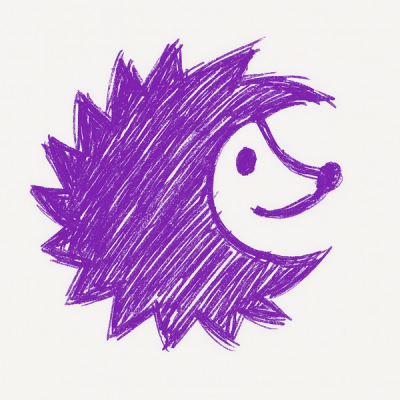
Security News
Browserslist-rs Gets Major Refactor, Cutting Binary Size by Over 1MB
Browserslist-rs now uses static data to reduce binary size by over 1MB, improving memory use and performance for Rust-based frontend tools.
string-array
Advanced tools
Parse string into array of string elements.
"[ hello, world, [ 1, [2, [ 3 ]]]]"
:arrow_right: [ "hello", "world", [ "1", [ "2", [ "3" ]]]]
npm i --save string-array
const stringArray = require("string-array");
const r1 = stringArray.parse("[]");
// r1 === { prefix: "", array: [], remain: "" }
const r2 = stringArray.parse("test[1,2,3]");
// r2 === { prefix: "test", array: ["1","2","3"], remain: "" }
Elements are automatically treated as strings, so quotes '
, "
and backtick are taken as part of element.
All leading and trailing whitespaces are automatically trim
ed.
Can't have these characters in elements: ,
[
]
stringArray.parse("")
:stringArray.parse("[]")
:{
prefix: "",
array: [],
remain: ""
}
stringArray.parse('test[1,2,"3"]')
:{
prefix: "test",
array: ["1","2", '"3"'],
remain: ""
}
stringArray.parse("[hello, world, [ [ foo, bar ], 1, [ 2 ], 3 ] ] some other stuff [blah]")
:{
prefix: "",
array: ["hello", "world", [ ["foo", "bar"], "1", ["2"], "3" ] ],
remain: "some other stuff [blah]"
}
More samples in test
stringArray.parse(str, noPrefix, noExtra)
str
- string array to be parsednoPrefix
- if true
, then do not check for a prefixnoExtra
- if true
, then do not allow trailing text following a complete array in str
AssertionError("array missing [")
str
doesn't start with [
and noPrefix
is true
AssertionError("array missing ]")
AssertionError("array has extra ]")
AssertionError("extra data at end of array")
- if noExtra
is true
and there are extra text following a complete array in str
Licensed under the Apache License, Version 2.0
FAQs
Parse string into array of string elements
The npm package string-array receives a total of 10,368 weekly downloads. As such, string-array popularity was classified as popular.
We found that string-array demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Browserslist-rs now uses static data to reduce binary size by over 1MB, improving memory use and performance for Rust-based frontend tools.
Research
Security News
Eight new malicious Firefox extensions impersonate games, steal OAuth tokens, hijack sessions, and exploit browser permissions to spy on users.
Security News
The official Go SDK for the Model Context Protocol is in development, with a stable, production-ready release expected by August 2025.