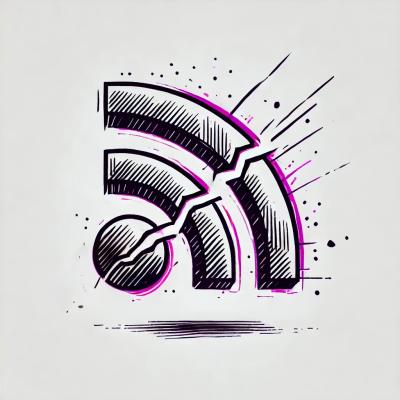
Security News
CISA Kills Off RSS Feeds for KEVs and Cyber Alerts
CISA is discontinuing official RSS support for KEV and cybersecurity alerts, shifting updates to email and social media, disrupting automation workflows.
sql-migrations
Advanced tools
raw SQL migrations for node
In your project
// migrate.js
var path = require('path');
require('sql-migrations').run({
// configuration here. See the Configuration section
});
run node ./migrate.js
with arguments
node ./migrate create migration_name
will create two migration files (up and down)
./migrations/1415860098827_up_migration_name.sql
./migrations/1415860098827_down_migration_name.sql
node ./migrate migrate
will run all pending migrations
node ./migrate.js rollback
will rollback the last migration if there is one
In your project
require('sql-migrations').migrate({
// configuration here. See the Configuration section
});
This returns a promise which resolves/rejects whenever the migration is complete.
In your project
require('sql-migrations').rollback({
// configuration here. See the Configuration section
});
This returns a promise which resolves/rejects whenever the rollback is complete.
Configuration should be specified as below:
var configuration = {
migrationsDir: path.resolve(__dirname, 'migrations'), // This is the directory that should contain your SQL migrations.
host: 'localhost', // Database host
port: 5432, // Database port
db: 'sql_migrations', // Database name
user: 'dabramov', // Database username
password: 'password', // Database password
adapter: 'pg', // Database adapter: pg, mysql
// Parameters are optional. If you provide them then any occurrences of the parameter (i.e. FOO) in the SQL scripts will be replaced by the value (i.e. bar).
parameters: {
"FOO": "bar"
},
minMigrationTime: new Date('2018-01-01').getTime() // Optional. Skip migrations before this before this time.
};
You can also swap out the default logger (the console
object) for another one that supports the log and error methods. You should do this before running any other commands:
require('sql-migrations').setLogger({
log: function() {},
error: function() {}
});
Write raw sql in your migrations. You can also include placeholders which will be substituted. example
-- ./migrations/1415860098827_up_migration_name.sql
create table "test_table" (id bigint, name varchar(255));
-- ./migrations/1415860098827_down_migration_name.sql
drop table "test_table";
FAQs
raw SQL migrations library for Node.js
The npm package sql-migrations receives a total of 274 weekly downloads. As such, sql-migrations popularity was classified as not popular.
We found that sql-migrations demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 2 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
CISA is discontinuing official RSS support for KEV and cybersecurity alerts, shifting updates to email and social media, disrupting automation workflows.
Security News
The MCP community is launching an official registry to standardize AI tool discovery and let agents dynamically find and install MCP servers.
Research
Security News
Socket uncovers an npm Trojan stealing crypto wallets and BullX credentials via obfuscated code and Telegram exfiltration.