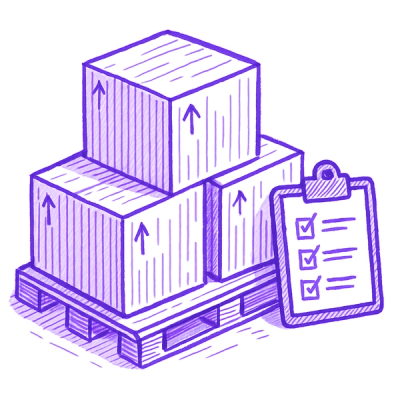
Security News
Open Source Maintainers Feeling the Weight of the EU’s Cyber Resilience Act
The EU Cyber Resilience Act is prompting compliance requests that open source maintainers may not be obligated or equipped to handle.
terminal-link
Advanced tools
The terminal-link npm package allows you to create clickable links in the terminal. This can be useful for providing users with easy access to URLs directly from the command line.
Basic Link Creation
This feature allows you to create a basic clickable link in the terminal. The link text ('My Website') will be clickable and will direct the user to the specified URL ('https://example.com').
const terminalLink = require('terminal-link');
const link = terminalLink('My Website', 'https://example.com');
console.log(link);
Conditional Link Creation
This feature allows you to create a link that only becomes clickable if the terminal supports it. If not, it will fall back to a plain text URL.
const terminalLink = require('terminal-link');
const link = terminalLink.isSupported ? terminalLink('My Website', 'https://example.com') : 'My Website (https://example.com)';
console.log(link);
Customizing Link Styles
This feature allows you to customize the appearance of the link text using other libraries like chalk. In this example, the link text is styled to be blue and underlined.
const terminalLink = require('terminal-link');
const chalk = require('chalk');
const link = terminalLink(chalk.blue.underline('My Website'), 'https://example.com');
console.log(link);
The ink-link package is used within the Ink framework to create clickable links in React-based terminal applications. It offers similar functionality to terminal-link but is designed to work seamlessly with Ink's component-based architecture.
Create clickable links in the terminal
npm install terminal-link
import terminalLink from 'terminal-link';
const link = terminalLink('My Website', 'https://sindresorhus.com');
console.log(link);
Create a link for use in stdout.
For unsupported terminals, the link will be printed in parens after the text: My website (https://sindresorhus.com)
.
Type: string
Text to linkify.
Type: string
URL to link to.
Type: object
Type: Function | boolean
Override the default fallback. The function receives the text
and url
as parameters and is expected to return a string.
If set to false
, the fallback will be disabled when a terminal is unsupported.
Type: boolean
Check whether the terminal's stdout supports links.
Prefer just using the default fallback or the fallback
option whenever possible.
Create a link for use in stderr.
Same arguments as terminalLink()
.
Type: boolean
Check whether the terminal's stderr supports links.
Prefer just using the default fallback or the fallback
option whenever possible.
FAQs
Create clickable links in the terminal
The npm package terminal-link receives a total of 13,300,457 weekly downloads. As such, terminal-link popularity was classified as popular.
We found that terminal-link demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
The EU Cyber Resilience Act is prompting compliance requests that open source maintainers may not be obligated or equipped to handle.
Security News
Crates.io adds Trusted Publishing support, enabling secure GitHub Actions-based crate releases without long-lived API tokens.
Research
/Security News
Undocumented protestware found in 28 npm packages disrupts UI for Russian-language users visiting Russian and Belarusian domains.