(
variables: { limit?: number; offset?: number; time_range: "short_term" | "medium_term" | "long_term" },
options?: ReactQueryOptions
)
function useUserTopArtists(
variables: { limit?: number; offset?: number; time_range: "short_term" | "medium_term" | "long_term" },
options?: ReactQueryOptions
)
function useRecentlyPlayedTracks(
variables: { after?: number; before?: number; limit?: number },
options?: ReactQueryOptions
)
You can also use the Spotify client directly to leverage all the methods available via spotify-web-api-node
import { useSpotifyClient } from "spotify-react-query"
const client = useSpotifyClient()
client.getMe().then((res) => console.log(res.body))
FAQs
React Query hooks for the Spotify Web API
The npm package spotify-react-query receives a total of 1 weekly downloads. As such, spotify-react-query popularity was classified as not popular.
We found that spotify-react-query demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
A deceptive PyPI package posing as an Instagram growth tool collects user credentials and sends them to third-party bot services.
Product
Socket now supports pylock.toml, enabling secure, reproducible Python builds with advanced scanning and full alignment with PEP 751's new standard.
Security News
Research
Socket uncovered two npm packages that register hidden HTTP endpoints to delete all files on command.