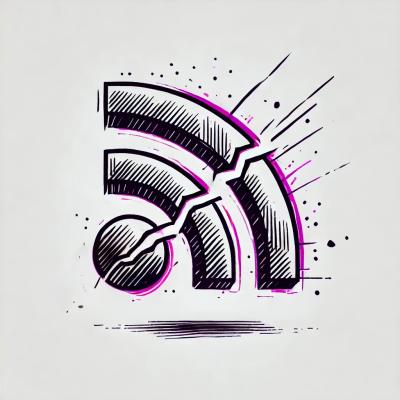
Security News
CISA Kills Off RSS Feeds for KEVs and Cyber Alerts
CISA is discontinuing official RSS support for KEV and cybersecurity alerts, shifting updates to email and social media, disrupting automation workflows.
:warning: We are aware of the migration from TDA to Schwab and will update this client as soon as the api information is made available. link
A Javascript thick client for the TD-Ameritrade Restful API written in Typescript for nodejs.
$ yarn add tdaclient
To gain access to the Td-Ameritrade (TDA) APIs, you will need to get an access token from your trading account.
You can get an access token by:
At the bare minimum, you will need to provide an access token and client id in order to instantiate the tdaClient. This can be done in a few ways:
import {TdaClient} from "tdaclient";
const tdaClient = TdaClient.from({
access_token: "MY-ACCESS-TOKEN",
client_id: "MY-CLIENT-ID",
refresh_token: "MY-REFRESH-TOKEN" // Optional: Refresh token is used to renew the access_token
});
The tdaClient will read the credentials from this file. Make sure that the tdaClient is able to read and write to this file.
import {TdaClient} from "tdaclient";
/* Example CREDENTIAL_FILE.json file
{
"access_token": "MY-ACCESS-TOKEN",
"refresh_token": "MY-REFRESH-TOKEN",
"client_id": "MY-CLIENT-ID"
}
*/
const tdaClient = TdaClient.from({
fileName: PATH_TO_CREDENTIAL_FILE_NAME,
});
You can specify your own way of providing the credential information. Here is an example of reading and writing to a
credential file stored in S3. The basic idea here is to create a class that extends the CredentialProvider abstract
class. You need to override the getCredential
and the updateCredential
member functions. You then pass that provider
when you instantiate the tdaClient.
export class S3CredentialProvider extends CredentialProvider {
async updateCredential(tdaCredential: TdaCredential): Promise<void> {
// do something
}
async getCredential(): Promise<TdaCredential> {
// do something
}
}
const tdaClient = TdaClient.from({
authorizationInterceptor: new AuthorizationTokenInterceptor(new S3CredentialProvider())
});
If you provide a refresh token to the tdaClient, when the access token expires, the tdaClient will automatically fetch a new access token using the existing valid refresh token. When the refresh token expires, the tdaClient will automatically refresh the credential information.
import {
AssetType,
DurationType,
InstructionType, Order, OrdersConfig,
OrderStrategyType,
OrderType,
SessionType
} from "tdaclient/dist/models/order";
// ...
const order = {
orderType: OrderType.LIMIT,
price: 100.0,
session: SessionType.NORMAL,
duration: DurationType.DAY,
orderStrategyType: OrderStrategyType.SINGLE,
orderLegCollection: [
{
instruction: InstructionType.BUY,
quantity: 1,
instrument: {
symbol: 'SPY',
assetType: AssetType.EQUITY,
},
},
],
} as Order;
const orderConfig = {
accountId,
order,
} as OrdersConfig;
const placeOrdersResponse = await tdaClient.placeOrder(orderConfig);
const orderId = placeOrdersResponse.orderId;
console.log(orderId);
import {getOptionChain} from "tdaclient/dist/optionChain";
import {
ContractType,
OptionChainConfig,
OptionStrategyType
} from "tdaClient/dist/models/optionChain";
const optionChainResponse = await tdaClient.getOptionChain({
symbol: 'SPY',
strike: 470,
strikeCount: 10
} as OptionChainConfig)
console.log(optionChainResponse);
import { HoursConfig } from "tdaClient/dist/models/hours";
const response = await tdaClient.getHours({
markets: ['EQUITY', 'OPTION']
} as HoursConfig);
console.log(response);
import { PriceHistoryConfig } from "tdaClient/dist/models/priceHistory";
const response = await tdaClient.getPriceHistory({
symbol: 'SPX',
periodType: 'day',
period: 1,
needExtendedHoursData: false,
} as PriceHistoryConfig);
console.log(response);
For more info refer to this page
credentials.json
and put it in the package root directory.{
"client_id": "example-client-id",
"redirect_uri": "example-redirect-uri"
}
node generateAuthUrl.js
code
.decodeURIComponent("MY_CODE")
credentials.json
{
"access_token": "example-token",
"refresh_token": "example-refresh-token",
"scope": "PlaceTrades AccountAccess MoveMoney",
"expires_in": 1800, // 30 minutes
"refresh_token_expires_in": 7776000, // 90 days
"token_type": "Bearer",
"client_id": "example-client-id",
"redirect_uri": "example-redirect-uri"
}
yarn test
and it will run the integration testsThis command will update the package version and then publish to the public npm repo
$ npm version [patch | minor major]
$ npm publish
Refer to this page for more info on TDA's APIs
tdaClient is MIT licensed.
FAQs
TD Ameritrade Client
The npm package tdaclient receives a total of 10 weekly downloads. As such, tdaclient popularity was classified as not popular.
We found that tdaclient demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
CISA is discontinuing official RSS support for KEV and cybersecurity alerts, shifting updates to email and social media, disrupting automation workflows.
Security News
The MCP community is launching an official registry to standardize AI tool discovery and let agents dynamically find and install MCP servers.
Research
Security News
Socket uncovers an npm Trojan stealing crypto wallets and BullX credentials via obfuscated code and Telegram exfiltration.