Comparing version
/** | ||
* Splits a string into an array of substrings. | ||
* T: The string to split. | ||
* delimiter: The delimiter. | ||
*/ | ||
type Split<T, delimiter extends string = ''> = T extends `${infer first}${delimiter}${infer rest}` ? [first, ...Split<rest, delimiter>] : T extends '' ? [] : [T]; | ||
/** | ||
* A strongly-typed version of `String.prototype.split`. | ||
* @param str the string to split. | ||
* @param delimiter the delimiter. | ||
* @returns the splitted string in both type level and runtime. | ||
* @example split('hello world', ' ') // ['hello', 'world'] | ||
*/ | ||
declare function split<T extends string, D extends string = ''>(str: T, delimiter?: D): Split<T, D>; | ||
/** | ||
* Gets the character at the given index. | ||
* T: The string to get the character from. | ||
* index: The index of the character. | ||
*/ | ||
type CharAt<T extends string, index extends number> = Split<T>[index]; | ||
/** | ||
* A strongly-typed version of `String.prototype.charAt`. | ||
* @param str the string to get the character from. | ||
* @param index the index of the character. | ||
* @returns the character in both type level and runtime. | ||
* @example charAt('hello world', 6) // 'w' | ||
*/ | ||
declare function charAt<T extends string, I extends number>(str: T, index: I): CharAt<T, I>; | ||
/** | ||
* Joins a tuple of strings with the given delimiter. | ||
* T: The tuple of strings to join. | ||
* delimiter: The delimiter. | ||
*/ | ||
type Join<T extends readonly string[], delimiter extends string = ''> = string[] extends T ? string : T extends readonly [ | ||
infer first extends string, | ||
...infer rest extends string[] | ||
] ? rest extends [] ? first : `${first}${delimiter}${Join<rest, delimiter>}` : ''; | ||
/** | ||
* A strongly-typed version of `Array.prototype.join`. | ||
* @param tuple the tuple of strings to join. | ||
* @param delimiter the delimiter. | ||
* @returns the joined string in both type level and runtime. | ||
* @example join(['hello', 'world'], '-') // 'hello-world' | ||
*/ | ||
declare function join<const T extends readonly string[], D extends string = ''>(tuple: T, delimiter?: D): Join<T, D>; | ||
/** | ||
* Concatenates a tuple of strings. | ||
* T: The tuple of strings to concatenate. | ||
*/ | ||
type Concat<T extends string[]> = Join<T>; | ||
/** | ||
* A strongly-typed version of `String.prototype.concat`. | ||
* @param strings the tuple of strings to concatenate. | ||
* @returns the concatenated string in both type level and runtime. | ||
* @example concat('a', 'bc', 'def') // 'abcdef' | ||
*/ | ||
declare function concat<T extends string[]>(...strings: T): Concat<T>; | ||
/** | ||
* Gets the length of a string. | ||
*/ | ||
type Length<T extends string> = Split<T>['length']; | ||
/** | ||
* A strongly-typed version of `String.prototype.length`. | ||
* @param str the string to get the length from. | ||
* @returns the length of the string in both type level and runtime. | ||
* @example length('hello world') // 11 | ||
*/ | ||
declare function length<T extends string>(str: T): Length<T>; | ||
/** | ||
* PascalCases all the words in a tuple of strings | ||
@@ -11,6 +84,6 @@ */ | ||
*/ | ||
type Filter<tuple, cond, output extends any[] = []> = tuple extends [ | ||
type Reject<tuple, cond, output extends any[] = []> = tuple extends [ | ||
infer first, | ||
...infer rest | ||
] ? Filter<rest, cond, first extends cond ? output : [...output, first]> : output; | ||
] ? Reject<rest, cond, first extends cond ? output : [...output, first]> : output; | ||
/** | ||
@@ -33,28 +106,19 @@ * Removes the given suffix from a sentence. | ||
/** | ||
* Gets the character at the given index. | ||
* T: The string to get the character from. | ||
* index: The index of the character. | ||
* Slices a string from a startIndex to an endIndex. | ||
* T: The string to slice. | ||
* startIndex: The start index. | ||
* endIndex: The end index. | ||
*/ | ||
type CharAt<T extends string, index extends number> = Split<T>[index]; | ||
type Slice<T extends string, startIndex extends number = 0, endIndex extends number = Length<T>> = T extends `${infer head}${infer rest}` ? startIndex extends 0 ? endIndex extends 0 ? '' : `${head}${Slice<rest, Math.Subtract<Math.GetPositiveIndex<T, startIndex>, 1>, Math.Subtract<Math.GetPositiveIndex<T, endIndex>, 1>>}` : `${Slice<rest, Math.Subtract<Math.GetPositiveIndex<T, startIndex>, 1>, Math.Subtract<Math.GetPositiveIndex<T, endIndex>, 1>>}` : ''; | ||
/** | ||
* A strongly-typed version of `String.prototype.charAt`. | ||
* @param str the string to get the character from. | ||
* @param index the index of the character. | ||
* @returns the character in both type level and runtime. | ||
* @example charAt('hello world', 6) // 'w' | ||
* A strongly-typed version of `String.prototype.slice`. | ||
* @param str the string to slice. | ||
* @param start the start index. | ||
* @param end the end index. | ||
* @returns the sliced string in both type level and runtime. | ||
* @example slice('hello world', 6) // 'world' | ||
*/ | ||
declare function charAt<T extends string, I extends number>(str: T, index: I): CharAt<T, I>; | ||
declare function slice<T extends string, S extends number = 0, E extends number = Length<T>>(str: T, start?: S, end?: E): Slice<T, S, E>; | ||
/** | ||
* Concatenates a tuple of strings. | ||
* T: The tuple of strings to concatenate. | ||
*/ | ||
type Concat<T extends string[]> = Join<T>; | ||
/** | ||
* A strongly-typed version of `String.prototype.concat`. | ||
* @param strings the tuple of strings to concatenate. | ||
* @returns the concatenated string in both type level and runtime. | ||
* @example concat('a', 'bc', 'def') // 'abcdef' | ||
*/ | ||
declare function concat<T extends string[]>(...strings: T): Concat<T>; | ||
/** | ||
* Checks if a string ends with another string. | ||
@@ -75,30 +139,35 @@ * T: The string to check. | ||
declare function endsWith<T extends string, S extends string, P extends number = Length<T>>(text: T, search: S, position?: P): EndsWith<T, S, P>; | ||
/** | ||
* Joins a tuple of strings with the given delimiter. | ||
* T: The tuple of strings to join. | ||
* delimiter: The delimiter. | ||
* Checks if a string includes another string. | ||
* T: The string to check. | ||
* S: The string to check against. | ||
* P: The position to start the search. | ||
*/ | ||
type Join<T extends readonly string[], delimiter extends string = ''> = string[] extends T ? string : T extends readonly [ | ||
infer first extends string, | ||
...infer rest extends string[] | ||
] ? rest extends [] ? first : `${first}${delimiter}${Join<rest, delimiter>}` : ''; | ||
type Includes<T extends string, S extends string, P extends number = 0> = Math.IsNegative<P> extends false ? P extends 0 ? T extends `${string}${S}${string}` ? true : false : Includes<Slice<T, P>, S, 0> : Includes<T, S, 0>; | ||
/** | ||
* A strongly-typed version of `Array.prototype.join`. | ||
* @param tuple the tuple of strings to join. | ||
* @param delimiter the delimiter. | ||
* @returns the joined string in both type level and runtime. | ||
* @example join(['hello', 'world'], '-') // 'hello-world' | ||
* A strongly-typed version of `String.prototype.includes`. | ||
* @param text the string to search | ||
* @param search the string to search with | ||
* @param position the index to start search at | ||
* @returns boolean, whether or not the text contains the search string. | ||
* @example includes('abcde', 'bcd') // true | ||
*/ | ||
declare function join<const T extends readonly string[], D extends string = ''>(tuple: T, delimiter?: D): Join<T, D>; | ||
declare function includes<T extends string, S extends string, P extends number = 0>(text: T, search: S, position?: P): Includes<T, S, P>; | ||
/** | ||
* Gets the length of a string. | ||
* Repeats a string N times. | ||
* T: The string to repeat. | ||
* N: The number of times to repeat. | ||
*/ | ||
type Length<T extends string> = Split<T>['length']; | ||
type Repeat<T extends string, times extends number = 0> = times extends 0 ? '' : Math.IsNegative<times> extends false ? Join<TupleOf<times, T>> : never; | ||
/** | ||
* A strongly-typed version of `String.prototype.length`. | ||
* @param str the string to get the length from. | ||
* @returns the length of the string in both type level and runtime. | ||
* @example length('hello world') // 11 | ||
* A strongly-typed version of `String.prototype.repeat`. | ||
* @param str the string to repeat. | ||
* @param times the number of times to repeat. | ||
* @returns the repeated string in both type level and runtime. | ||
* @example repeat('hello', 3) // 'hellohellohello' | ||
*/ | ||
declare function length<T extends string>(str: T): Length<T>; | ||
declare function repeat<T extends string, N extends number = 0>(str: T, times?: N): Repeat<T, N>; | ||
/** | ||
@@ -120,2 +189,3 @@ * Pads a string at the end with another string. | ||
declare function padEnd<T extends string, N extends number = 0, U extends string = ' '>(str: T, length?: N, pad?: U): PadEnd<T, N, U>; | ||
/** | ||
@@ -137,17 +207,4 @@ * Pads a string at the start with another string. | ||
declare function padStart<T extends string, N extends number = 0, U extends string = ' '>(str: T, length?: N, pad?: U): PadStart<T, N, U>; | ||
/** | ||
* Repeats a string N times. | ||
* T: The string to repeat. | ||
* N: The number of times to repeat. | ||
*/ | ||
type Repeat<T extends string, times extends number = 0> = times extends 0 ? '' : Math.IsNegative<times> extends false ? Join<TupleOf<times, T>> : never; | ||
/** | ||
* A strongly-typed version of `String.prototype.repeat`. | ||
* @param str the string to repeat. | ||
* @param times the number of times to repeat. | ||
* @returns the repeated string in both type level and runtime. | ||
* @example repeat('hello', 3) // 'hellohellohello' | ||
*/ | ||
declare function repeat<T extends string, N extends number = 0>(str: T, times?: N): Repeat<T, N>; | ||
/** | ||
* Replaces the first occurrence of a string with another string. | ||
@@ -168,2 +225,3 @@ * sentence: The sentence to replace. | ||
declare function replace<T extends string, S extends string | RegExp, R extends string = ''>(sentence: T, lookup: S, replacement?: R): Replace<T, S, R>; | ||
/** | ||
@@ -185,33 +243,4 @@ * Replaces all the occurrences of a string with another string. | ||
declare function replaceAll<T extends string, S extends string | RegExp, R extends string = ''>(sentence: T, lookup: S, replacement?: R): ReplaceAll<T, S, R>; | ||
/** | ||
* Slices a string from a startIndex to an endIndex. | ||
* T: The string to slice. | ||
* startIndex: The start index. | ||
* endIndex: The end index. | ||
*/ | ||
type Slice<T extends string, startIndex extends number = 0, endIndex extends number = Length<T>> = T extends `${infer head}${infer rest}` ? startIndex extends 0 ? endIndex extends 0 ? '' : `${head}${Slice<rest, Math.Subtract<Math.GetPositiveIndex<T, startIndex>, 1>, Math.Subtract<Math.GetPositiveIndex<T, endIndex>, 1>>}` : `${Slice<rest, Math.Subtract<Math.GetPositiveIndex<T, startIndex>, 1>, Math.Subtract<Math.GetPositiveIndex<T, endIndex>, 1>>}` : ''; | ||
/** | ||
* A strongly-typed version of `String.prototype.slice`. | ||
* @param str the string to slice. | ||
* @param start the start index. | ||
* @param end the end index. | ||
* @returns the sliced string in both type level and runtime. | ||
* @example slice('hello world', 6) // 'world' | ||
*/ | ||
declare function slice<T extends string, S extends number = 0, E extends number = Length<T>>(str: T, start?: S, end?: E): Slice<T, S, E>; | ||
/** | ||
* Splits a string into an array of substrings. | ||
* T: The string to split. | ||
* delimiter: The delimiter. | ||
*/ | ||
type Split<T, delimiter extends string = ''> = T extends `${infer first}${delimiter}${infer rest}` ? [first, ...Split<rest, delimiter>] : T extends '' ? [] : [T]; | ||
/** | ||
* A strongly-typed version of `String.prototype.split`. | ||
* @param str the string to split. | ||
* @param delimiter the delimiter. | ||
* @returns the splitted string in both type level and runtime. | ||
* @example split('hello world', ' ') // ['hello', 'world'] | ||
*/ | ||
declare function split<T extends string, D extends string = ''>(str: T, delimiter?: D): Split<T, D>; | ||
/** | ||
* Checks if a string starts with another string. | ||
@@ -232,19 +261,4 @@ * T: The string to check. | ||
declare function startsWith<T extends string, S extends string, P extends number = 0>(text: T, search: S, position?: P): StartsWith<T, S, P>; | ||
/** | ||
* Checks if a string includes another string. | ||
* T: The string to check. | ||
* S: The string to check against. | ||
* P: The position to start the search. | ||
*/ | ||
type Includes<T extends string, S extends string, P extends number = 0> = Math.IsNegative<P> extends false ? P extends 0 ? T extends `${string}${S}${string}` ? true : false : Includes<Slice<T, P>, S, 0> : Includes<T, S, 0>; | ||
/** | ||
* A strongly-typed version of `String.prototype.includes`. | ||
* @param text the string to search | ||
* @param search the string to search with | ||
* @param position the index to start search at | ||
* @returns boolean, whether or not the text contains the search string. | ||
* @example includes('abcde', 'bcd') // true | ||
*/ | ||
declare function includes<T extends string, S extends string, P extends number = 0>(text: T, search: S, position?: P): Includes<T, S, P>; | ||
/** | ||
* Trims all whitespaces at the start of a string. | ||
@@ -261,2 +275,3 @@ * T: The string to trim. | ||
declare function trimStart<T extends string>(str: T): TrimStart<T>; | ||
/** | ||
@@ -274,2 +289,3 @@ * Trims all whitespaces at the end of a string. | ||
declare function trimEnd<T extends string>(str: T): TrimEnd<T>; | ||
/** | ||
@@ -280,10 +296,48 @@ * Trims all whitespaces at the start and end of a string. | ||
type Trim<T extends string> = TrimEnd<TrimStart<T>>; | ||
/** | ||
* A strongly-typed version of `String.prototype.trim`. | ||
* @param str the string to trim. | ||
* @returns the trimmed string in both type level and runtime. | ||
* @example trim(' hello world ') // 'hello world' | ||
* This function is a strongly-typed counterpart of String.prototype.toLowerCase. | ||
* @param str the string to make lowercase. | ||
* @returns the lowercased string. | ||
* @example toLowerCase('HELLO WORLD') // 'hello world' | ||
*/ | ||
declare function trim<T extends string>(str: T): TrimEnd<TrimStart<T>>; | ||
declare function toLowerCase<T extends string>(str: T): Lowercase<T>; | ||
/** | ||
* This function is a strongly-typed counterpart of String.prototype.toUpperCase. | ||
* @param str the string to make uppercase. | ||
* @returns the uppercased string. | ||
* @example toUpperCase('hello world') // 'HELLO WORLD' | ||
*/ | ||
declare function toUpperCase<T extends string>(str: T): Uppercase<T>; | ||
/** | ||
* Reverses a string. | ||
* - `T` The string to reverse. | ||
*/ | ||
type Reverse<T extends string> = T extends `${infer Head}${infer Tail}` ? `${Reverse<Tail>}${Head}` : T; | ||
/** | ||
* A strongly-typed function to reverse a string. | ||
* @param str the string to reverse. | ||
* @returns the reversed string in both type level and runtime. | ||
* @example reverse('hello world') // 'dlrow olleh' | ||
*/ | ||
declare function reverse<T extends string>(str: T): Reverse<T>; | ||
/** | ||
* Truncate a string if it's longer than the given maximum length. | ||
* The last characters of the truncated string are replaced with the omission string which defaults to "...". | ||
*/ | ||
type Truncate<T extends string, Size extends number, Omission extends string = '...'> = Math.IsNegative<Size> extends true ? Omission : Math.Subtract<Length<T>, Size> extends 0 ? T : Join<[Slice<T, 0, Math.Subtract<Size, Length<Omission>>>, Omission]>; | ||
/** | ||
* A strongly typed function to truncate a string if it's longer than the given maximum string length. | ||
* The last characters of the truncated string are replaced with the omission string which defaults to "...". | ||
* @param sentence the sentence to extract the words from. | ||
* @param length the maximum length of the string. | ||
* @param omission the string to append to the end of the truncated string. | ||
* @returns the truncated string | ||
* @example truncate('Hello, World', 8) // 'Hello...' | ||
*/ | ||
declare function truncate<T extends string, S extends number, P extends string = '...'>(sentence: T, length: S, omission?: P): Truncate<T, S, P>; | ||
declare const SEPARATORS: readonly ["[", "]", "{", "}", "(", ")", "|", "/", "-", "\\", " ", "_", "."]; | ||
@@ -297,3 +351,2 @@ type Separator = (typeof SEPARATORS)[number]; | ||
type Digit = '0' | '1' | '2' | '3' | '4' | '5' | '6' | '7' | '8' | '9'; | ||
type UpperChars = 'A' | 'B' | 'C' | 'D' | 'E' | 'F' | 'G' | 'H' | 'I' | 'J' | 'K' | 'L' | 'M' | 'N' | 'O' | 'P' | 'Q' | 'R' | 'S' | 'T' | 'U' | 'V' | 'W' | 'X' | 'Y' | 'Z'; | ||
@@ -306,13 +359,16 @@ type LowerChars = Lowercase<UpperChars>; | ||
/** | ||
* Checks if the given character is a lower case letter. | ||
*/ | ||
type IsLower<T extends string> = T extends LowerChars ? true : false; | ||
/** | ||
* Checks if the given character is a letter. | ||
*/ | ||
type IsLetter<T extends string> = IsUpper<T> extends true ? true : IsLower<T> extends true ? true : false; | ||
type Digit = '0' | '1' | '2' | '3' | '4' | '5' | '6' | '7' | '8' | '9'; | ||
/** | ||
* Checks if the given character is a lower case letter. | ||
*/ | ||
type IsLower<T extends string> = T extends LowerChars ? true : false; | ||
/** | ||
* Checks if the given character is a number. | ||
*/ | ||
type IsDigit<T extends string> = T extends Digit ? true : false; | ||
/** | ||
@@ -330,3 +386,3 @@ * Checks if the given character is a special character. | ||
*/ | ||
type Words<sentence extends string, word extends string = '', prev extends string = ''> = string extends sentence ? string[] : sentence extends `${infer curr}${infer rest}` ? IsSeparator<curr> extends true ? Filter<[word, ...Words<rest>], ''> : prev extends '' ? Filter<Words<rest, curr, curr>, ''> : [false, true] extends [IsDigit<prev>, IsDigit<curr>] ? [ | ||
type Words<sentence extends string, word extends string = '', prev extends string = ''> = string extends sentence ? string[] : sentence extends `${infer curr}${infer rest}` ? IsSeparator<curr> extends true ? Reject<[word, ...Words<rest>], ''> : prev extends '' ? Reject<Words<rest, curr, curr>, ''> : [false, true] extends [IsDigit<prev>, IsDigit<curr>] ? [ | ||
word, | ||
@@ -343,3 +399,3 @@ ...Words<rest, curr, curr> | ||
...Words<rest, curr, curr> | ||
] : [true, true] extends [IsDigit<prev>, IsDigit<curr>] ? Filter<Words<rest, `${word}${curr}`, curr>, ''> : [true, true] extends [IsLower<prev>, IsUpper<curr>] ? [ | ||
] : [true, true] extends [IsDigit<prev>, IsDigit<curr>] ? Reject<Words<rest, `${word}${curr}`, curr>, ''> : [true, true] extends [IsLower<prev>, IsUpper<curr>] ? [ | ||
word, | ||
@@ -350,3 +406,3 @@ ...Words<rest, curr, curr> | ||
...Words<rest, `${prev}${curr}`, curr> | ||
] : Filter<Words<rest, `${word}${curr}`, curr>, ''> : Filter<[word], ''>; | ||
] : Reject<Words<rest, `${word}${curr}`, curr>, ''> : Reject<[word], ''>; | ||
/** | ||
@@ -359,46 +415,39 @@ * A strongly typed function to extract the words from a sentence. | ||
declare function words<T extends string>(sentence: T): Words<T>; | ||
/** | ||
* Truncate a string if it's longer than the given maximum length. | ||
* The last characters of the truncated string are replaced with the omission string which defaults to "...". | ||
* Transforms a string to PascalCase. | ||
*/ | ||
type Truncate<T extends string, Size extends number, Omission extends string = '...'> = Math.IsNegative<Size> extends true ? Omission : Math.Subtract<Length<T>, Size> extends 0 ? T : Join<[Slice<T, 0, Math.Subtract<Size, Length<Omission>>>, Omission]>; | ||
type PascalCase<T extends string> = Join<PascalCaseAll<Words<T>>>; | ||
/** | ||
* A strongly typed function to truncate a string if it's longer than the given maximum string length. | ||
* The last characters of the truncated string are replaced with the omission string which defaults to "...". | ||
* @param sentence the sentence to extract the words from. | ||
* @param length the maximum length of the string. | ||
* @param omission the string to append to the end of the truncated string. | ||
* @returns the truncated string | ||
* @example truncate('Hello, World', 8) // 'Hello...' | ||
* A strongly typed version of `pascalCase` that works in both runtime and type level. | ||
* @param str the string to convert to pascal case. | ||
* @returns the pascal cased string. | ||
* @example pascalCase('hello world') // 'HelloWorld' | ||
*/ | ||
declare function truncate<T extends string, S extends number, P extends string = '...'>(sentence: T, length: S, omission?: P): Truncate<T, S, P>; | ||
declare function pascalCase<T extends string>(str: T): PascalCase<T>; | ||
/** | ||
* Capitalizes the first letter of a string. This is a runtime counterpart of `Capitalize<T>` from `src/types.d.ts`. | ||
* @param str the string to capitalize. | ||
* @returns the capitalized string. | ||
* @example capitalize('hello world') // 'Hello world' | ||
* @deprecated | ||
* Use `pascalCase` instead. | ||
* Read more about the deprecation [here](https://github.com/gustavoguichard/string-ts/issues/44). | ||
*/ | ||
declare function capitalize<T extends string>(str: T): Capitalize<T>; | ||
declare const toPascalCase: typeof pascalCase; | ||
/** | ||
* This function is a strongly-typed counterpart of String.prototype.toLowerCase. | ||
* @param str the string to make lowercase. | ||
* @returns the lowercased string. | ||
* @example toLowerCase('HELLO WORLD') // 'hello world' | ||
* Transforms a string to camelCase. | ||
*/ | ||
declare function toLowerCase<T extends string>(str: T): Lowercase<T>; | ||
type CamelCase<T extends string> = Uncapitalize<PascalCase<T>>; | ||
/** | ||
* This function is a strongly-typed counterpart of String.prototype.toUpperCase. | ||
* @param str the string to make uppercase. | ||
* @returns the uppercased string. | ||
* @example toUpperCase('hello world') // 'HELLO WORLD' | ||
* A strongly typed version of `camelCase` that works in both runtime and type level. | ||
* @param str the string to convert to camel case. | ||
* @returns the camel cased string. | ||
* @example camelCase('hello world') // 'helloWorld' | ||
*/ | ||
declare function toUpperCase<T extends string>(str: T): Uppercase<T>; | ||
declare function camelCase<T extends string>(str: T): CamelCase<T>; | ||
/** | ||
* Uncapitalizes the first letter of a string. This is a runtime counterpart of `Uncapitalize<T>` from `src/types.d.ts`. | ||
* @param str the string to uncapitalize. | ||
* @returns the uncapitalized string. | ||
* @example uncapitalize('Hello world') // 'hello world' | ||
* @deprecated | ||
* Use `camelCase` instead. | ||
* Read more about the deprecation [here](https://github.com/gustavoguichard/string-ts/issues/44). | ||
*/ | ||
declare function uncapitalize<T extends string>(str: T): Uncapitalize<T>; | ||
declare const toCamelCase: typeof camelCase; | ||
/** | ||
@@ -413,27 +462,30 @@ * Transforms a string with the specified separator (delimiter). | ||
* @returns the transformed string. | ||
* @example toDelimiterCase('hello world', '.') // 'hello.world' | ||
* @example delimiterCase('hello world', '.') // 'hello.world' | ||
*/ | ||
declare function toDelimiterCase<T extends string, D extends string>(str: T, delimiter: D): DelimiterCase<T, D>; | ||
declare function delimiterCase<T extends string, D extends string>(str: T, delimiter: D): DelimiterCase<T, D>; | ||
/** | ||
* Transforms a string to camelCase. | ||
* @deprecated | ||
* Use `delimiterCase` instead. | ||
* Read more about the deprecation [here](https://github.com/gustavoguichard/string-ts/issues/44). | ||
*/ | ||
type CamelCase<T extends string> = Uncapitalize<PascalCase<T>>; | ||
declare const toDelimiterCase: typeof delimiterCase; | ||
/** | ||
* A strongly typed version of `toCamelCase` that works in both runtime and type level. | ||
* @param str the string to convert to camel case. | ||
* @returns the camel cased string. | ||
* @example toCamelCase('hello world') // 'helloWorld' | ||
* Transforms a string to CONSTANT_CASE. | ||
*/ | ||
declare function toCamelCase<T extends string>(str: T): CamelCase<T>; | ||
type ConstantCase<T extends string> = Uppercase<DelimiterCase<T, '_'>>; | ||
/** | ||
* Transforms a string to PascalCase. | ||
* A strongly typed version of `constantCase` that works in both runtime and type level. | ||
* @param str the string to convert to constant case. | ||
* @returns the constant cased string. | ||
* @example constantCase('hello world') // 'HELLO_WORLD' | ||
*/ | ||
type PascalCase<T extends string> = Join<PascalCaseAll<Words<T>>>; | ||
declare function constantCase<T extends string>(str: T): ConstantCase<T>; | ||
/** | ||
* A strongly typed version of `toPascalCase` that works in both runtime and type level. | ||
* @param str the string to convert to pascal case. | ||
* @returns the pascal cased string. | ||
* @example toPascalCase('hello world') // 'HelloWorld' | ||
* @deprecated | ||
* Use `constantCase` instead. | ||
* Read more about the deprecation [here](https://github.com/gustavoguichard/string-ts/issues/44). | ||
*/ | ||
declare function toPascalCase<T extends string>(str: T): PascalCase<T>; | ||
declare const toConstantCase: typeof constantCase; | ||
/** | ||
@@ -444,9 +496,16 @@ * Transforms a string to kebab-case. | ||
/** | ||
* A strongly typed version of `toKebabCase` that works in both runtime and type level. | ||
* A strongly typed version of `kebabCase` that works in both runtime and type level. | ||
* @param str the string to convert to kebab case. | ||
* @returns the kebab cased string. | ||
* @example toKebabCase('hello world') // 'hello-world' | ||
* @example kebabCase('hello world') // 'hello-world' | ||
*/ | ||
declare function toKebabCase<T extends string>(str: T): KebabCase<T>; | ||
declare function kebabCase<T extends string>(str: T): KebabCase<T>; | ||
/** | ||
* @deprecated | ||
* Use `kebabCase` instead. | ||
* Read more about the deprecation [here](https://github.com/gustavoguichard/string-ts/issues/44). | ||
*/ | ||
declare const toKebabCase: typeof kebabCase; | ||
/** | ||
* Transforms a string to snake_case. | ||
@@ -456,20 +515,16 @@ */ | ||
/** | ||
* A strongly typed version of `toSnakeCase` that works in both runtime and type level. | ||
* A strongly typed version of `snakeCase` that works in both runtime and type level. | ||
* @param str the string to convert to snake case. | ||
* @returns the snake cased string. | ||
* @example toSnakeCase('hello world') // 'hello_world' | ||
* @example snakeCase('hello world') // 'hello_world' | ||
*/ | ||
declare function toSnakeCase<T extends string>(str: T): SnakeCase<T>; | ||
declare function snakeCase<T extends string>(str: T): SnakeCase<T>; | ||
/** | ||
* Transforms a string to CONSTANT_CASE. | ||
* @deprecated | ||
* Use `snakeCase` instead. | ||
* Read more about the deprecation [here](https://github.com/gustavoguichard/string-ts/issues/44). | ||
*/ | ||
type ConstantCase<T extends string> = Uppercase<DelimiterCase<T, '_'>>; | ||
declare const toSnakeCase: typeof snakeCase; | ||
/** | ||
* A strongly typed version of `toConstantCase` that works in both runtime and type level. | ||
* @param str the string to convert to constant case. | ||
* @returns the constant cased string. | ||
* @example toConstantCase('hello world') // 'HELLO_WORLD' | ||
*/ | ||
declare function toConstantCase<T extends string>(str: T): ConstantCase<T>; | ||
/** | ||
* Transforms a string to "Title Case". | ||
@@ -479,10 +534,48 @@ */ | ||
/** | ||
* A strongly typed version of `toTitleCase` that works in both runtime and type level. | ||
* A strongly typed version of `titleCase` that works in both runtime and type level. | ||
* @param str the string to convert to title case. | ||
* @returns the title cased string. | ||
* @example toTitleCase('hello world') // 'Hello World' | ||
* @example titleCase('hello world') // 'Hello World' | ||
*/ | ||
declare function toTitleCase<T extends string>(str: T): TitleCase<T>; | ||
declare function titleCase<T extends string>(str: T): TitleCase<T>; | ||
/** | ||
* @deprecated | ||
* Use `titleCase` instead. | ||
* Read more about the deprecation [here](https://github.com/gustavoguichard/string-ts/issues/44). | ||
*/ | ||
declare const toTitleCase: typeof titleCase; | ||
/** | ||
* Capitalizes the first letter of a string. This is a runtime counterpart of `Capitalize<T>` from `src/types.d.ts`. | ||
* @param str the string to capitalize. | ||
* @returns the capitalized string. | ||
* @example capitalize('hello world') // 'Hello world' | ||
*/ | ||
declare function capitalize<T extends string>(str: T): Capitalize<T>; | ||
/** | ||
* A strongly-typed version of `lowerCase` that works in both runtime and type level. | ||
* @param str the string to convert to lower case. | ||
* @returns the lowercased string. | ||
* @example lowerCase('HELLO-WORLD') // 'hello world' | ||
*/ | ||
declare function lowerCase<T extends string>(str: T): Lowercase<DelimiterCase<T, ' '>>; | ||
/** | ||
* Uncapitalizes the first letter of a string. This is a runtime counterpart of `Uncapitalize<T>` from `src/types.d.ts`. | ||
* @param str the string to uncapitalize. | ||
* @returns the uncapitalized string. | ||
* @example uncapitalize('Hello world') // 'hello world' | ||
*/ | ||
declare function uncapitalize<T extends string>(str: T): Uncapitalize<T>; | ||
/** | ||
* A strongly-typed version of `upperCase` that works in both runtime and type level. | ||
* @param str the string to convert to upper case. | ||
* @returns the uppercased string. | ||
* @example upperCase('hello-world') // 'HELLO WORLD' | ||
*/ | ||
declare function upperCase<T extends string>(str: T): Uppercase<DelimiterCase<T, ' '>>; | ||
/** | ||
* Shallowly transforms the keys of an Record to camelCase. | ||
@@ -501,2 +594,3 @@ * T: the type of the Record to transform. | ||
declare function camelKeys<T>(obj: T): CamelKeys<T>; | ||
/** | ||
@@ -516,2 +610,3 @@ * Shallowly transforms the keys of an Record to CONSTANT_CASE. | ||
declare function constantKeys<T>(obj: T): ConstantKeys<T>; | ||
/** | ||
@@ -533,2 +628,3 @@ * Shallowly transforms the keys of an Record to a custom delimiter case. | ||
declare function delimiterKeys<T, D extends string>(obj: T, delimiter: D): DelimiterKeys<T, D>; | ||
/** | ||
@@ -548,2 +644,3 @@ * Shallowly transforms the keys of an Record to kebab-case. | ||
declare function kebabKeys<T>(obj: T): KebabKeys<T>; | ||
/** | ||
@@ -563,2 +660,3 @@ * Shallowly transforms the keys of an Record to PascalCase. | ||
declare function pascalKeys<T>(obj: T): PascalKeys<T>; | ||
/** | ||
@@ -580,12 +678,2 @@ * Shallowly transforms the keys of an Record to snake_case. | ||
/** | ||
* This function is used to transform the keys of an object deeply. | ||
* It will only be transformed at runtime, so it's not type safe. | ||
* @param obj the object to transform. | ||
* @param transform the function to transform the keys from string to string. | ||
* @returns the transformed object. | ||
* @example deepTransformKeys({ 'foo-bar': { 'fizz-buzz': true } }, toCamelCase) | ||
* // { fooBar: { fizzBuzz: true } } | ||
*/ | ||
declare function deepTransformKeys<T>(obj: T, transform: (s: string) => string): T; | ||
/** | ||
* Recursively transforms the keys of an Record to camelCase. | ||
@@ -606,2 +694,3 @@ * T: the type of the Record to transform. | ||
declare function deepCamelKeys<T>(obj: T): DeepCamelKeys<T>; | ||
/** | ||
@@ -623,2 +712,3 @@ * Recursively transforms the keys of an Record to CONSTANT_CASE. | ||
declare function deepConstantKeys<T>(obj: T): DeepConstantKeys<T>; | ||
/** | ||
@@ -642,2 +732,3 @@ * Recursively transforms the keys of an Record to a custom delimiter case. | ||
declare function deepDelimiterKeys<T, D extends string>(obj: T, delimiter: D): DeepDelimiterKeys<T, D>; | ||
/** | ||
@@ -659,2 +750,3 @@ * Recursively transforms the keys of an Record to kebab-case. | ||
declare function deepKebabKeys<T>(obj: T): DeepKebabKeys<T>; | ||
/** | ||
@@ -676,2 +768,3 @@ * Recursively transforms the keys of an Record to PascalCase. | ||
declare function deepPascalKeys<T>(obj: T): DeepPascalKeys<T>; | ||
/** | ||
@@ -694,2 +787,2 @@ * Recursively transforms the keys of an Record to snake_case. | ||
export { CamelCase, CamelKeys, CharAt, Concat, ConstantCase, ConstantKeys, DeepCamelKeys, DeepConstantKeys, DeepDelimiterKeys, DeepKebabKeys, DeepPascalKeys, DeepSnakeKeys, DelimiterCase, DelimiterKeys, Digit, EndsWith, Includes, IsDigit, IsLetter, IsLower, IsSeparator, IsSpecial, IsUpper, Join, KebabCase, KebabKeys, Length, PadEnd, PadStart, PascalCase, PascalKeys, Repeat, Replace, ReplaceAll, Separator, Slice, SnakeCase, SnakeKeys, Split, StartsWith, TitleCase, Trim, TrimEnd, TrimStart, Truncate, Words, camelKeys, capitalize, charAt, concat, constantKeys, deepCamelKeys, deepConstantKeys, deepDelimiterKeys, deepKebabKeys, deepPascalKeys, deepSnakeKeys, deepTransformKeys, delimiterKeys, endsWith, includes, join, kebabKeys, length, padEnd, padStart, pascalKeys, repeat, replace, replaceAll, slice, snakeKeys, split, startsWith, toCamelCase, toConstantCase, toDelimiterCase, toKebabCase, toLowerCase, toPascalCase, toSnakeCase, toTitleCase, toUpperCase, trim, trimEnd, trimStart, truncate, uncapitalize, words }; | ||
export { CamelCase, CamelKeys, CharAt, Concat, ConstantCase, ConstantKeys, DeepCamelKeys, DeepConstantKeys, DeepDelimiterKeys, DeepKebabKeys, DeepPascalKeys, DeepSnakeKeys, DelimiterCase, DelimiterKeys, Digit, EndsWith, Includes, IsDigit, IsLetter, IsLower, IsSeparator, IsSpecial, IsUpper, Join, KebabCase, KebabKeys, Length, PadEnd, PadStart, PascalCase, PascalKeys, Repeat, Replace, ReplaceAll, Reverse, Separator, Slice, SnakeCase, SnakeKeys, Split, StartsWith, TitleCase, Trim, TrimEnd, TrimStart, Truncate, Words, camelCase, camelKeys, capitalize, charAt, concat, constantCase, constantKeys, deepCamelKeys, deepConstantKeys, deepDelimiterKeys, deepKebabKeys, deepPascalKeys, deepSnakeKeys, delimiterCase, delimiterKeys, endsWith, includes, join, kebabCase, kebabKeys, length, lowerCase, padEnd, padStart, pascalCase, pascalKeys, repeat, replace, replaceAll, reverse, slice, snakeCase, snakeKeys, split, startsWith, titleCase, toCamelCase, toConstantCase, toDelimiterCase, toKebabCase, toLowerCase, toPascalCase, toSnakeCase, toTitleCase, toUpperCase, trimEnd, trimStart, truncate, uncapitalize, upperCase, words }; |
@@ -1,97 +0,54 @@ | ||
"use strict"; | ||
var __defProp = Object.defineProperty; | ||
var __getOwnPropDesc = Object.getOwnPropertyDescriptor; | ||
var __getOwnPropNames = Object.getOwnPropertyNames; | ||
var __hasOwnProp = Object.prototype.hasOwnProperty; | ||
var __export = (target, all) => { | ||
for (var name in all) | ||
__defProp(target, name, { get: all[name], enumerable: true }); | ||
}; | ||
var __copyProps = (to, from, except, desc) => { | ||
if (from && typeof from === "object" || typeof from === "function") { | ||
for (let key of __getOwnPropNames(from)) | ||
if (!__hasOwnProp.call(to, key) && key !== except) | ||
__defProp(to, key, { get: () => from[key], enumerable: !(desc = __getOwnPropDesc(from, key)) || desc.enumerable }); | ||
} | ||
return to; | ||
}; | ||
var __toCommonJS = (mod) => __copyProps(__defProp({}, "__esModule", { value: true }), mod); | ||
'use strict'; | ||
// src/index.ts | ||
var src_exports = {}; | ||
__export(src_exports, { | ||
camelKeys: () => camelKeys, | ||
capitalize: () => capitalize, | ||
charAt: () => charAt, | ||
concat: () => concat, | ||
constantKeys: () => constantKeys, | ||
deepCamelKeys: () => deepCamelKeys, | ||
deepConstantKeys: () => deepConstantKeys, | ||
deepDelimiterKeys: () => deepDelimiterKeys, | ||
deepKebabKeys: () => deepKebabKeys, | ||
deepPascalKeys: () => deepPascalKeys, | ||
deepSnakeKeys: () => deepSnakeKeys, | ||
deepTransformKeys: () => deepTransformKeys, | ||
delimiterKeys: () => delimiterKeys, | ||
endsWith: () => endsWith, | ||
includes: () => includes, | ||
join: () => join, | ||
kebabKeys: () => kebabKeys, | ||
length: () => length, | ||
padEnd: () => padEnd, | ||
padStart: () => padStart, | ||
pascalKeys: () => pascalKeys, | ||
repeat: () => repeat, | ||
replace: () => replace, | ||
replaceAll: () => replaceAll, | ||
slice: () => slice, | ||
snakeKeys: () => snakeKeys, | ||
split: () => split, | ||
startsWith: () => startsWith, | ||
toCamelCase: () => toCamelCase, | ||
toConstantCase: () => toConstantCase, | ||
toDelimiterCase: () => toDelimiterCase, | ||
toKebabCase: () => toKebabCase, | ||
toLowerCase: () => toLowerCase, | ||
toPascalCase: () => toPascalCase, | ||
toSnakeCase: () => toSnakeCase, | ||
toTitleCase: () => toTitleCase, | ||
toUpperCase: () => toUpperCase, | ||
trim: () => trim, | ||
trimEnd: () => trimEnd, | ||
trimStart: () => trimStart, | ||
truncate: () => truncate, | ||
uncapitalize: () => uncapitalize, | ||
words: () => words | ||
}); | ||
module.exports = __toCommonJS(src_exports); | ||
// src/primitives.ts | ||
// src/native/char-at.ts | ||
function charAt(str, index) { | ||
return str.charAt(index); | ||
} | ||
// src/native/join.ts | ||
function join(tuple, delimiter = "") { | ||
return tuple.join(delimiter); | ||
} | ||
// src/native/concat.ts | ||
function concat(...strings) { | ||
return join(strings); | ||
} | ||
// src/native/ends-with.ts | ||
function endsWith(text, search, position = text.length) { | ||
return text.endsWith(search, position); | ||
} | ||
function join(tuple, delimiter = "") { | ||
return tuple.join(delimiter); | ||
// src/native/includes.ts | ||
function includes(text, search, position = 0) { | ||
return text.includes(search, position); | ||
} | ||
// src/native/length.ts | ||
function length(str) { | ||
return str.length; | ||
} | ||
// src/native/pad-end.ts | ||
function padEnd(str, length2 = 0, pad = " ") { | ||
return str.padEnd(length2, pad); | ||
} | ||
// src/native/pad-start.ts | ||
function padStart(str, length2 = 0, pad = " ") { | ||
return str.padStart(length2, pad); | ||
} | ||
// src/native/repeat.ts | ||
function repeat(str, times = 0) { | ||
return str.repeat(times); | ||
} | ||
// src/native/replace.ts | ||
function replace(sentence, lookup, replacement = "") { | ||
return sentence.replace(lookup, replacement); | ||
} | ||
// src/native/replace-all.ts | ||
function replaceAll(sentence, lookup, replacement = "") { | ||
@@ -104,25 +61,53 @@ if (typeof sentence.replaceAll === "function") { | ||
} | ||
// src/native/slice.ts | ||
function slice(str, start = 0, end = str.length) { | ||
return str.slice(start, end); | ||
} | ||
// src/native/split.ts | ||
function split(str, delimiter = "") { | ||
return str.split(delimiter); | ||
} | ||
// src/native/starts-with.ts | ||
function startsWith(text, search, position = 0) { | ||
return text.startsWith(search, position); | ||
} | ||
function includes(text, search, position = 0) { | ||
return text.includes(search, position); | ||
// src/native/to-lower-case.ts | ||
function toLowerCase(str) { | ||
return str.toLowerCase(); | ||
} | ||
// src/native/to-upper-case.ts | ||
function toUpperCase(str) { | ||
return str.toUpperCase(); | ||
} | ||
// src/native/trim-start.ts | ||
function trimStart(str) { | ||
return str.trimStart(); | ||
} | ||
// src/native/trim-end.ts | ||
function trimEnd(str) { | ||
return str.trimEnd(); | ||
} | ||
function trim(str) { | ||
return str.trim(); | ||
// src/utils/reverse.ts | ||
function reverse(str) { | ||
return str.split("").reverse().join(""); | ||
} | ||
// src/separators.ts | ||
// src/utils/truncate.ts | ||
function truncate(sentence, length2, omission = "...") { | ||
if (length2 < 0) | ||
return omission; | ||
if (sentence.length <= length2) | ||
return sentence; | ||
return join([sentence.slice(0, length2 - omission.length), omission]); | ||
} | ||
// src/utils/characters/separators.ts | ||
var UNESCAPED_SEPARATORS = [ | ||
@@ -149,15 +134,24 @@ "[", | ||
// src/utils.ts | ||
// src/utils/words.ts | ||
function words(sentence) { | ||
return sentence.replace(SEPARATOR_REGEX, " ").replace(/([a-zA-Z])([0-9])/g, "$1 $2").replace(/([0-9])([a-zA-Z])/g, "$1 $2").replace(/([a-zA-Z0-9_\-./])([^a-zA-Z0-9_\-./])/g, "$1 $2").replace(/([^a-zA-Z0-9_\-./])([a-zA-Z0-9_\-./])/g, "$1 $2").replace(/([a-z])([A-Z])/g, "$1 $2").replace(/([A-Z])([A-Z][a-z])/g, "$1 $2").trim().split(/\s+/g); | ||
} | ||
function truncate(sentence, length2, omission = "...") { | ||
if (length2 < 0) | ||
return omission; | ||
if (sentence.length <= length2) | ||
return sentence; | ||
return join([sentence.slice(0, length2 - omission.length), omission]); | ||
// src/utils/capitalize.ts | ||
function capitalize(str) { | ||
return join([toUpperCase(charAt(str, 0)), slice(str, 1)]); | ||
} | ||
// src/internals.ts | ||
// src/utils/word-case/delimiter-case.ts | ||
function delimiterCase(str, delimiter) { | ||
return join(words(str), delimiter); | ||
} | ||
var toDelimiterCase = delimiterCase; | ||
// src/utils/word-case/lower-case.ts | ||
function lowerCase(str) { | ||
return toLowerCase(delimiterCase(str, " ")); | ||
} | ||
// src/internal/internals.ts | ||
function typeOf(t) { | ||
@@ -170,38 +164,49 @@ return Object.prototype.toString.call(t).replace(/^\[object (.+)\]$/, "$1").toLowerCase(); | ||
// src/casing.ts | ||
function capitalize(str) { | ||
return join([toUpperCase(charAt(str, 0)), slice(str, 1)]); | ||
// src/utils/word-case/pascal-case.ts | ||
function pascalCase(str) { | ||
return join(pascalCaseAll(words(str))); | ||
} | ||
function toLowerCase(str) { | ||
return str.toLowerCase(); | ||
} | ||
function toUpperCase(str) { | ||
return str.toUpperCase(); | ||
} | ||
var toPascalCase = pascalCase; | ||
// src/utils/uncapitalize.ts | ||
function uncapitalize(str) { | ||
return join([toLowerCase(charAt(str, 0)), slice(str, 1)]); | ||
} | ||
function toDelimiterCase(str, delimiter) { | ||
return join(words(str), delimiter); | ||
// src/utils/word-case/camel-case.ts | ||
function camelCase(str) { | ||
return uncapitalize(pascalCase(str)); | ||
} | ||
function toCamelCase(str) { | ||
return uncapitalize(toPascalCase(str)); | ||
var toCamelCase = camelCase; | ||
// src/utils/word-case/constant-case.ts | ||
function constantCase(str) { | ||
return toUpperCase(delimiterCase(str, "_")); | ||
} | ||
function toPascalCase(str) { | ||
return join(pascalCaseAll(words(str))); | ||
var toConstantCase = constantCase; | ||
// src/utils/word-case/kebab-case.ts | ||
function kebabCase(str) { | ||
return toLowerCase(delimiterCase(str, "-")); | ||
} | ||
function toKebabCase(str) { | ||
return toLowerCase(toDelimiterCase(str, "-")); | ||
var toKebabCase = kebabCase; | ||
// src/utils/word-case/snake-case.ts | ||
function snakeCase(str) { | ||
return toLowerCase(delimiterCase(str, "_")); | ||
} | ||
function toSnakeCase(str) { | ||
return toLowerCase(toDelimiterCase(str, "_")); | ||
var toSnakeCase = snakeCase; | ||
// src/utils/word-case/title-case.ts | ||
function titleCase(str) { | ||
return delimiterCase(pascalCase(str), " "); | ||
} | ||
function toConstantCase(str) { | ||
return toUpperCase(toDelimiterCase(str, "_")); | ||
var toTitleCase = titleCase; | ||
// src/utils/word-case/upper-case.ts | ||
function upperCase(str) { | ||
return toUpperCase(delimiterCase(str, " ")); | ||
} | ||
function toTitleCase(str) { | ||
return toDelimiterCase(toPascalCase(str), " "); | ||
} | ||
// src/key-casing.ts | ||
// src/utils/object-keys/transform-keys.ts | ||
function transformKeys(obj, transform) { | ||
@@ -216,22 +221,34 @@ if (typeOf(obj) !== "object") | ||
} | ||
// src/utils/object-keys/camel-keys.ts | ||
function camelKeys(obj) { | ||
return transformKeys(obj, toCamelCase); | ||
return transformKeys(obj, camelCase); | ||
} | ||
// src/utils/object-keys/constant-keys.ts | ||
function constantKeys(obj) { | ||
return transformKeys(obj, toConstantCase); | ||
return transformKeys(obj, constantCase); | ||
} | ||
// src/utils/object-keys/delimiter-keys.ts | ||
function delimiterKeys(obj, delimiter) { | ||
return transformKeys(obj, (str) => toDelimiterCase(str, delimiter)); | ||
return transformKeys(obj, (str) => delimiterCase(str, delimiter)); | ||
} | ||
// src/utils/object-keys/kebab-keys.ts | ||
function kebabKeys(obj) { | ||
return transformKeys(obj, toKebabCase); | ||
return transformKeys(obj, kebabCase); | ||
} | ||
// src/utils/object-keys/pascal-keys.ts | ||
function pascalKeys(obj) { | ||
return transformKeys(obj, toPascalCase); | ||
return transformKeys(obj, pascalCase); | ||
} | ||
// src/utils/object-keys/snake-keys.ts | ||
function snakeKeys(obj) { | ||
return transformKeys(obj, toSnakeCase); | ||
return transformKeys(obj, snakeCase); | ||
} | ||
// src/deep-key-casing.ts | ||
// src/utils/object-keys/deep-transform-keys.ts | ||
function deepTransformKeys(obj, transform) { | ||
@@ -249,68 +266,83 @@ if (!["object", "array"].includes(typeOf(obj))) | ||
} | ||
// src/utils/object-keys/deep-camel-keys.ts | ||
function deepCamelKeys(obj) { | ||
return deepTransformKeys(obj, toCamelCase); | ||
return deepTransformKeys(obj, camelCase); | ||
} | ||
// src/utils/object-keys/deep-constant-keys.ts | ||
function deepConstantKeys(obj) { | ||
return deepTransformKeys(obj, toConstantCase); | ||
return deepTransformKeys(obj, constantCase); | ||
} | ||
// src/utils/object-keys/deep-delimiter-keys.ts | ||
function deepDelimiterKeys(obj, delimiter) { | ||
return deepTransformKeys( | ||
obj, | ||
(str) => toDelimiterCase(str, delimiter) | ||
); | ||
return deepTransformKeys(obj, (str) => delimiterCase(str, delimiter)); | ||
} | ||
// src/utils/object-keys/deep-kebab-keys.ts | ||
function deepKebabKeys(obj) { | ||
return deepTransformKeys(obj, toKebabCase); | ||
return deepTransformKeys(obj, kebabCase); | ||
} | ||
// src/utils/object-keys/deep-pascal-keys.ts | ||
function deepPascalKeys(obj) { | ||
return deepTransformKeys(obj, toPascalCase); | ||
return deepTransformKeys(obj, pascalCase); | ||
} | ||
// src/utils/object-keys/deep-snake-keys.ts | ||
function deepSnakeKeys(obj) { | ||
return deepTransformKeys(obj, toSnakeCase); | ||
return deepTransformKeys(obj, snakeCase); | ||
} | ||
// Annotate the CommonJS export names for ESM import in node: | ||
0 && (module.exports = { | ||
camelKeys, | ||
capitalize, | ||
charAt, | ||
concat, | ||
constantKeys, | ||
deepCamelKeys, | ||
deepConstantKeys, | ||
deepDelimiterKeys, | ||
deepKebabKeys, | ||
deepPascalKeys, | ||
deepSnakeKeys, | ||
deepTransformKeys, | ||
delimiterKeys, | ||
endsWith, | ||
includes, | ||
join, | ||
kebabKeys, | ||
length, | ||
padEnd, | ||
padStart, | ||
pascalKeys, | ||
repeat, | ||
replace, | ||
replaceAll, | ||
slice, | ||
snakeKeys, | ||
split, | ||
startsWith, | ||
toCamelCase, | ||
toConstantCase, | ||
toDelimiterCase, | ||
toKebabCase, | ||
toLowerCase, | ||
toPascalCase, | ||
toSnakeCase, | ||
toTitleCase, | ||
toUpperCase, | ||
trim, | ||
trimEnd, | ||
trimStart, | ||
truncate, | ||
uncapitalize, | ||
words | ||
}); | ||
exports.camelCase = camelCase; | ||
exports.camelKeys = camelKeys; | ||
exports.capitalize = capitalize; | ||
exports.charAt = charAt; | ||
exports.concat = concat; | ||
exports.constantCase = constantCase; | ||
exports.constantKeys = constantKeys; | ||
exports.deepCamelKeys = deepCamelKeys; | ||
exports.deepConstantKeys = deepConstantKeys; | ||
exports.deepDelimiterKeys = deepDelimiterKeys; | ||
exports.deepKebabKeys = deepKebabKeys; | ||
exports.deepPascalKeys = deepPascalKeys; | ||
exports.deepSnakeKeys = deepSnakeKeys; | ||
exports.delimiterCase = delimiterCase; | ||
exports.delimiterKeys = delimiterKeys; | ||
exports.endsWith = endsWith; | ||
exports.includes = includes; | ||
exports.join = join; | ||
exports.kebabCase = kebabCase; | ||
exports.kebabKeys = kebabKeys; | ||
exports.length = length; | ||
exports.lowerCase = lowerCase; | ||
exports.padEnd = padEnd; | ||
exports.padStart = padStart; | ||
exports.pascalCase = pascalCase; | ||
exports.pascalKeys = pascalKeys; | ||
exports.repeat = repeat; | ||
exports.replace = replace; | ||
exports.replaceAll = replaceAll; | ||
exports.reverse = reverse; | ||
exports.slice = slice; | ||
exports.snakeCase = snakeCase; | ||
exports.snakeKeys = snakeKeys; | ||
exports.split = split; | ||
exports.startsWith = startsWith; | ||
exports.titleCase = titleCase; | ||
exports.toCamelCase = toCamelCase; | ||
exports.toConstantCase = toConstantCase; | ||
exports.toDelimiterCase = toDelimiterCase; | ||
exports.toKebabCase = toKebabCase; | ||
exports.toLowerCase = toLowerCase; | ||
exports.toPascalCase = toPascalCase; | ||
exports.toSnakeCase = toSnakeCase; | ||
exports.toTitleCase = toTitleCase; | ||
exports.toUpperCase = toUpperCase; | ||
exports.trimEnd = trimEnd; | ||
exports.trimStart = trimStart; | ||
exports.truncate = truncate; | ||
exports.uncapitalize = uncapitalize; | ||
exports.upperCase = upperCase; | ||
exports.words = words; |
{ | ||
"name": "string-ts", | ||
"version": "1.2.0", | ||
"version": "1.3.0", | ||
"description": "Strongly-typed string functions.", | ||
@@ -11,3 +11,3 @@ "main": "./dist/index.js", | ||
"scripts": { | ||
"build": "tsup ./src/index.ts --format esm,cjs --dts", | ||
"build": "tsup ./src/index.ts --format esm,cjs --dts --treeshake", | ||
"dev": "tsup ./src/index.ts --format esm,cjs --watch --dts", | ||
@@ -20,2 +20,3 @@ "lint": "eslint *.ts*", | ||
"@typescript-eslint/eslint-plugin": "latest", | ||
"@vitest/coverage-v8": "^0.34.6", | ||
"eslint": "latest", | ||
@@ -37,3 +38,4 @@ "prettier": "latest", | ||
"url": "https://github.com/gustavoguichard/string-ts/issues" | ||
} | ||
}, | ||
"sideEfects": false | ||
} |
164
README.md
@@ -1,3 +0,10 @@ | ||
# Strongly-typed string functions for all! | ||
[](https://www.npmjs.org/package/string-ts) | ||
 | ||
[](https://app.codecov.io/gh/gustavoguichard/string-ts) | ||
[](#-contributors) | ||
# string-ts | ||
Strongly-typed string functions for all! | ||
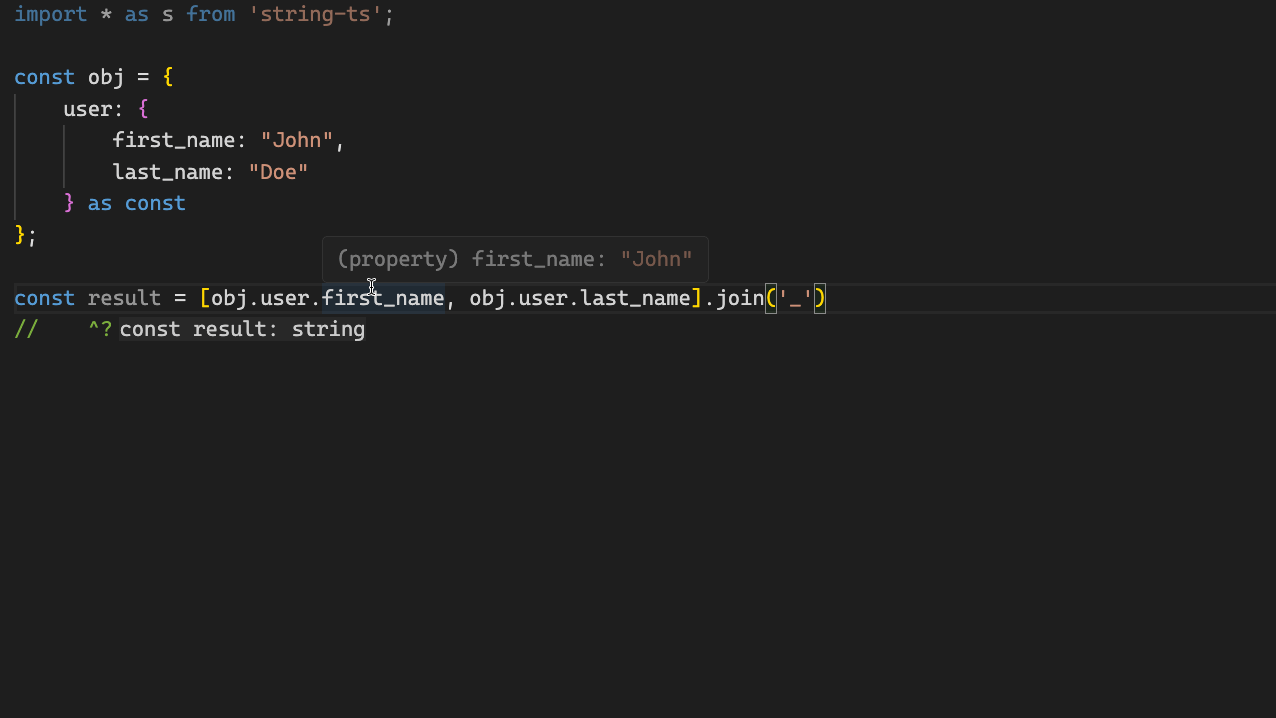 | ||
@@ -85,2 +92,7 @@ | ||
## 🌳 Tree shaking | ||
`string-ts` has been designed with tree shaking in mind. | ||
We have tested it with build tools like Webpack, Vite, Rollup, etc. | ||
## 👌 Supported TypeScript versions | ||
@@ -120,9 +132,10 @@ | ||
- [Strongly-typed alternatives to common loosely-typed functions](#strongly-typed-alternatives-to-common-loosely-typed-functions) | ||
- [toCamelCase](#tocamelcase) | ||
- [toConstantCase](#toconstantcase) | ||
- [toDelimiterCase](#todelimitercase) | ||
- [toKebabCase](#tokebabcase) | ||
- [toPascalCase](#topascalcase) | ||
- [toSnakeCase](#tosnakecase) | ||
- [toTitleCase](#totitlecase) | ||
- [camelCase](#camelcase) | ||
- [constantCase](#constantcase) | ||
- [delimiterCase](#delimitercase) | ||
- [kebabCase](#kebabcase) | ||
- [pascalCase](#pascalcase) | ||
- [reverse](#reverse) | ||
- [snakeCase](#snakecase) | ||
- [titleCase](#titlecase) | ||
- [truncate](#truncate) | ||
@@ -421,15 +434,28 @@ - [words](#words) | ||
### toCamelCase | ||
### lowerCase | ||
This function converts a string to `lower case` at both runtime and type levels. | ||
_NOTE: this function will split by words and join them with `" "`, unlike `toLowerCase`._ | ||
```ts | ||
import { lowerCase } from 'string-ts' | ||
const str = 'HELLO-WORLD' | ||
const result = lowerCase(str) | ||
// ^ 'hello world' | ||
``` | ||
### camelCase | ||
This function converts a string to `camelCase` at both runtime and type levels. | ||
```ts | ||
import { toCamelCase } from 'string-ts' | ||
import { camelCase } from 'string-ts' | ||
const str = 'hello-world' | ||
const result = toCamelCase(str) | ||
const result = camelCase(str) | ||
// ^ 'helloWorld' | ||
``` | ||
### toConstantCase | ||
### constantCase | ||
@@ -439,10 +465,10 @@ This function converts a string to `CONSTANT_CASE` at both runtime and type levels. | ||
```ts | ||
import { toConstantCase } from 'string-ts' | ||
import { constantCase } from 'string-ts' | ||
const str = 'helloWorld' | ||
const result = toConstantCase(str) | ||
const result = constantCase(str) | ||
// ^ 'HELLO_WORLD' | ||
``` | ||
### toDelimiterCase | ||
### delimiterCase | ||
@@ -452,10 +478,10 @@ This function converts a string to a new case with a custom delimiter at both runtime and type levels. | ||
```ts | ||
import { toDelimiterCase } from 'string-ts' | ||
import { delimiterCase } from 'string-ts' | ||
const str = 'helloWorld' | ||
const result = toDelimiterCase(str, '.') | ||
const result = delimiterCase(str, '.') | ||
// ^ 'hello.World' | ||
``` | ||
### toKebabCase | ||
### kebabCase | ||
@@ -465,10 +491,10 @@ This function converts a string to `kebab-case` at both runtime and type levels. | ||
```ts | ||
import { toKebabCase } from 'string-ts' | ||
import { kebabCase } from 'string-ts' | ||
const str = 'helloWorld' | ||
const result = toKebabCase(str) | ||
const result = kebabCase(str) | ||
// ^ 'hello-world' | ||
``` | ||
### toPascalCase | ||
### pascalCase | ||
@@ -478,10 +504,10 @@ This function converts a string to `PascalCase` at both runtime and type levels. | ||
```ts | ||
import { toPascalCase } from 'string-ts' | ||
import { pascalCase } from 'string-ts' | ||
const str = 'hello-world' | ||
const result = toPascalCase(str) | ||
const result = pascalCase(str) | ||
// ^ 'HelloWorld' | ||
``` | ||
### toSnakeCase | ||
### snakeCase | ||
@@ -491,10 +517,10 @@ This function converts a string to `snake_case` at both runtime and type levels. | ||
```ts | ||
import { toSnakeCase } from 'string-ts' | ||
import { snakeCase } from 'string-ts' | ||
const str = 'helloWorld' | ||
const result = toSnakeCase(str) | ||
const result = snakeCase(str) | ||
// ^ 'hello_world' | ||
``` | ||
### toTitleCase | ||
### titleCase | ||
@@ -504,9 +530,34 @@ This function converts a string to `Title Case` at both runtime and type levels. | ||
```ts | ||
import { toTitleCase } from 'string-ts' | ||
import { titleCase } from 'string-ts' | ||
const str = 'helloWorld' | ||
const result = toTitleCase(str) | ||
const result = titleCase(str) | ||
// ^ 'Hello World' | ||
``` | ||
### upperCase | ||
This function converts a string to `UPPER CASE` at both runtime and type levels. | ||
_NOTE: this function will split by words and join them with `" "`, unlike `toUpperCase`._ | ||
```ts | ||
import { upperCase } from 'string-ts' | ||
const str = 'hello-world' | ||
const result = upperCase(str) | ||
// ^ 'HELLO WORLD' | ||
``` | ||
### reverse | ||
This function reverses a string. | ||
```ts | ||
import { reverse } from 'string-ts' | ||
const str = 'Hello StringTS!' | ||
const result = reverse(str) | ||
// ^ '!TSgnirtS olleH' | ||
``` | ||
### truncate | ||
@@ -516,3 +567,2 @@ | ||
```ts | ||
@@ -764,2 +814,3 @@ import { truncate } from 'string-ts' | ||
St.ReplaceAll<'hello-world', 'l', '1'> // 'he11o-wor1d' | ||
St.Reverse<'Hello World!'> // '!dlroW olleH' | ||
St.Slice<'hello-world', -5> // 'world' | ||
@@ -777,2 +828,4 @@ St.Split<'hello-world', '-'> // ['hello', 'world'] | ||
#### Core | ||
```ts | ||
@@ -786,4 +839,21 @@ St.CamelCase<'hello-world'> // 'helloWorld' | ||
St.TitleCase<'helloWorld'> // 'Hello World' | ||
``` | ||
// SHALLOW OBJECT KEY TRANSFORMATION | ||
##### Missing types | ||
_Note that we do not include `UpperCase` and `LowerCase` types. These would be too close to the existing TS types `Uppercase` and `Lowercase`._ | ||
One could create either by doing like so: | ||
```ts | ||
type LowerCase<T extends string> = Lowercase<DelimiterCase<T, ' '>> | ||
type UpperCase<T extends string> = Uppercase<DelimiterCase<T, ' '>> | ||
// or | ||
type LowerCase<T extends string> = ReturnType<typeof lowerCase<T>> | ||
type UpperCase<T extends string> = ReturnType<typeof upperCase<T>> | ||
``` | ||
#### Shallow object key transformation | ||
```ts | ||
St.CamelKeys<{ | ||
@@ -806,4 +876,7 @@ 'hello-world': { 'foo-bar': 'baz' } | ||
}> // { 'hello_world': { fooBar: 'baz' } } | ||
``` | ||
// DEEP OBJECT KEY TRANSFORMATION | ||
#### Deep object key transformation | ||
```ts | ||
St.DeepCamelKeys<{ | ||
@@ -867,4 +940,31 @@ 'hello-world': { 'foo-bar': 'baz' } | ||
## 🐝 Contributors | ||
Thanks goes to these wonderful people ([emoji key](https://allcontributors.org/docs/en/emoji-key)): | ||
<!-- ALL-CONTRIBUTORS-LIST:START - Do not remove or modify this section --> | ||
<!-- prettier-ignore-start --> | ||
<!-- markdownlint-disable --> | ||
<table> | ||
<tbody> | ||
<tr> | ||
<td align="center" valign="top" width="14.28%"><a href="https://github.com/gustavoguichard"><img src="https://avatars.githubusercontent.com/u/566971?v=4?s=100" width="100px;" alt="Guga Guichard"/><br /><sub><b>Guga Guichard</b></sub></a><br /><a href="https://github.com/gustavoguichard/string-ts/commits?author=gustavoguichard" title="Code">💻</a> <a href="#projectManagement-gustavoguichard" title="Project Management">📆</a> <a href="#promotion-gustavoguichard" title="Promotion">📣</a> <a href="#maintenance-gustavoguichard" title="Maintenance">🚧</a> <a href="https://github.com/gustavoguichard/string-ts/commits?author=gustavoguichard" title="Documentation">📖</a> <a href="https://github.com/gustavoguichard/string-ts/issues?q=author%3Agustavoguichard" title="Bug reports">🐛</a> <a href="#infra-gustavoguichard" title="Infrastructure (Hosting, Build-Tools, etc)">🚇</a> <a href="#question-gustavoguichard" title="Answering Questions">💬</a> <a href="#research-gustavoguichard" title="Research">🔬</a> <a href="https://github.com/gustavoguichard/string-ts/pulls?q=is%3Apr+reviewed-by%3Agustavoguichard" title="Reviewed Pull Requests">👀</a> <a href="#ideas-gustavoguichard" title="Ideas, Planning, & Feedback">🤔</a> <a href="#example-gustavoguichard" title="Examples">💡</a></td> | ||
<td align="center" valign="top" width="14.28%"><a href="https://github.com/jly36963"><img src="https://avatars.githubusercontent.com/u/33426811?v=4?s=100" width="100px;" alt="Landon Yarrington"/><br /><sub><b>Landon Yarrington</b></sub></a><br /><a href="https://github.com/gustavoguichard/string-ts/commits?author=jly36963" title="Code">💻</a> <a href="#maintenance-jly36963" title="Maintenance">🚧</a> <a href="https://github.com/gustavoguichard/string-ts/commits?author=jly36963" title="Documentation">📖</a> <a href="https://github.com/gustavoguichard/string-ts/pulls?q=is%3Apr+reviewed-by%3Ajly36963" title="Reviewed Pull Requests">👀</a> <a href="#ideas-jly36963" title="Ideas, Planning, & Feedback">🤔</a> <a href="#example-jly36963" title="Examples">💡</a> <a href="#question-jly36963" title="Answering Questions">💬</a> <a href="https://github.com/gustavoguichard/string-ts/issues?q=author%3Ajly36963" title="Bug reports">🐛</a></td> | ||
<td align="center" valign="top" width="14.28%"><a href="https://github.com/p9f"><img src="https://avatars.githubusercontent.com/u/20539361?v=4?s=100" width="100px;" alt="Guillaume"/><br /><sub><b>Guillaume</b></sub></a><br /><a href="https://github.com/gustavoguichard/string-ts/commits?author=p9f" title="Code">💻</a> <a href="#maintenance-p9f" title="Maintenance">🚧</a> <a href="https://github.com/gustavoguichard/string-ts/commits?author=p9f" title="Documentation">📖</a> <a href="https://github.com/gustavoguichard/string-ts/issues?q=author%3Ap9f" title="Bug reports">🐛</a> <a href="#infra-p9f" title="Infrastructure (Hosting, Build-Tools, etc)">🚇</a> <a href="#question-p9f" title="Answering Questions">💬</a> <a href="#ideas-p9f" title="Ideas, Planning, & Feedback">🤔</a></td> | ||
<td align="center" valign="top" width="14.28%"><a href="https://totaltypescript.com"><img src="https://avatars.githubusercontent.com/u/28293365?v=4?s=100" width="100px;" alt="Matt Pocock"/><br /><sub><b>Matt Pocock</b></sub></a><br /><a href="https://github.com/gustavoguichard/string-ts/commits?author=mattpocock" title="Documentation">📖</a> <a href="https://github.com/gustavoguichard/string-ts/commits?author=mattpocock" title="Code">💻</a> <a href="#promotion-mattpocock" title="Promotion">📣</a></td> | ||
<td align="center" valign="top" width="14.28%"><a href="https://luca.md"><img src="https://avatars.githubusercontent.com/u/1881266?v=4?s=100" width="100px;" alt="Andrew Luca"/><br /><sub><b>Andrew Luca</b></sub></a><br /><a href="https://github.com/gustavoguichard/string-ts/commits?author=iamandrewluca" title="Documentation">📖</a> <a href="#promotion-iamandrewluca" title="Promotion">📣</a></td> | ||
<td align="center" valign="top" width="14.28%"><a href="https://github.com/mjuksel"><img src="https://avatars.githubusercontent.com/u/10691584?v=4?s=100" width="100px;" alt="Mjuksel"/><br /><sub><b>Mjuksel</b></sub></a><br /><a href="https://github.com/gustavoguichard/string-ts/commits?author=mjuksel" title="Code">💻</a> <a href="#ideas-mjuksel" title="Ideas, Planning, & Feedback">🤔</a></td> | ||
</tr> | ||
</tbody> | ||
</table> | ||
<!-- markdownlint-restore --> | ||
<!-- prettier-ignore-end --> | ||
<!-- ALL-CONTRIBUTORS-LIST:END --> | ||
This project follows the [all-contributors](https://github.com/all-contributors/all-contributors) specification. Contributions of any kind welcome! | ||
## 🫶 Acknowledgements | ||
This library got a lot of inspiration from libraries such as [lodash](https://github.com/lodash/lodash), [ts-reset](https://github.com/total-typescript/ts-reset), [type-fest](https://github.com/sindresorhus/type-fest), [HOTScript](https://github.com/gvergnaud/hotscript), and many others. |
Sorry, the diff of this file is not supported yet
79098
16.44%1250
4.25%956
11.68%7
16.67%