text-annotator
News
We released a new version of text-annotator: text-annotator-v2, with some improvements and breaking changes. We will still maintain this library here and it still works in our product stably. But you are welcome to try text-annotator-v2 which is supposed to be more robust and lightweight.
Introduction
text-annotator is a JavaScript library for annotating plain text in the HTML.
The annotation process is:
- Search: Search for a piece of plain text in the HTML; if finding it, store its location identified by an index and then return the index for later annotation
- Annotate: Annotate the found text given its index
It can be seen that in order to annotate a piece of text, two steps, search and annotate, are taken. The idea of decomposing the annotation process into the two steps is to allow more flexibility, e.g., the user can search for all pieces of text first, and then annotate them later when required (e.g., when clicking a button). There is also a function combining the two steps, as can be seen in the An example of the usage section.
text-annotator can be used in the browser or the Node.js server.
Import
install it via npm
npm install --save text-annotator
import TextAnnotator from 'text-annotator'
include it into the head tag
<script src="public/js/text-annotator.min.js"></script>
An example of the usage
var annotator = new TextAnnotator({content: document.getElementById('content').innerHTML})
var highlightIndex = annotator.search('EMBL-EBI')
if (highlightIndex !== -1) {
document.getElementById('content').innerHTML = annotator.highlight(highlightIndex)
}
var highlightIndexes = annotator.searchAll('Europe PMC')
if (highlightIndexes.length) {
document.getElementById('content').innerHTML = annotator.highlightAll(highlightIndexes)
}
document.getElementById('content').innerHTML = annotator.searchAndHighlight('a partner of PubMed Central')
document.getElementById('content').innerHTML = annotator.unhighlight(highlightIndex)
var highlightIndex = annotator.search('science', { prefix: 'international ', postfix: ' funders' })
if (highlightIndex !== -1) {
document.getElementById('content').innerHTML = annotator.highlight(highlightIndex)
}
Constructor
new TextAnnotator(options = {content})
Prop | Type | Description |
---|
content | string | The HTML string within which a piece of text can be annotated. |
Search APIs
search(str, options = {trim, caseSensitive, prefix, postfix})
searchAll(str, options = {trim, caseSensitive, prefix, postfix})
Prop | Type | Description |
---|
trim | boolean | Whether to trim the piece of text to be annotated. Default is true. |
caseSensitive | boolean | Whether to consider case in search. Default is false. |
prefix | string | A string BEFORE the piece of text to be annotated. Default is ''. |
postfix | string | A string AFTER the piece of text to be annotated. Default is ''. |
Annotation APIs
highlight(highlightIndex, options = {highlightTagName, highlightClass, highlightIdPattern})
highlightAll(highlightIndexes, options = {highlightTagName, highlightClass, highlightIdPattern})
unhighlight(highlightIndex, options = {highlightTagName, highlightClass, highlightIdPattern})
Prop | Type | Description |
---|
highlightTagName | string | The name of the annotation tag. Default is span so that the tag is <span ...>. |
highlightClass | string | The class name of the annotation tag. Default is highlight so that the tag is <span class="highlight" ...>. |
highlightIdPattern | string | The ID pattern of the annotation tag. Default is highlight- so that the tag is <span id="highlight-[highlightIndex]" ...>. |
searchAndHighlight API
searchAndHighlight(str, options), where options = { searchOptions, highlightOptions }, searchOptions and highlightOptions are described above in the Annotation options table.
Examples from Europe PMC
text-annotator has been widely used in Europe PMC, an open science platform that enables access to a worldwide collection of life science publications. Here is a list of examples:
- Article title highlighting: https://europepmc.org/search?query=cancer
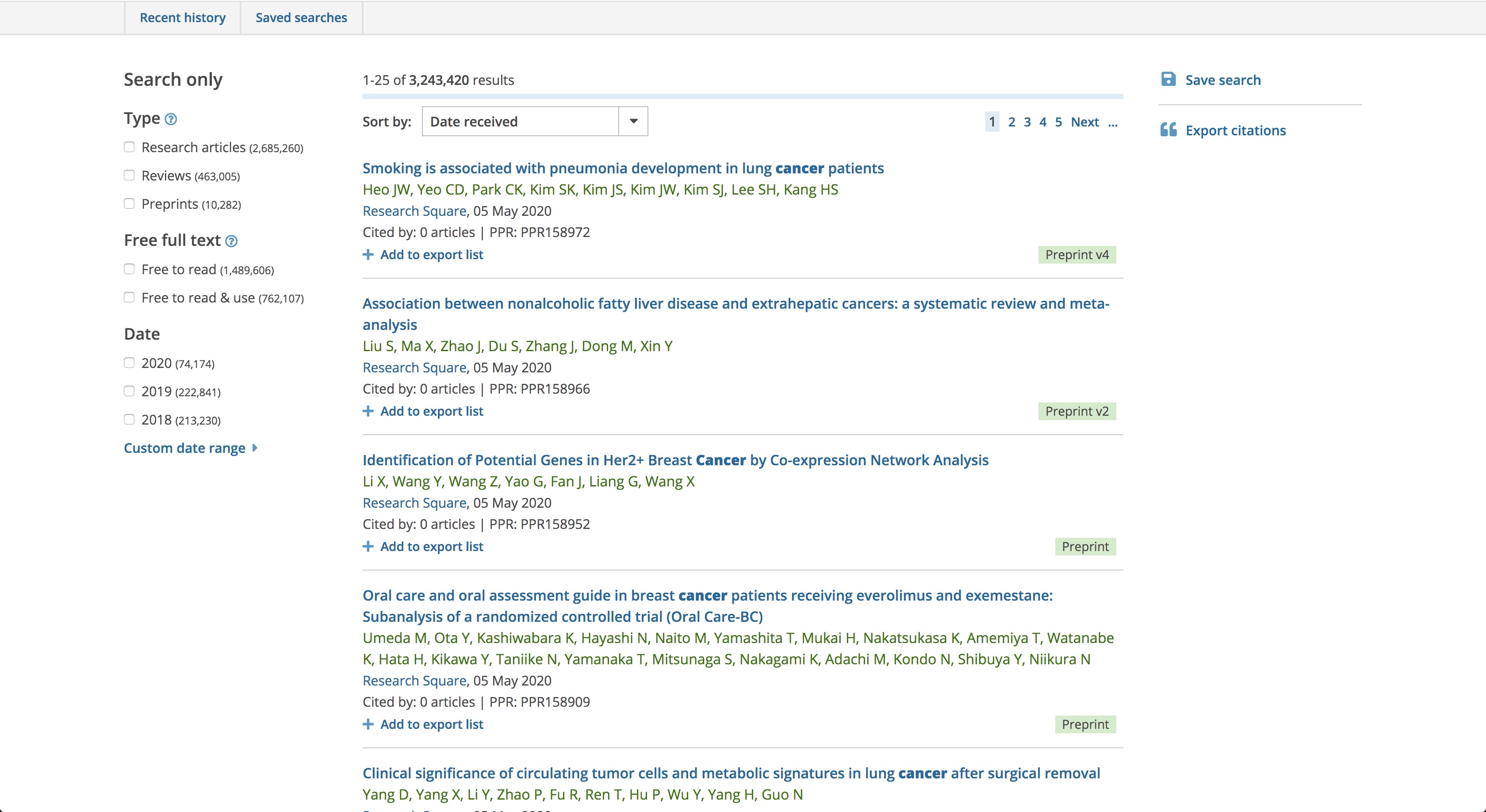
- Snippets: https://europepmc.org/article/PPR/PPR158972 (Visit from https://europepmc.org/search?query=cancer)
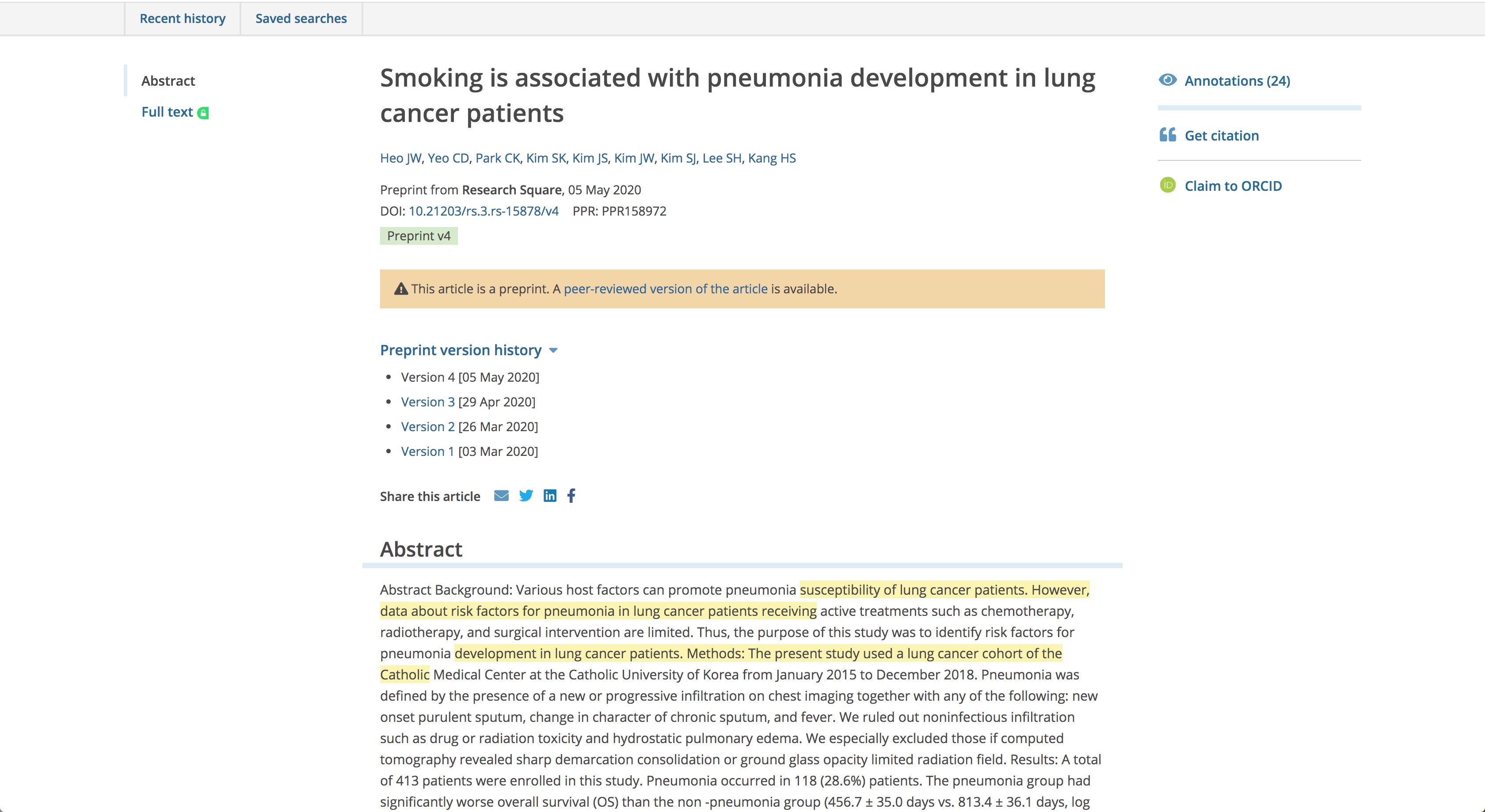
- SciLite: https://europepmc.org/article/PPR/PPR158972 (Click the Annotations link in the right panel)
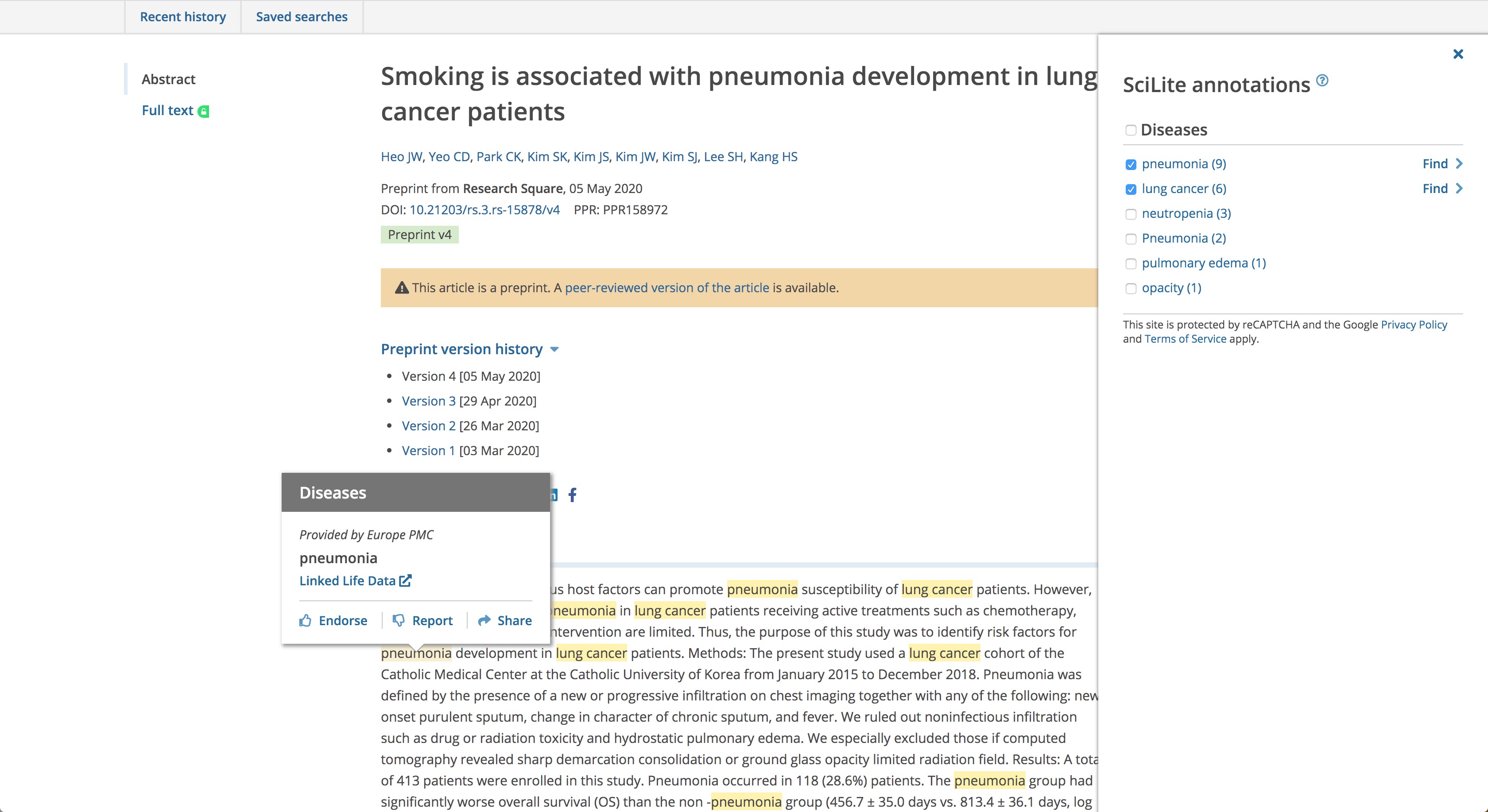
- Linkback: https://europepmc.org/article/PPR/PPR158972#europepmc-6e6312219dcad15c9a7dda8f71dce9af (In the popup shown in Example 3, click "Share" to get this linkback URL)
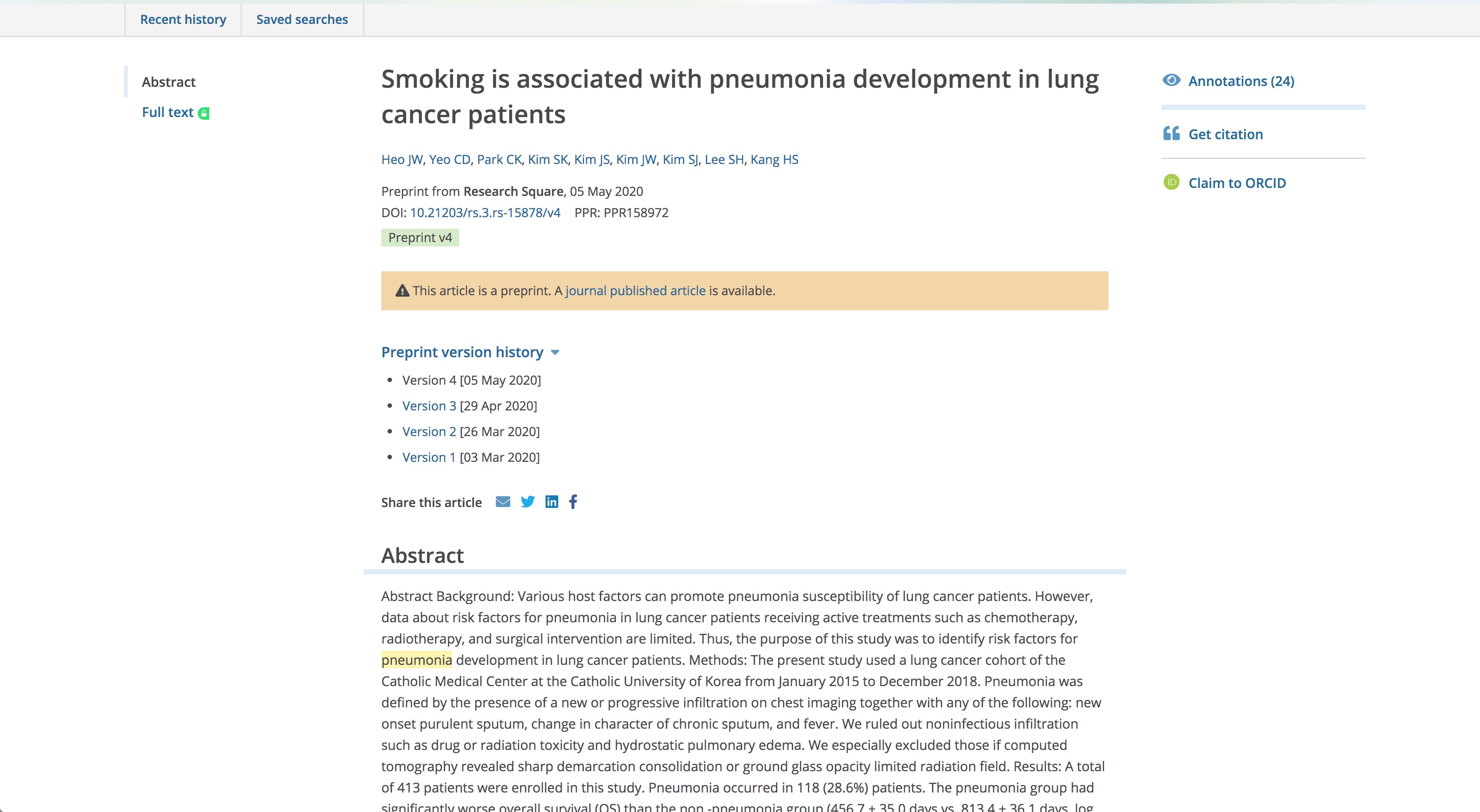
Contact
Zhan Huang