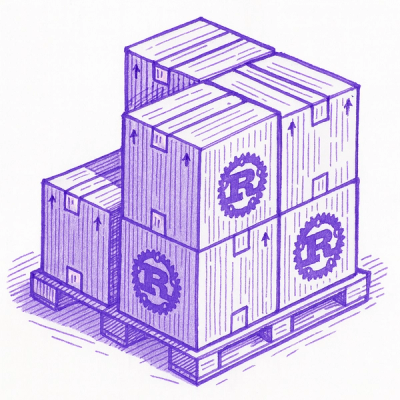
Security News
Crates.io Implements Trusted Publishing Support
Crates.io adds Trusted Publishing support, enabling secure GitHub Actions-based crate releases without long-lived API tokens.
stream-combiner
Advanced tools
[](https://npmjs.org/package/stream-combiner) [](https://travis-ci.org/dominictarr/stream-combiner)
The stream-combiner npm package is used to combine multiple Node.js streams (readable or writable) into a single stream. This is particularly useful for managing stream pipelines and handling data transformations in a more organized and readable manner.
Stream Pipeline Creation
This code sample demonstrates how to create a stream pipeline using stream-combiner. It combines stdin, a decryption stream, a gzip compression stream, and stdout into a single stream pipeline. Errors in any part of the stream are handled by logging to the console.
const { combine } = require('stream-combiner');
const zlib = require('zlib');
const crypto = require('crypto');
const combinedStream = combine(
process.stdin,
crypto.createDecipher('aes192', 'a_secret'),
zlib.createGzip(),
process.stdout
);
combinedStream.on('error', console.error);
Pump is a small node module that pipes streams together and destroys all of them if one of them closes. Compared to stream-combiner, pump provides a similar functionality but with a focus on properly cleaning up the streams to avoid memory leaks, which is not explicitly handled by stream-combiner.
Multistream allows you to combine multiple streams into a single readable stream. It differs from stream-combiner in that it focuses more on sequential rather than parallel stream combination, making it suitable for scenarios where streams need to be processed one after another.
Turn a pipeline into a single stream. Combine
returns a stream that writes to the first stream
and reads from the last stream.
Listening for 'error' will recieve errors from all streams inside the pipe.
var Combine = require('stream-combiner')
var es = require('event-stream')
Combine( // connect streams together with `pipe`
process.openStdin(), // open stdin
es.split(), // split stream to break on newlines
es.map(function (data, callback) { // turn this async function into a stream
var repr = util.inspect(JSON.parse(data)) // render it nicely
callback(null, repr)
}),
process.stdout // pipe it to stdout !
)
Can also be called with an array:
var combinedStream = Combine([
stream1,
stream2,
]);
Or to combine gulp plugins:
function coffeePipe() {
return Combine(
coffeescript(),
coffeelint.reporter('fail').on('error', function(){
gutil.beep()
gulp.run('lint')
})
}
//usage:
gulp.src().pipe(coffeePipe());
MIT
FAQs
[](https://npmjs.org/package/stream-combiner) [](https://travis-ci.org/dominictarr/stream-combiner)
The npm package stream-combiner receives a total of 4,612,499 weekly downloads. As such, stream-combiner popularity was classified as popular.
We found that stream-combiner demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Crates.io adds Trusted Publishing support, enabling secure GitHub Actions-based crate releases without long-lived API tokens.
Research
/Security News
Undocumented protestware found in 28 npm packages disrupts UI for Russian-language users visiting Russian and Belarusian domains.
Research
/Security News
North Korean threat actors deploy 67 malicious npm packages using the newly discovered XORIndex malware loader.