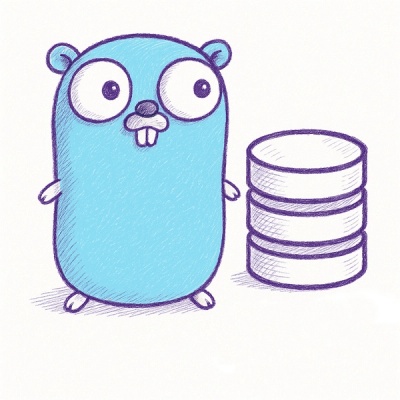
Security News
Official Go SDK for MCP in Development, Stable Release Expected in August
The official Go SDK for the Model Context Protocol is in development, with a stable, production-ready release expected by August 2025.
Turns every JavaScript object or primitive into valid source code that can be evaled again.
You can use it to serialize classes, modules or other programming objects and reuse them in an other environment such as a browser. JSON.stringify doesnt work with programming objects (that contain functions, dates, etc.) because they're no legal JSONs.
npm install toSrc
var toSrc = require("toSrc");
// Primitives
///////////////////////////////////////
toSrc(1); // = '1'
toSrc(true); // = 'true'
toSrc(undefined); // = 'undefined'
tOSrc(null); // = null
toSrc("1"); // = '"1"'
toSrc('1'); // = '"1"' toSrc always uses double-quotes
// Constants
///////////////////////////////////////
toSrc(Math.PI); // = 'Math.PI'
toSrc(NaN); // = 'NaN'
// RegExp
///////////////////////////////////////
toSrc(/myRegEx/gi); // = '/myRegEx/gi'
toSrc(new RegExp("myRegEx")); // = '/myRegEx/'
// Date
///////////////////////////////////////
toSrc(new Date()); // = 'new Date(<the time of creation in ms>)'
// Functions
///////////////////////////////////////
function testFunc() {
var test = "hello";
}
toSrc(testFunc); // = 'function testFunc() {\n var test = "hello";\n}'
toSrc(String); // = 'String', native functions don't expose the source code
// Arrays
///////////////////////////////////////
toSrc([1, 2, "3"]); // = '[1, 2, "3"]'
toSrc([1, 2, ["a", "b", "c"]]); // = '[1, 2, undefined]' because the depth
// is 1 by default
toSrc([1, 2, ["a", "b", "c"]], 2); // = '[1, 2, ["a", "b", "c"]]'
// Objects
///////////////////////////////////////
toSrc({
regEx: /regex/gi,
anotherObj: {
test: "test"
}
});
// = '{"regEx": /regex/gi, "anotherObj": undefined}'
// anotherObj is undefined because the depth is 1 by default.
toSrc({
"regEx": /regex/gi,
"anotherObj": {
"test": "test"
}
}, 2);
// = '{"regEx": /regex/gi, "anotherObj": {"test": "test"}}'
For more examples check out test/test.js
###toSrc(obj: *, depth: Number): String
obj:
The object to stringify. Can also be a primitive like 1
or true
.
{Number=1} depth:
The depth to go. All nested structures like objects or arrays deeper than this will be undefined. Defaults to 1, meaning that every object or array within obj
will be undefined by default.
var toSrc = require("toSrc");
toSrc(obj, depth);
Just call toSrc(obj, depth);
toSrc(Math.PI); // = 'Math.PI' instead of 3.14...
toSrc(new Date()) // = 'new Date(<time of creation in ms>)'
new RegExp()
will not be dynamic anymore. toSrc(new RegExp(someString))
will return '/<value of someString>/'
instead of 'new RegExp(someString)'
Feel free to modify the code to meet your needs.
MIT
FAQs
Turns every JavaScript object or primitive into valid source code.
The npm package toSrc receives a total of 953 weekly downloads. As such, toSrc popularity was classified as not popular.
We found that toSrc demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
The official Go SDK for the Model Context Protocol is in development, with a stable, production-ready release expected by August 2025.
Security News
New research reveals that LLMs often fake understanding, passing benchmarks but failing to apply concepts or stay internally consistent.
Security News
Django has updated its security policies to reject AI-generated vulnerability reports that include fabricated or unverifiable content.