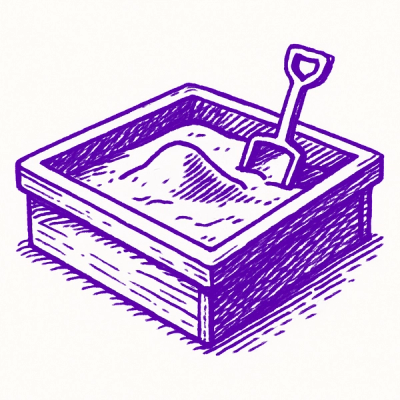
Research
/Security News
Critical Vulnerability in NestJS Devtools: Localhost RCE via Sandbox Escape
A flawed sandbox in @nestjs/devtools-integration lets attackers run code on your machine via CSRF, leading to full Remote Code Execution (RCE).
The tinyqueue npm package is a minimal priority queue implementation in JavaScript. It is designed to be very lightweight and fast, and it is often used for tasks that require a priority queue, such as pathfinding algorithms, scheduling, and event handling.
Creating a priority queue
This code sample demonstrates how to import the TinyQueue class and create a new priority queue instance.
const TinyQueue = require('tinyqueue');
const queue = new TinyQueue();
Adding items to the queue
This code sample shows how to add items to the queue with a specified priority. The lower the priority number, the sooner the item will be dequeued.
queue.push({ value: 'item1', priority: 1 });
queue.push({ value: 'item2', priority: 2 });
Removing the item with the highest priority
This code sample demonstrates how to remove and return the item with the highest priority (the item with the lowest priority number) from the queue.
const highestPriorityItem = queue.pop();
Peeking at the item with the highest priority without removing it
This code sample shows how to look at the item with the highest priority without removing it from the queue.
const highestPriorityItem = queue.peek();
Checking the size of the queue
This code sample demonstrates how to check the number of items in the queue.
const size = queue.length;
PriorityQueue.js is another npm package that provides a priority queue implementation. It offers similar functionality to tinyqueue but has a different API and may have different performance characteristics.
js-priority-queue is a priority queue library for JavaScript that supports customizable ordering and initial queue contents. It is more feature-rich than tinyqueue, allowing for different strategies for storing the data and more complex comparison functions.
The heap npm package is a binary heap implementation that can be used as a priority queue. It is more complex than tinyqueue and includes additional methods for heap operations, which might be useful for certain applications that require more control over the data structure.
The smallest and simplest binary heap priority queue in JavaScript.
// create an empty priority queue
var queue = new TinyQueue();
// add some items
queue.push(7);
queue.push(5);
queue.push(10);
// remove the top item
var top = queue.pop(); // returns 5
// return the top item (without removal)
top = queue.peek(); // returns 7
// get queue length
queue.length; // returns 2
// create a priority queue from an existing array (modifies the array)
queue = new TinyQueue([7, 5, 10]);
// pass a custom item comparator as a second argument
queue = new TinyQueue([{value: 5}, {value: 7}], function (a, b) {
return a.value - b.value;
});
// turn a queue into a sorted array
var array = [];
while (queue.length) array.push(queue.pop());
For a faster number-based queue, see flatqueue.
Install using NPM (npm install tinyqueue
) or Yarn (yarn add tinyqueue
), then:
// import as an ES module
import TinyQueue from 'tinyqueue';
// or require in Node / Browserify
const TinyQueue = require('tinyqueue');
Or use a browser build directly:
<script src="https://unpkg.com/tinyqueue@2.0.0/tinyqueue.min.js"></script>
Inspired by js-priority-queue by Adam Hooper.
FAQs
The smallest and simplest JavaScript priority queue
The npm package tinyqueue receives a total of 3,483,451 weekly downloads. As such, tinyqueue popularity was classified as popular.
We found that tinyqueue demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
/Security News
A flawed sandbox in @nestjs/devtools-integration lets attackers run code on your machine via CSRF, leading to full Remote Code Execution (RCE).
Product
Customize license detection with Socket’s new license overlays: gain control, reduce noise, and handle edge cases with precision.
Product
Socket now supports Rust and Cargo, offering package search for all users and experimental SBOM generation for enterprise projects.