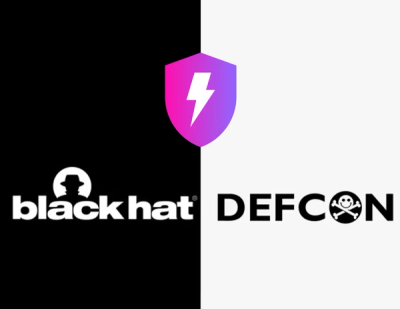
Security News
Meet Socket at Black Hat and DEF CON 2025 in Las Vegas
Meet Socket at Black Hat & DEF CON 2025 for 1:1s, insider security talks at Allegiant Stadium, and a private dinner with top minds in software supply chain security.
svgo-loader
Advanced tools
svgo-loader is an npm package that optimizes SVG files by using the SVGO (SVG Optimizer) library. It is typically used as a loader in webpack configurations to automatically optimize SVG files during the build process, reducing file size and improving performance.
Basic SVG Optimization
This feature allows you to optimize SVG files by applying various SVGO plugins. The code sample demonstrates a basic webpack configuration that uses svgo-loader to remove titles, convert colors, and disable path data conversion.
module.exports = {
module: {
rules: [
{
test: /\.svg$/,
use: [
{
loader: 'svgo-loader',
options: {
plugins: [
{ removeTitle: true },
{ convertColors: { shorthex: false } },
{ convertPathData: false }
]
}
}
]
}
]
}
};
Custom Plugin Configuration
This feature allows you to customize the SVGO plugins used during the optimization process. The code sample shows how to configure svgo-loader to remove dimensions and specific attributes like stroke and fill from SVG files.
module.exports = {
module: {
rules: [
{
test: /\.svg$/,
use: [
{
loader: 'svgo-loader',
options: {
plugins: [
{ removeDimensions: true },
{ removeAttrs: { attrs: '(stroke|fill)' } }
]
}
}
]
}
]
}
};
Integration with Other Loaders
This feature demonstrates how svgo-loader can be integrated with other loaders like babel-loader in a webpack configuration. The code sample shows a setup where SVG files are first processed by babel-loader and then optimized by svgo-loader.
module.exports = {
module: {
rules: [
{
test: /\.svg$/,
use: [
'babel-loader',
{
loader: 'svgo-loader',
options: {
plugins: [
{ removeViewBox: false }
]
}
}
]
}
]
}
};
image-webpack-loader is a similar package that optimizes images, including SVGs, during the webpack build process. It uses various optimization tools like imagemin and SVGO. Compared to svgo-loader, image-webpack-loader is more versatile as it supports multiple image formats, not just SVG.
svg-url-loader is a webpack loader that inlines SVGs as URLs. It can also optimize SVGs using SVGO. Compared to svgo-loader, svg-url-loader provides additional functionality by converting SVGs into data URLs, which can be useful for embedding SVGs directly into HTML or CSS.
$ npm install svgo-loader --save-dev
... or with Yarn
$ yarn add svgo-loader -D
module.exports = {
...,
module: {
rules: [
{
test: /\.svg$/,
type: 'asset',
loader: 'svgo-loader'
}
]
}
}
By default svgo-loader uses config from svgo.config.js
similar to svgo cli.
See how to configure svgo.
Specify configFile option to load custom config module:
module.exports = {
...,
module: {
rules: [
{
test: /\.svg$/,
type: 'asset',
loader: 'svgo-loader',
options: {
configFile: './scripts/svgo.config.js'
}
}
]
}
}
or to disable loading config:
module.exports = {
...,
module: {
rules: [
{
test: /\.svg$/,
type: 'asset',
loader: 'svgo-loader',
options: {
configFile: false
}
}
]
}
}
You can also specify options which override loaded from config
module.exports = {
...,
module: {
rules: [
{
test: /\.svg$/,
type: 'asset',
loader: 'svgo-loader',
options: {
multipass: true,
js2svg: {
indent: 2,
pretty: true,
}
}
}
]
}
}
This software is released under the terms of the MIT license.
FAQs
svgo loader for webpack
The npm package svgo-loader receives a total of 241,458 weekly downloads. As such, svgo-loader popularity was classified as popular.
We found that svgo-loader demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 2 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Meet Socket at Black Hat & DEF CON 2025 for 1:1s, insider security talks at Allegiant Stadium, and a private dinner with top minds in software supply chain security.
Security News
CAI is a new open source AI framework that automates penetration testing tasks like scanning and exploitation up to 3,600× faster than humans.
Security News
Deno 2.4 brings back bundling, improves dependency updates and telemetry, and makes the runtime more practical for real-world JavaScript projects.