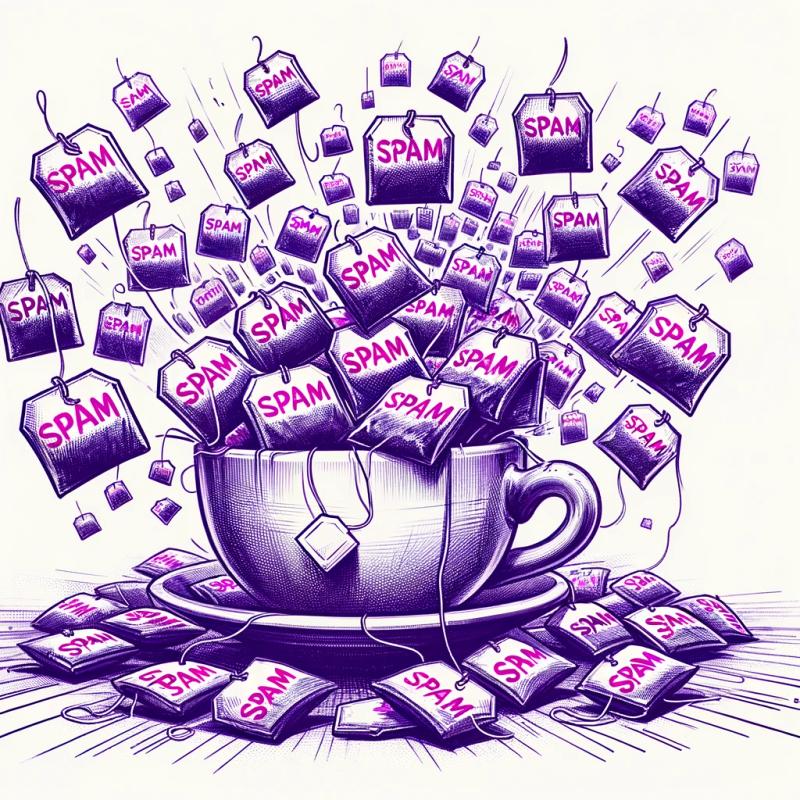
Security News
tea.xyz Spam Plagues npm and RubyGems Package Registries
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
svelte-vertical-timeline
Advanced tools
Readme
Svelte components for creating a vertical timeline.
Check out the demo.
yarn add svelte-vertical-timeline
or
npm install svelte-vertical-timeline
A timeline consists of components below.
<Timeline/>
<TimelineItem/>
<TimelineSeparator/>
<TimelineDot/>
<TimelineConnector/>
<TimelineContent/>
<TimelineOppositeContent/>
I listed some examples of timelines you can create with this library below.
A basic timeline showing list of events.
<script>
import {
Timeline,
TimelineItem,
TimelineSeparator,
TimelineDot,
TimelineConnector,
TimelineContent
} from 'svelte-vertical-timeline';
const options = [{ title: 'Eat' }, { title: 'Sleep' }, { title: 'Code' }];
</script>
<Timeline>
{#each options as option}
<TimelineItem>
<TimelineSeparator>
<TimelineDot />
<TimelineConnector />
</TimelineSeparator>
<TimelineContent>
<h3>{option.title}</h3>
</TimelineContent>
</TimelineItem>
{/each}
</Timeline>
The main content of the timeline can be positioned on the left side.
<script>
import {
Timeline,
TimelineItem,
TimelineSeparator,
TimelineDot,
TimelineConnector,
TimelineContent
} from 'svelte-vertical-timeline';
const options = [{ title: 'Eat' }, { title: 'Sleep' }, { title: 'Code' }];
</script>
<Timeline position="left">
{#each options as option}
<TimelineItem>
<TimelineSeparator>
<TimelineDot />
<TimelineConnector />
</TimelineSeparator>
<TimelineContent>
<h3>{option.title}</h3>
</TimelineContent>
</TimelineItem>
{/each}
</Timeline>
The timeline can display the events on alternating sides.
<script>
import {
Timeline,
TimelineItem,
TimelineSeparator,
TimelineDot,
TimelineConnector,
TimelineContent
} from 'svelte-vertical-timeline';
const options = [{ title: 'Eat' }, { title: 'Sleep' }, { title: 'Code' }];
</script>
<Timeline position="alternate">
{#each options as option}
<TimelineItem>
<TimelineSeparator>
<TimelineDot />
<TimelineConnector />
</TimelineSeparator>
<TimelineContent>
<h3>{option.title}</h3>
</TimelineContent>
</TimelineItem>
{/each}
</Timeline>
The timeline can display content on opposite sides.
Please make sure to add slot="opposite-content"
to the <TimelineOppositeContent/>
component.
<script>
import {
Timeline,
TimelineItem,
TimelineSeparator,
TimelineDot,
TimelineConnector,
TimelineContent,
TimelineOppositeContent
} from 'svelte-vertical-timeline';
const options = [
{ title: 'Eat', time: '09:30 am' },
{ title: 'Sleep', time: '10:00 am' },
{ title: 'Code', time: '11:00 am' },
{ title: 'Eat', time: '01:00 pm' }
];
</script>
<Timeline position="alternate">
{#each options as option}
<TimelineItem>
<TimelineOppositeContent slot="opposite-content">
<p>{option.time}</p>
</TimelineOppositeContent>
<TimelineSeparator>
<TimelineDot />
<TimelineConnector />
</TimelineSeparator>
<TimelineContent>
<h3>{option.title}</h3>
</TimelineContent>
</TimelineItem>
{/each}
</Timeline>
You can customize the timeline anyway you want. Check here for furthur information.
<script>
import {
Timeline,
TimelineItem,
TimelineSeparator,
TimelineDot,
TimelineConnector,
TimelineContent,
TimelineOppositeContent
} from 'svelte-vertical-timeline';
</script>
<!-- Icons from: https://icons8.com/pricing -->
<Timeline position="alternate">
<TimelineItem>
<TimelineOppositeContent slot="opposite-content">
<p>09:30 am</p>
</TimelineOppositeContent>
<TimelineSeparator>
<TimelineDot
style={'width: 45px; height: 45px; background-color: #fff; display: flex; justify-content: center; border-color: transparent; '}
>
<img src="https://img.icons8.com/nolan/64/taco.png" />
</TimelineDot>
<TimelineConnector />
</TimelineSeparator>
<TimelineContent style={'height: 150px;'}>
<h3>Eat</h3>
<p>You need to eat.</p>
</TimelineContent>
</TimelineItem>
<TimelineItem>
<TimelineOppositeContent slot="opposite-content">
<p style={'margin-top: -1px;'}>10:30 am</p>
</TimelineOppositeContent>
<TimelineSeparator>
<TimelineDot style={'background-color: #FEC048;'} />
<TimelineConnector />
</TimelineSeparator>
<TimelineContent>
<h3 style={'margin-top: -2px;'}>Sleep</h3>
<p style={'margin-top: -2px;'}>You need to take a nap.</p>
</TimelineContent>
</TimelineItem>
<TimelineItem>
<TimelineOppositeContent slot="opposite-content">
<p>11:00 am</p>
</TimelineOppositeContent>
<TimelineSeparator>
<TimelineDot
style={'width: 45px; height: 45px; background-color: #fff; display: flex; justify-content: center; border-color: transparent; '}
>
<img src="https://img.icons8.com/doodle/48/000000/svetle.png" />
</TimelineDot>
<TimelineConnector />
</TimelineSeparator>
<TimelineContent style={'height: 200px;'}>
<h3>Code</h3>
<p>Svelte is Awesome.</p>
</TimelineContent>
</TimelineItem>
<TimelineItem>
<TimelineOppositeContent slot="opposite-content">
<p>01:00 am</p>
</TimelineOppositeContent>
<TimelineSeparator>
<TimelineDot
style={'width: 45px; height: 65px; background-color: #fff; display: flex; justify-content: center; border-color: transparent; '}
>
<img src="https://img.icons8.com/nolan/64/birthday-cake.png" />
</TimelineDot>
</TimelineSeparator>
<TimelineContent style={'height: 200px;'}>
<h3>Snack</h3>
<p>You need to treat yourself.</p>
</TimelineContent>
</TimelineItem>
</Timeline>
<style>
p {
margin: px 0;
color: grey;
}
</style>
<Timeline/>
Name | type | isRequired | Description |
---|---|---|---|
position | right , left or alternate | x | The position where the TimelineContent should appear |
style | string | x | Custom style for this component |
<TimelineItem/>
Name | type | isRequired | Description |
---|---|---|---|
position | right or left | x | The position where the timeline's item should appear |
style | string | x | Custom style for this component |
<TimelineSeparator/>
Name | type | isRequired | Description |
---|---|---|---|
style | string | x | Custom style for this component |
<TimelineDot/>
Name | type | isRequired | Description |
---|---|---|---|
style | string | x | Custom style for this component |
<TimelineConnector/>
Name | type | isRequired | Description |
---|---|---|---|
style | string | x | Custom style for this component |
<TimelineContent/>
Name | type | isRequired | Description |
---|---|---|---|
style | string | x | Custom style for this component |
<TimelineOppositeContent/>
Name | type | isRequired | Description |
---|---|---|---|
style | string | x | Custom style for this component |
FAQs
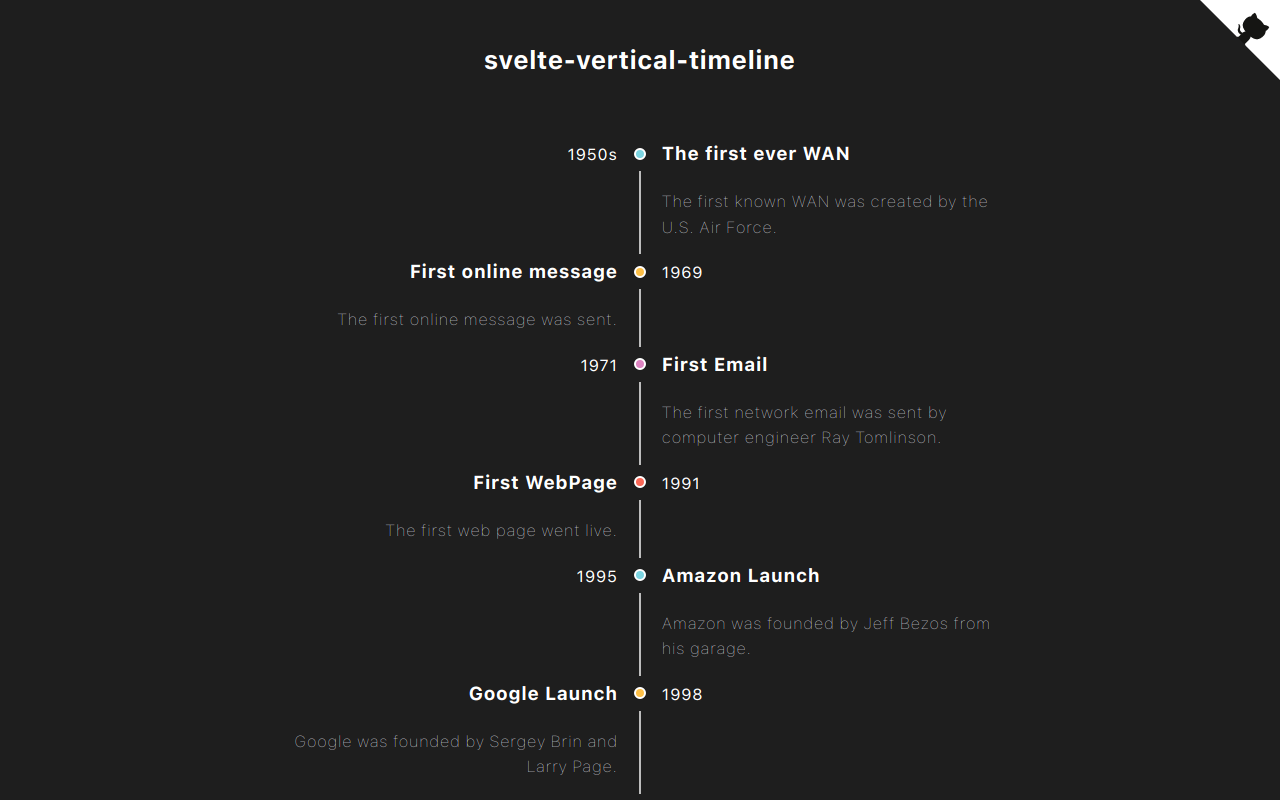
The npm package svelte-vertical-timeline receives a total of 297 weekly downloads. As such, svelte-vertical-timeline popularity was classified as not popular.
We found that svelte-vertical-timeline demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
Security News
As cyber threats become more autonomous, AI-powered defenses are crucial for businesses to stay ahead of attackers who can exploit software vulnerabilities at scale.
Security News
UnitedHealth Group disclosed that the ransomware attack on Change Healthcare compromised protected health information for millions in the U.S., with estimated costs to the company expected to reach $1 billion.