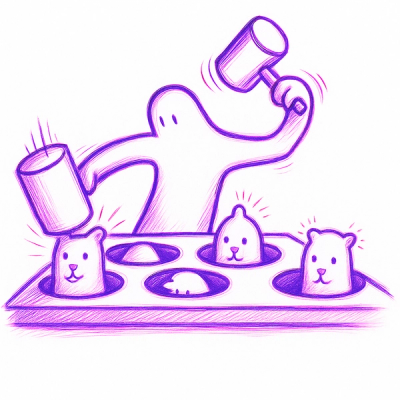
Research
/Security News
Contagious Interview Campaign Escalates With 67 Malicious npm Packages and New Malware Loader
North Korean threat actors deploy 67 malicious npm packages using the newly discovered XORIndex malware loader.
topological-sort
Advanced tools
This package is distributed as Javascript, but you can also use it in your TypeScript project.
const { TopologicalSort } = require('topological-sort');
// you can pass nodes as a map into constructor:
const nodes = new Map();
nodes.set('variables', variablesObj);
nodes.set('mixins', mixinsObj);
const sortOp = new TopologicalSort(nodes);
// ...or add them to existing object instance with addNode() or addNodes():
sortOp.addNode('block', blocksObj);
sortOp.addNodes(new Map([
['block_mod_val1', blockModObj1],
['block_mod_val2', blockModObj2]
]));
// then you should add adges between nodes
sortOp.addEdge('variables', 'mixins'); // from, to
sortOp.addEdge('mixins', 'block');
sortOp.addEdge('variables', 'block');
sortOp.addEdge('block', 'block_mod_val2');
sortOp.addEdge('block', 'block_mod_val1');
// sorting is simple: it returns a new map wih sorted elements
// if circular dependency is found, sort() operation throws an AssertionError
const sorted = sortOp.sort();
const sortedKeys = [...sorted.keys()]; // ['variables', 'mixins', 'block', 'block_mod_val1', 'block_mod_val2']
// values of the `sorted` map are objects with this shape: `{ children, node }`
// where node is the node object that you provided
// and children is a map which values have the same shape
const { node: variablesObj, children: variablesChildren } = sorted.get('variables');
const { node: blocksObj1 } = variablesChildren.get('block');
const { node: blocksObj2 } = sorted.get('block');
assert(blocksObj1 === blocksObj2); // true
import TopologicalSort from 'topological-sort';
// TopologicalSort class instances have a map inside.
// This map stores the references between your nodes (edges)
// "NodesKeyType" is the type for your tree node identifiers
// "NodesValueType" is the type for your tree nodes
const nodes = new Map<NodesKeyType, NodesValueType>();
nodes.set('variables', variablesObj);
nodes.set('mixins', mixinsObj);
const sortOp = new TopologicalSort<NodesKeyType, NodesValueType>(nodes);
// `sortedKeys` is a topologically sorted list of node keys
sortOp.addEdge('variables', 'mixins');
const sorted = sortOp.sort();
const sortedKeys = [...sorted.keys()]; // ['variables', 'mixins']
// `sorted` contains all nodes and their children
const { node: variablesObj, children: variablesChildren } = sorted.get('variables');
const { node: blocksObj1 } = variablesChildren.get('block');
const { node: blocksObj2 } = sorted.get('block');
assert(blocksObj1 === blocksObj2); // true
0.3.0
sort()
return map values are now {node, children}
where children
is also a map. See README for examples.FAQs
Topological sort
The npm package topological-sort receives a total of 32,528 weekly downloads. As such, topological-sort popularity was classified as popular.
We found that topological-sort demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
/Security News
North Korean threat actors deploy 67 malicious npm packages using the newly discovered XORIndex malware loader.
Security News
Meet Socket at Black Hat & DEF CON 2025 for 1:1s, insider security talks at Allegiant Stadium, and a private dinner with top minds in software supply chain security.
Security News
CAI is a new open source AI framework that automates penetration testing tasks like scanning and exploitation up to 3,600× faster than humans.