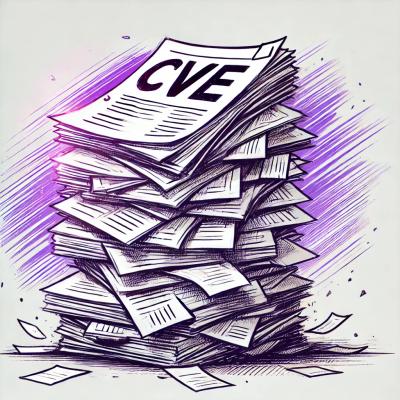
Security News
Node.js EOL Versions CVE Dubbed the "Worst CVE of the Year" by Security Experts
Critics call the Node.js EOL CVE a misuse of the system, sparking debate over CVE standards and the growing noise in vulnerability databases.
swagger-jsdoc
Advanced tools
swagger-jsdoc is a Node.js library that allows you to integrate Swagger/OpenAPI documentation into your Express.js or other Node.js applications. It generates the Swagger specification based on JSDoc comments in your code, making it easier to maintain and update API documentation.
Generate Swagger Documentation
This feature allows you to generate Swagger documentation from JSDoc comments in your code. The `options` object specifies the OpenAPI version, API info, and the files containing the JSDoc comments.
const swaggerJsdoc = require('swagger-jsdoc');
const options = {
definition: {
openapi: '3.0.0',
info: {
title: 'Express API with Swagger',
version: '1.0.0',
},
},
apis: ['./routes/*.js'], // files containing annotations as above
};
const swaggerSpec = swaggerJsdoc(options);
Integrate with Express
This feature demonstrates how to integrate Swagger UI with an Express application. The Swagger documentation is served at the `/api-docs` endpoint.
const express = require('express');
const swaggerUi = require('swagger-ui-express');
const swaggerJsdoc = require('swagger-jsdoc');
const app = express();
const options = {
definition: {
openapi: '3.0.0',
info: {
title: 'Express API with Swagger',
version: '1.0.0',
},
},
apis: ['./routes/*.js'],
};
const swaggerSpec = swaggerJsdoc(options);
app.use('/api-docs', swaggerUi.serve, swaggerUi.setup(swaggerSpec));
app.listen(3000, () => {
console.log('Server is running on port 3000');
});
JSDoc Annotations
This feature shows how to use JSDoc annotations to document an API endpoint. The annotations provide metadata about the endpoint, such as the HTTP method, summary, and response schema.
/**
* @swagger
* /users:
* get:
* summary: Retrieve a list of users
* responses:
* 200:
* description: A list of users
* content:
* application/json:
* schema:
* type: array
* items:
* type: object
* properties:
* id:
* type: integer
* name:
* type: string
*/
app.get('/users', (req, res) => {
res.json([{ id: 1, name: 'John Doe' }]);
});
swagger-ui-express is a middleware for Express.js that serves auto-generated Swagger UI. It is often used in conjunction with swagger-jsdoc to serve the generated Swagger documentation. While swagger-jsdoc focuses on generating the Swagger specification, swagger-ui-express focuses on serving it in a user-friendly interface.
openapi-generator is a tool that can generate client libraries, server stubs, API documentation, and configuration from an OpenAPI Specification. Unlike swagger-jsdoc, which generates the OpenAPI spec from JSDoc comments, openapi-generator takes an existing OpenAPI spec and generates code and documentation from it.
redoc is a tool for generating API documentation from OpenAPI specifications. It provides a customizable and responsive HTML documentation. Unlike swagger-jsdoc, which generates the OpenAPI spec, redoc focuses on rendering the documentation from an existing spec.
This library reads your JSDoc-annotated source code and generates an OpenAPI (Swagger) specification.
Imagine having API files like these:
/**
* @openapi
* /:
* get:
* description: Welcome to swagger-jsdoc!
* responses:
* 200:
* description: Returns a mysterious string.
*/
app.get('/', (req, res) => {
res.send('Hello World!');
});
The library will take the contents of @openapi
(or @swagger
) with the following configuration:
const swaggerJsdoc = require('swagger-jsdoc');
const options = {
definition: {
openapi: '3.0.0',
info: {
title: 'Hello World',
version: '1.0.0',
},
},
apis: ['./src/routes*.js'], // files containing annotations as above
};
const openapiSpecification = swaggerJsdoc(options);
The resulting openapiSpecification
will be a swagger tools-compatible (and validated) specification.
You are viewing swagger-jsdoc
v6 which is published in CommonJS module system.
npm install swagger-jsdoc --save
Or
yarn add swagger-jsdoc
By default swagger-jsdoc
tries to parse all docs to it's best capabilities. If you'd like to you can instruct an Error to be thrown instead if validation failed by setting the options flag failOnErrors
to true
. This is for instance useful if you want to verify that your swagger docs validate using a unit test.
const swaggerJsdoc = require('swagger-jsdoc');
const options = {
failOnErrors: true, // Whether or not to throw when parsing errors. Defaults to false.
definition: {
openapi: '3.0.0',
info: {
title: 'Hello World',
version: '1.0.0',
},
},
apis: ['./src/routes*.js'],
};
const openapiSpecification = swaggerJsdoc(options);
Click on the version you are using for further details:
FAQs
Generates swagger doc based on JSDoc
The npm package swagger-jsdoc receives a total of 620,840 weekly downloads. As such, swagger-jsdoc popularity was classified as popular.
We found that swagger-jsdoc demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 4 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Critics call the Node.js EOL CVE a misuse of the system, sparking debate over CVE standards and the growing noise in vulnerability databases.
Security News
cURL and Go security teams are publicly rejecting CVSS as flawed for assessing vulnerabilities and are calling for more accurate, context-aware approaches.
Security News
Bun 1.2 enhances its JavaScript runtime with 90% Node.js compatibility, built-in S3 and Postgres support, HTML Imports, and faster, cloud-first performance.