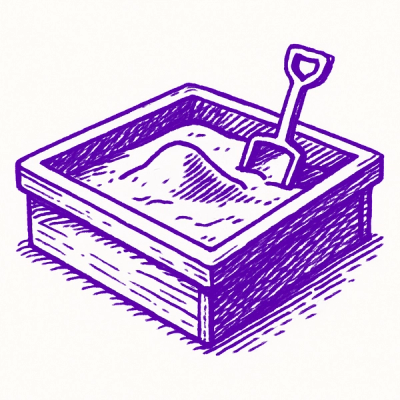
Research
/Security News
Critical Vulnerability in NestJS Devtools: Localhost RCE via Sandbox Escape
A flawed sandbox in @nestjs/devtools-integration lets attackers run code on your machine via CSRF, leading to full Remote Code Execution (RCE).
style-inject
Advanced tools
The 'style-inject' npm package is a utility for injecting CSS styles into the DOM. It is particularly useful for dynamically adding styles to a web page without needing to modify the HTML or CSS files directly. This can be useful in various scenarios, such as theming, dynamic styling, or when working with component-based frameworks.
Injecting CSS Styles
This feature allows you to inject a string of CSS styles directly into the DOM. The provided code sample demonstrates how to change the background color of the body to blue.
const styleInject = require('style-inject');
const css = 'body { background-color: blue; }';
styleInject(css);
Injecting CSS with Options
This feature allows you to inject CSS styles with additional options. In this example, the CSS is inserted at the top of the head element, which can be useful for ensuring that the styles take precedence over others.
const styleInject = require('style-inject');
const css = 'body { background-color: red; }';
const options = { insertAt: 'top' };
styleInject(css, options);
The 'insert-css' package provides similar functionality by allowing you to insert CSS into the DOM. It offers a simple API for adding styles and is often used in component-based frameworks. Compared to 'style-inject', 'insert-css' is more focused on simplicity and ease of use.
The 'styled-components' package is a popular library for styling React components using tagged template literals. It allows for dynamic styling and theming within React applications. While 'styled-components' offers more advanced features and is tightly integrated with React, 'style-inject' is more lightweight and can be used in any JavaScript environment.
The 'emotion' package is a library designed for writing CSS styles with JavaScript. It provides powerful and flexible styling capabilities, including support for theming and dynamic styles. 'Emotion' is more feature-rich and versatile compared to 'style-inject', which is more focused on simple CSS injection.
Inject style tag to document head.
npm install style-inject
import styleInject from 'style-inject';
const css = `
body {
margin: 0;
}
`;
styleInject(css, options);
Type: string
Possible values: top
Default: undefined
Insert style
tag to specific position of head
element.
MIT © EGOIST
FAQs
Inject style tag to document head.
The npm package style-inject receives a total of 987,084 weekly downloads. As such, style-inject popularity was classified as popular.
We found that style-inject demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 2 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
/Security News
A flawed sandbox in @nestjs/devtools-integration lets attackers run code on your machine via CSRF, leading to full Remote Code Execution (RCE).
Product
Customize license detection with Socket’s new license overlays: gain control, reduce noise, and handle edge cases with precision.
Product
Socket now supports Rust and Cargo, offering package search for all users and experimental SBOM generation for enterprise projects.