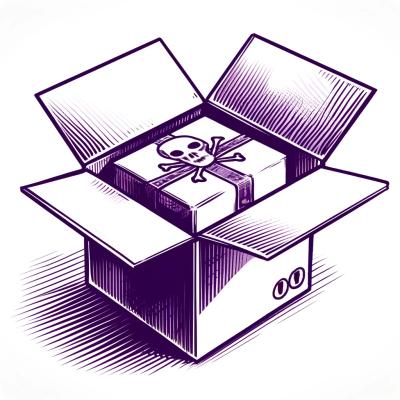
Security News
Research
Data Theft Repackaged: A Case Study in Malicious Wrapper Packages on npm
The Socket Research Team breaks down a malicious wrapper package that uses obfuscation to harvest credentials and exfiltrate sensitive data.
[](https://github.com/tinylibs/tinybench/actions/workflows/test.yml) [](https://www.npmjs.com
The tinybench npm package is a lightweight benchmarking tool for JavaScript. It allows developers to measure the performance of their code by running benchmarks and comparing the execution times of different code snippets.
Basic Benchmarking
This feature allows you to create a basic benchmark test. You can add multiple tests to the benchmark and run them to measure their performance.
const { Bench } = require('tinybench');
const bench = new Bench();
bench.add('Example Test', () => {
// Code to benchmark
for (let i = 0; i < 1000; i++) {}
});
bench.run().then(() => {
console.log(bench.table());
});
Asynchronous Benchmarking
This feature allows you to benchmark asynchronous code. You can add tests that return promises and the benchmark will wait for them to resolve before measuring their performance.
const { Bench } = require('tinybench');
const bench = new Bench();
bench.add('Async Test', async () => {
// Asynchronous code to benchmark
await new Promise(resolve => setTimeout(resolve, 1000));
});
bench.run().then(() => {
console.log(bench.table());
});
Customizing Benchmark Options
This feature allows you to customize the benchmark options such as the total time to run the benchmark and the number of iterations. This can help in fine-tuning the benchmarking process.
const { Bench } = require('tinybench');
const bench = new Bench({ time: 2000, iterations: 10 });
bench.add('Custom Options Test', () => {
// Code to benchmark
for (let i = 0; i < 1000; i++) {}
});
bench.run().then(() => {
console.log(bench.table());
});
The 'benchmark' package is a popular benchmarking library for JavaScript. It provides a robust API for measuring the performance of code snippets. Compared to tinybench, 'benchmark' offers more advanced features and a more comprehensive API, but it is also larger in size.
The 'perf_hooks' module is a built-in Node.js module that provides an API for measuring performance. It is more low-level compared to tinybench and requires more manual setup, but it is very powerful and flexible for detailed performance analysis.
The 'benny' package is another benchmarking tool for JavaScript. It focuses on simplicity and ease of use, similar to tinybench. However, 'benny' provides a more modern API and better integration with modern JavaScript features like async/await.
Benchmark your code easily with Tinybench, a simple, tiny and light-weight 10KB
(2KB
minified and gzipped) benchmarking library!
You can run your benchmarks in multiple JavaScript runtimes, Tinybench is completely based on the Web APIs with proper timing using
process.hrtime
or performance.now
.
Event
and EventTarget
compatible eventsIn case you need more tiny libraries like tinypool or tinyspy, please consider submitting an RFC
$ npm install -D tinybench
You can start benchmarking by instantiating the Bench
class and adding benchmark tasks to it.
import { Bench } from 'tinybench'
const bench = new Bench({ name: 'simple benchmark', time: 100 })
bench
.add('faster task', () => {
console.log('I am faster')
})
.add('slower task', async () => {
await new Promise(resolve => setTimeout(resolve, 1)) // we wait 1ms :)
console.log('I am slower')
})
await bench.run()
console.log(bench.name)
console.table(bench.table())
// Output:
// simple benchmark
// ┌─────────┬───────────────┬────────────────────────────┬───────────────────────────┬──────────────────────┬─────────────────────┬─────────┐
// │ (index) │ Task name │ Throughput average (ops/s) │ Throughput median (ops/s) │ Latency average (ns) │ Latency median (ns) │ Samples │
// ├─────────┼───────────────┼────────────────────────────┼───────────────────────────┼──────────────────────┼─────────────────────┼─────────┤
// │ 0 │ 'faster task' │ '102906 ± 0.89%' │ '82217 ± 14' │ '11909.14 ± 3.95%' │ '12163.00 ± 2.00' │ 8398 │
// │ 1 │ 'slower task' │ '988 ± 26.26%' │ '710' │ '1379560.47 ± 6.72%' │ '1408552.00' │ 73 │
// └─────────┴───────────────┴────────────────────────────┴───────────────────────────┴──────────────────────┴─────────────────────┴─────────┘
The add
method accepts a task name and a task function, so it can benchmark
it! This method returns a reference to the Bench instance, so it's possible to
use it to create an another task for that instance.
Note that the task name should always be unique in an instance, because Tinybench stores the tasks based
on their names in a Map
.
Also note that tinybench
does not log any result by default. You can extract the relevant stats
from bench.tasks
or any other API after running the benchmark, and process them however you want.
More usage examples can be found in the examples directory.
Bench
Task
TaskResult
Events
Both the Task
and Bench
classes extend the EventTarget
object. So you can attach listeners to different types of events in each class instance using the universal addEventListener
and removeEventListener
methods.
BenchEvents
// runs on each benchmark task's cycle
bench.addEventListener('cycle', (evt) => {
const task = evt.task!;
});
TaskEvents
// runs only on this benchmark task's cycle
task.addEventListener('cycle', (evt) => {
const task = evt.task!;
});
BenchEvent
process.hrtime
if you want more accurate results for nodejs with process.hrtime
, then import
the hrtimeNow
function from the library and pass it to the Bench
options.
import { hrtimeNow } from 'tinybench'
It may make your benchmarks slower.
mode
is set to null
(default), concurrency is disabled.mode
is set to 'task', each task's iterations (calls of a task function) run concurrently.mode
is set to 'bench', different tasks within the bench run concurrently. Concurrent cycles.bench.threshold = 10 // The maximum number of concurrent tasks to run. Defaults to Number.POSITIVE_INFINITY.
bench.concurrency = 'task' // The concurrency mode to determine how tasks are run.
await bench.run()
Mohammad Bagher |
---|
Uzlopak | poyoho |
---|
Feel free to create issues/discussions and then PRs for the project!
Your sponsorship can make a huge difference in continuing our work in open source!
FAQs
[](https://github.com/tinylibs/tinybench/actions/workflows/test.yml) [](https://www.npmjs.com
The npm package tinybench receives a total of 5,569,265 weekly downloads. As such, tinybench popularity was classified as popular.
We found that tinybench demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 3 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Research
The Socket Research Team breaks down a malicious wrapper package that uses obfuscation to harvest credentials and exfiltrate sensitive data.
Research
Security News
Attackers used a malicious npm package typosquatting a popular ESLint plugin to steal sensitive data, execute commands, and exploit developer systems.
Security News
The Ultralytics' PyPI Package was compromised four times in one weekend through GitHub Actions cache poisoning and failure to rotate previously compromised API tokens.