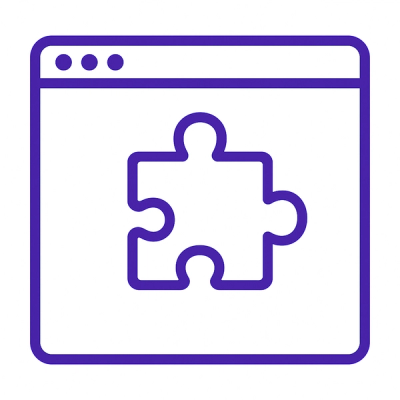
Research
Security News
The Growing Risk of Malicious Browser Extensions
Socket researchers uncover how browser extensions in trusted stores are used to hijack sessions, redirect traffic, and manipulate user behavior.
termux-api-library
Advanced tools
Termux-API library for NodeJS
Make sure you've first installed the termux and termux-api on your Android device from the f-droid or github releases.
To use Termux:API you also need to install the termux-api package.
pkg install termux-api
npm i --save termux-api-library
library import
const api = require('termux-api-library');
or
import api from 'termux-api-library';
const isTermux = api.isTermux // return is true or false
if (isTermux) {
await api.termux_wifi_connectioninfo((e) => {
console.log(e);
});
}
await api.isTermuxApi((e) => {
console.log(e); // return is true or false
});
or
const isTermuxApi = await api.isTermuxApi();
console.log(isTermuxApi); // return is true or false
Get the status of the device battery.
await api.termux_battery_status((e) => {
console.log(e);
});
Set the screen brightness between 0 and 255.
await api.termux_brightness(255);
List call log history.
await api.termux_call_log(10, (e) => {
console.log(e);
})
Get information about device camera(s).
await api.termux_camera_info((e) => {
console.log(e);
})
Take a photo and save it to a file in JPEG format.
await api.termux_camera_photo(0, './test.jpeg');
Get the system clipboard text.
await api.termux_clipboard_get((e) => {
console.log(e);
})
Set the system clipboard text.
await api.termux_clipboard_set("hello world")
List all contacts.
await api.termux_contact_list((e) => {
console.log(e);
})
Show a text entry dialog.
const hint = 'Put your password here'
const title = 'input password'
await api.termux_dialog(hint, title, false, true, true, (e) => {
console.log(e);
})
Download a files
const url = 'https://example.com/image.jpeg'
const filename = 'photo_2022'
const savePath = '/data/data/com.termux/files/home'
await api.termux_download(url, filename, savePath);
Use fingerprint sensor on device to check for authentication.
await api.termux_fingerprint((e) => {
console.log(e);
})
Get the device location.
await api.termux_location("gps", "once", (e) => {
console.log(e);
})
or
const data = await api.termux_location('gps', 'once')
console.log(data);
Play media files.
const info = await api.termux_media_player.info();
console.log(info);
const play = await api.termux_media_player.play();
console.log(play);
const playFile = await api.termux_media_player.playFile(path);
console.log(playFile);
const pause = await api.termux_media_player.pause();
console.log(pause);
const stop = await api.termux_media_player.stop();
console.log(stop);
Recording using microphone on your device.
const info = await api.termux_microphone_record.info();
console.log(info);
const path = '/data/data/com.termux/files/home/filename.mp3'
const limit = 0
const start = await api.termux_microphone_record.start(path, limit);
console.log(start);
const stop = await api.termux_microphone_record.stop();
console.log(stop);
await api.termux_notification(title, text, id);
await api.termux_notification_remove(id);
await api.termux_sensor((e) => {
console.log(e);
});
or
const sensor = await api.termux_sensor();
console.log(sensor);
const filepath = "../image.jpeg"
await api.termux_share('send', filepath);
const type = "inbox"
const limit = 10
const sender = undefined || "all" // the number for locate message : To display all senders, type the "all" or don't assign a value to the variable
await api.termux_sms_list(type, limit, sender, (e) => {
console.log(e);
});
or
const list = await api.termux_sms_list(type, limit, sender);
console.log(list);
const number = 05592xxxxx
const slot = "0"
const text = "hello world"
await api.termux_sms_send(number, slot, text);
// connect
const number = 05592xxxxx
await api.termux_telephony_call.connect(number);
// Close call .
await api.termux_telephony_call.close();
const info = await api.termux_telephony_cellinfo();
console.log(info);
const deviceinfo = await api.termux_telephony_deviceinfo();
console.log(deviceinfo);
const position = "middle"
const background = "gray"
const text_color = "white"
const text = "hello world"
await api.termux_toast(position, background, text_color, text);
const values = "on" // on - enable torch. | off - disable torch.
await api.termux_torch(values);
await api.termux_wifi_connectioninfo((e) => {
console.log(e);
});
or
const wifi = await api.termux_wifi_connectioninfo();
console.log(wifi);
const values = true // true - enable Wi-Fi | false - disable Wi-Fi
await api.termux_wifi_enable(values);
await api.termux_wifi_scaninfo((e) => {
console.log(e);
});
or
const scaninfo = await api.termux_wifi_scaninfo();
console.log(scaninfo);
FAQs
Termux-api library for NodeJS
The npm package termux-api-library receives a total of 5 weekly downloads. As such, termux-api-library popularity was classified as not popular.
We found that termux-api-library demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
Socket researchers uncover how browser extensions in trusted stores are used to hijack sessions, redirect traffic, and manipulate user behavior.
Research
Security News
An in-depth analysis of credential stealers, crypto drainers, cryptojackers, and clipboard hijackers abusing open source package registries to compromise Web3 development environments.
Security News
pnpm 10.12.1 introduces a global virtual store for faster installs and new options for managing dependencies with version catalogs.