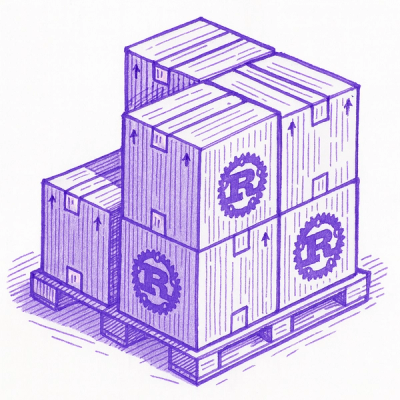
Security News
Crates.io Implements Trusted Publishing Support
Crates.io adds Trusted Publishing support, enabling secure GitHub Actions-based crate releases without long-lived API tokens.
The ssim.js npm package is used for calculating the Structural Similarity Index (SSIM) between two images. SSIM is a method for measuring the similarity between two images, which is often used in the context of image quality assessment.
Calculate SSIM between two images
This feature allows you to calculate the SSIM between two images. The `compare` function takes the paths to two images and returns a promise that resolves with the SSIM value.
const ssim = require('ssim.js');
const img1 = 'path/to/image1.png';
const img2 = 'path/to/image2.png';
ssim.compare(img1, img2).then(result => {
console.log('SSIM:', result.ssim);
}).catch(err => {
console.error(err);
});
Calculate SSIM for image buffers
This feature allows you to calculate the SSIM between two image buffers. The `compare` function can also take image buffers as input and returns a promise that resolves with the SSIM value.
const ssim = require('ssim.js');
const fs = require('fs');
const img1Buffer = fs.readFileSync('path/to/image1.png');
const img2Buffer = fs.readFileSync('path/to/image2.png');
ssim.compare(img1Buffer, img2Buffer).then(result => {
console.log('SSIM:', result.ssim);
}).catch(err => {
console.error(err);
});
The image-ssim package is another library for calculating the Structural Similarity Index (SSIM) between two images. It provides similar functionality to ssim.js but may have different performance characteristics and API design.
The sharp package is a high-performance image processing library. While its primary focus is on image transformations (resizing, cropping, etc.), it also includes functionality for comparing images, including SSIM calculations. Sharp is known for its speed and efficiency.
The gm (GraphicsMagick) package is a Node.js wrapper for the GraphicsMagick and ImageMagick tools. It provides a wide range of image processing capabilities, including image comparison and SSIM calculations. It is a more comprehensive tool for image manipulation compared to ssim.js.
Get a
0
to1
score on how similar two images are
The closer SSIM is to 1
the higher the similarity. It correlates better with subjective ratings than other measures like PSNR and MSE. For instance:
![]() | ![]() | ![]() |
Original, MSE = 0, SSIM = 1 | MSE = 144, SSIM = 0.988 | MSE = 144, SSIM = 0.913 |
![]() | ![]() | ![]() |
MSE = 144, SSIM = 0.840 | MSE = 144, SSIM = 0.694 | MSE = 142, SSIM = 0.662 |
Table extracted from http://www.cns.nyu.edu/~lcv/ssim/
npm install ssim.js
You can also use the web version directly from unpkg's CDN: https://unpkg.com/ssim.js@{{version}}/dist/ssim.web.js
.
Check out the playground for node and web usage examples.
SSIM.js takes in 2 image buffers and optional options parameter. You can find all options available here.
For examples on how to implement image loading strategies for both node and the web, check out this wiki page.
import ssim from "ssim.js";
const img1 = loadImage("./img1.jpg");
const img2 = loadImage("./img2.jpg");
const { mssim, performance } = ssim(img1, img2);
console.log(`SSIM: ${mssim} (${performance}ms)`);
If you run into any issues or want a more info, check the wiki.
The code is fully documented but feel free to create an issue here if you have any questions.
Process | Status |
---|---|
Versioning | |
Dependencies |
This project is a direct port of algorithms published by Wang, et al. 2004 on "Image Quality Assessment: From Error Visibility to Structural Similarity". The original Matlab scripts are available here with their datasets. To view the steps taken to validate ssim.js
results, check the wiki.
The current (default) implementation uses the Weber algorithm, originally developed by Dan Weber, 2020 at notatypical.agency.
FAQs
JavaScript implementation of the SSIM algorithm
The npm package ssim.js receives a total of 492,405 weekly downloads. As such, ssim.js popularity was classified as popular.
We found that ssim.js demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Crates.io adds Trusted Publishing support, enabling secure GitHub Actions-based crate releases without long-lived API tokens.
Research
/Security News
Undocumented protestware found in 28 npm packages disrupts UI for Russian-language users visiting Russian and Belarusian domains.
Research
/Security News
North Korean threat actors deploy 67 malicious npm packages using the newly discovered XORIndex malware loader.