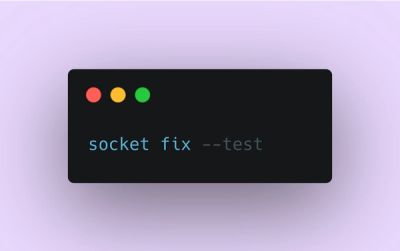
Product
Introducing Socket Fix for Safe, Automated Dependency Upgrades
Automatically fix and test dependency updates with socket fix—a new CLI tool that turns CVE alerts into safe, automated upgrades.
Connects to Doublepoint Touch SDK compatible Bluetooth devices – like this WearOS app.
Works with Chrome-based browsers. (more info)
<script src="https://cdn.jsdelivr.net/npm/touch-sdk@0.6.0/dist/main.js"></script>
const Watch = TouchSDK.Watch
npm install touch-sdk
import { Watch } from 'touch-sdk'
<!DOCTYPE html>
<html>
<head>
<script src="https://cdn.jsdelivr.net/npm/touch-sdk@0.5.2/dist/main.js"></script>
</head>
<body>
<script>
const watch = new TouchSDK.Watch()
const connectButton = watch.createConnectButton()
document.body.appendChild(connectButton)
watch.addEventListener('tap', () => {
console.log('tap')
})
</script>
</body>
</html>
watch.createConnectButton
Returns a button element with the following properties:
onclick
calls watch.requestConnection
touch-sdk-connect-button
This function does not add the button to the DOM.
watch.requestConnection
Can only be called as a result of a user action, for example a button click. Otherwise doesn't do anything.
watch.requestConnection().then(() => {
console.log('connected')
}, error => {
alert(error.message)
})
watch.addEventListener('tap', (event) => {
console.log('tap')
})
```javascript
watch.addEventListener('probability', (event) => {
console.log("gesture probability:", event.detail)
})
watch.addEventListener('armdirectionchanged', event => {
const { dx, dy } = event.detail
console.log(dx, dy)
})
All applicable units are SI-based.
watch.addEventListener('accelerationchanged', (event) => {
const { x, y, z } = event.detail
console.log(x, y, z)
})
watch.addEventListener('angularvelocitychanged', (event) => {
const { x, y, z } = event.detail
console.log(x, y, z)
})
watch.addEventListener('gravityvectorchanged', (event) => {
const { x, y, z } = event.detail
console.log(x, y, z)
})
watch.addEventListener('orientationchanged', (event) => {
const { x, y, z, w } = event.detail
console.log(x, y, z, w)
})
watch.addEventListener('touchstart', (event) => {
const { x, y } = event.detail
console.log(x, y)
})
watch.addEventListener('touchmove', (event) => {
const { x, y } = event.detail
console.log(x, y)
})
watch.addEventListener('touchend', (event) => {
const { x, y } = event.detail
console.log(x, y)
})
For example the user swiped from the edge of the screen, and triggered an operating system gesture. Usually touchcancel
should be handled the same way as touchend
.
watch.addEventListener('touchcancel', (event) => {
const { x, y } = event.detail
console.log(x, y)
})
watch.addEventListener('rotary', (event) => {
const { step } = event.detail
console.log(step)
})
In Wear OS this is the back button. Only clicks are registered, no button down and button up events.
watch.addEventListener('button', (event) => {
console.log('button')
})
After the connected
event, several watch properties become available.
watch.addEventListener('connected', (event) => {
watch.hand // 'left' or 'right'
watch.hapticsAvailable // true or false
watch.touchScreenResolution // (width, height). (0, 0) if no touch screen
watch.batteryPercentage // 0-100
})
Intensity is between 0 and 1. Length is milliseconds, between 0 and 5000.
watch.triggerHaptics(intensity, length)
npm install
npm run build
npm publish
FAQs
Doublepoint Touch SDK
The npm package touch-sdk receives a total of 5 weekly downloads. As such, touch-sdk popularity was classified as not popular.
We found that touch-sdk demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 2 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Product
Automatically fix and test dependency updates with socket fix—a new CLI tool that turns CVE alerts into safe, automated upgrades.
Security News
CISA denies CVE funding issues amid backlash over a new CVE foundation formed by board members, raising concerns about transparency and program governance.
Product
We’re excited to announce a powerful new capability in Socket: historical data and enhanced analytics.