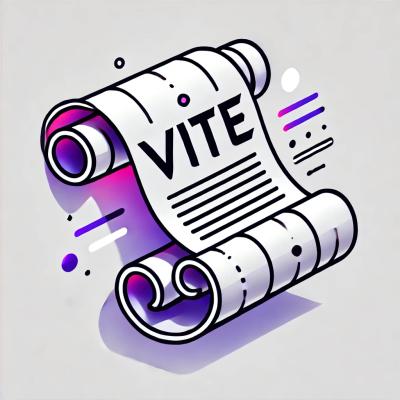
Security News
Vite Releases Technical Preview of Rolldown-Vite, a Rust-Based Bundler
Vite releases Rolldown-Vite, a Rust-based bundler preview offering faster builds and lower memory usage as a drop-in replacement for Vite.
tcp-http-client
Advanced tools
This is a basic http client written in node.js. It uses the built in tls and net modules to send http requests. The client has built in socket pooling, a built in cookie container that can be turned on or off, and also supports using a proxy.
npm install tcp-http-client
This example will send an HTTP POST request to www.example.com. It creates the TCPHttpClient, and then creates a request to be sent by the client. It adds headers and content to the request, then sends the request / receives and then logs the response.
const {TCPHttpClient, TCPHttpRequest, TCPHttpMethod} = require('tcp-http-client');
const { URL } = require('url');
const client = new TCPHttpClient();
const request = new TCPHttpRequest(new URL("https://www.example.com"), TCPHttpMethod.POST);
request.addHeader("name", "value");
request.addContent("Example Content", "text/plain");
const response = await client.sendRequest(request); //returns a promise
console.log(response.content.toString()); //response.content will be a byte[] by default. It does not automatically decode encoded data.
client.dispose(); //Make sure to dispose of the client when finished using.
This class is the main class responsible for sending and receiving requests over sockets using net and tls modules. It does the parsing of http responses, and also connects to the proxy.
This class is used to create a request to be used with the TCPHttpClient.
This class represents the raw HTTP message that is sent back from the server when using TCPHttpClient.sendRequest()
FAQs
Basic Http client using built in net and tls modules
The npm package tcp-http-client receives a total of 0 weekly downloads. As such, tcp-http-client popularity was classified as not popular.
We found that tcp-http-client demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Vite releases Rolldown-Vite, a Rust-based bundler preview offering faster builds and lower memory usage as a drop-in replacement for Vite.
Research
Security News
A malicious npm typosquat uses remote commands to silently delete entire project directories after a single mistyped install.
Research
Security News
Malicious PyPI package semantic-types steals Solana private keys via transitive dependency installs using monkey patching and blockchain exfiltration.