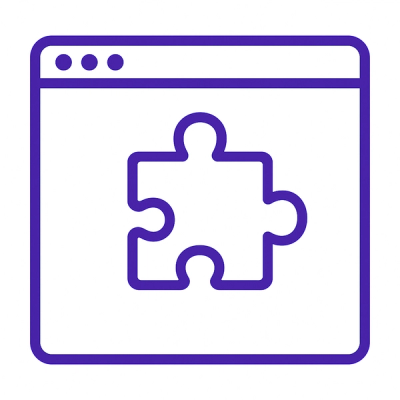
Research
Security News
The Growing Risk of Malicious Browser Extensions
Socket researchers uncover how browser extensions in trusted stores are used to hijack sessions, redirect traffic, and manipulate user behavior.
text-classification-rephrase
Advanced tools
A framework to classify and rephrase text/comments, turning negative comments into positive/encouraging ones and enhancing positive comments. Supports multiple languages including Indonesian.
Text Classification Rephrase adalah framework JavaScript yang menggunakan TensorFlow.js untuk mengklasifikasikan dan merephrase teks/komentar. Jika komentar bersifat negatif, framework ini akan mengubahnya menjadi positif/menyemangati. Jika komentar sudah positif, framework ini akan memperbaikinya menjadi lebih positif. Framework ini mendukung berbagai bahasa termasuk bahasa Indonesia.
Untuk menginstal package, jalankan perintah berikut:
npm install text-classification-rephrase
Berikut adalah contoh penggunaan dasar framework:
const TextClassificationRephrase = require('text-classification-rephrase');
(async () => {
const framework = new TextClassificationRephrase({
en: './models/en/text_classification_model.json',
es: './models/es/text_classification_model.json',
fr: './models/fr/text_classification_model.json',
id: './models/id/text_classification_model.json'
}, {
en: './embeddings/en/word2vec.bin',
es: './embeddings/es/word2vec.bin',
fr: './embeddings/fr/word2vec.bin',
id: './embeddings/id/word2vec.bin'
});
await framework.init();
const comment = "Kerja bagus!";
const processedComment = await framework.processComment(comment);
console.log(processedComment); // Output: "Itu hebat! Kerja bagus!"
})();
processComment
untuk mengklasifikasikan dan merephrase komentar.Anda dapat melatih model baru menggunakan TensorFlow dan menyimpannya dalam format yang dapat dibaca oleh TensorFlow.js. Berikut adalah contoh pelatihan model untuk bahasa Indonesia:
import tensorflow as tf
from tensorflow.keras.models import Sequential
from tensorflow.keras.layers import Dense
# Data dummy untuk ilustrasi
comments = ["Kerja bagus", "Ini buruk"]
labels = [1, 0] # 1 untuk positif, 0 untuk negatif
def preprocess(comment):
return [len(word) for word in comment.split()]
X = tf.keras.preprocessing.sequence.pad_sequences([preprocess(c) for c in comments], maxlen=10)
y = tf.keras.utils.to_categorical(labels)
model = Sequential([
Dense(10, activation='relu', input_shape=(10,)),
Dense(2, activation='softmax')
])
model.compile(optimizer='adam', loss='categorical_crossentropy', metrics=['accuracy'])
model.fit(X, y, epochs=10)
model.save('text_classification_model')
Ekspor model untuk TensorFlow.js:
tensorflowjs_converter --input_format keras text_classification_model models/id/text_classification_model
Dengan mengikuti langkah-langkah di atas, Anda akan memiliki model yang siap digunakan dalam framework ini.
Framework ini juga mendukung logging untuk memantau dan mendebug proses. Log disimpan di file app.log
di direktori proyek.
const { logger } = require('./src/logger');
logger.info('Informational message');
logger.error('Error message');
Untuk menambahkan bahasa baru, Anda perlu:
modelPaths
dan embeddingPaths
dalam inisialisasi framework dengan jalur ke model dan embeddings baru.const framework = new TextClassificationRephrase({
en: './models/en/text_classification_model.json',
es: './models/es/text_classification_model.json',
fr: './models/fr/text_classification_model.json',
id: './models/id/text_classification_model.json',
your_lang: './models/your_lang/text_classification_model.json'
}, {
en: './embeddings/en/word2vec.bin',
es: './embeddings/es/word2vec.bin',
fr: './embeddings/fr/word2vec.bin',
id: './embeddings/id/word2vec.bin',
your_lang: './embeddings/your_lang/word2vec.bin'
});
Kami menerima kontribusi dari semua pihak. Jika Anda menemukan bug atau memiliki fitur yang ingin ditambahkan, silakan buat pull request atau ajukan issue di repositori GitHub kami.
Framework ini dilisensikan di bawah lisensi MIT. Lihat file LICENSE
untuk informasi lebih lanjut.
text-classification-rephrase/
ā
āāā src/
ā āāā index.js
ā āāā classify.js
ā āāā rephrase.js
ā āāā language.js
ā āāā preprocess.js
ā āāā logger.js
ā
āāā models/
ā āāā en/
ā ā āāā text_classification_model.json
ā āāā es/
ā ā āāā text_classification_model.json
ā āāā fr/
ā ā āāā text_classification_model.json
ā āāā id/
ā āāā text_classification_model.json
ā
āāā embeddings/
ā āāā en/
ā ā āāā word2vec.bin
ā āāā es/
ā ā āāā word2vec.bin
ā āāā fr/
ā ā āāā word2vec.bin
ā āāā id/
ā āāā word2vec.bin
ā
āāā tests/
ā āāā index.test.js
ā
āāā .gitignore
āāā package.json
āāā README.md
Gunakan Jest untuk pengujian:
npm test
tests/index.test.js
const TextClassificationRephrase = require('../src/index');
test('Framework processes comments correctly', async () => {
const framework = new TextClassificationRephrase({
en: './models/en/text_classification_model.json',
es: './models/es/text_classification_model.json',
fr: './models/fr/text_classification_model.json',
id: './models/id/text_classification_model.json'
}, {
en: './embeddings/en/word2vec.bin',
es: './embeddings/es/word2vec.bin',
fr: './embeddings/fr/word2vec.bin',
id: './embeddings/id/word2vec.bin'
});
await framework.init();
const positiveCommentEn = "Great job!";
const negativeCommentEn = "This is terrible.";
const positiveCommentId = "Kerja bagus!";
const negativeCommentId = "Ini buruk.";
const processedPositiveCommentEn = await framework.processComment(positiveCommentEn);
const processedNegativeCommentEn = await framework.processComment(negativeCommentEn);
const processedPositiveCommentId = await framework.processComment(positiveCommentId);
const processedNegativeCommentId = await framework.processComment(negativeCommentId);
expect(processedPositiveCommentEn).toBe("That's great! Great job!");
expect(processedNegativeCommentEn).toBe("Keep it up! Don't let it get you down. This is terrible.");
expect(processedPositiveCommentId).toBe("Itu hebat! Kerja bagus!");
expect(processedNegativeCommentId).toBe("Tetap semangat! Jangan biarkan hal itu membuatmu down. Ini buruk.");
});
FAQs
A framework to classify and rephrase text/comments, turning negative comments into positive/encouraging ones and enhancing positive comments. Supports multiple languages including Indonesian.
The npm package text-classification-rephrase receives a total of 3 weekly downloads. As such, text-classification-rephrase popularity was classified as not popular.
We found that text-classification-rephrase demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago.Ā It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
Socket researchers uncover how browser extensions in trusted stores are used to hijack sessions, redirect traffic, and manipulate user behavior.
Research
Security News
An in-depth analysis of credential stealers, crypto drainers, cryptojackers, and clipboard hijackers abusing open source package registries to compromise Web3 development environments.
Security News
pnpm 10.12.1 introduces a global virtual store for faster installs and new options for managing dependencies with version catalogs.